mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-11-10 18:15:19 +00:00
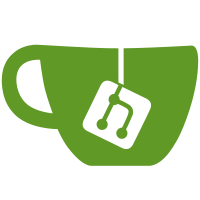
* rough auction type refactor * replace endTime type * split keeper file up * update store methods * move store methods to keeper.go * move nextAuctionID from params to genState * simplify auction type to not use pointers * add basic auction tests * update endblocker test * add payout to depositors feature * add more tests * move index updates to Get/Set for more safety * remove slightly unecessary ID type * remove unused message types * feat: add spec, update redundant type names * stop sending zero coins * use only one coins field in MsgPlaceBid * remove uncessary Auction interface methods * give auction types more accurate names * remove vuepress comments from spec * minor spec updates * update doc comments * add params validation * code cleanup, address review comments * resolve minor TODOs * sync spec with code Co-authored-by: Kevin Davis <karzak@users.noreply.github.com>
33 lines
921 B
Markdown
33 lines
921 B
Markdown
# Messages
|
|
|
|
## Bidding
|
|
|
|
Users can bid on auctions using the `MsgPlaceBid` message type. All auction types can be bid on using the same message type.
|
|
|
|
```go
|
|
// MsgPlaceBid is the message type used to place a bid on any type of auction.
|
|
type MsgPlaceBid struct {
|
|
AuctionID uint64
|
|
Bidder sdk.AccAddress
|
|
Amount sdk.Coin
|
|
}
|
|
```
|
|
|
|
**State Modifications:**
|
|
|
|
* Update bidder if different than previous bidder
|
|
* For Surplus auctions:
|
|
* Update Bid to msg.Amount
|
|
* Return bid coins to previous bidder
|
|
* Burn coins equal to the increment in the bid (CurrentBid - PreviousBid)
|
|
* For Debt auctions:
|
|
* Update Lot amount to msg.Amount
|
|
* Return bid coins to previous bidder
|
|
* For Collateral auctions:
|
|
* Return bid coins to previous bidder
|
|
* If in forward phase:
|
|
* Update Bid amount to msg.Amount
|
|
* If in reverse phase:
|
|
* Update Lot amount to msg.Amount
|
|
* Extend auction by `BidDuration`, up to `MaxEndTime`
|