mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 22:57:32 +00:00
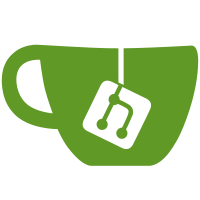
* bump SDK version to v0.38.2 * fix module.go and remove codespaces * fix coins Add() * fixes to handlers * migrate errors * more fixes * fixes fixes fixes * build * check for paramstore keytable * empty param validation function (TODO) * param validations * fix some tests * fix all tests * simulation fixes (WIP) * auction and bep3 sim refactor * fixes * bep3 sims fixes * auction and pricefeed fix * cdp sims fixes * fix tests * Update x/auction/keeper/auctions.go Co-Authored-By: Denali Marsh <denali@kava.io> * Update x/bep3/types/params.go Co-Authored-By: Denali Marsh <denali@kava.io> * Apply suggestions from code review Co-Authored-By: Denali Marsh <denali@kava.io> * Update x/bep3/keeper/swap.go Co-Authored-By: Denali Marsh <denali@kava.io> * address comments from review * address comments from review * fix: run sims * fix: implement marshal/unmarshal JSON for validator vesting account * fix: don't call set on sealed config * remove swap interface * add concepts spec * add state spec * add messages spec * update event names * implement swap expired event * add events spec * add params spec * add begin block spec * add module readme * update alias * revisions * aggregate expired swap ids for event emisison * markdown-link-check-disable for circleci * exclude api-endpoint links in Makefile Co-authored-by: Federico Kunze <federico.kunze94@gmail.com> Co-authored-by: Federico Kunze <31522760+fedekunze@users.noreply.github.com> Co-authored-by: Kevin Davis <kjydavis3@gmail.com>
3.4 KiB
3.4 KiB
State
Parameters and genesis state
Paramaters
define the rules according to which swaps are executed. Parameter updates can be made via on-chain parameter update proposals.
// Params governance parameters for bep3 module
type Params struct {
BnbDeputyAddress sdk.AccAddress `json:"bnb_deputy_address" yaml:"bnb_deputy_address"` // deputy's address on Kava
MinBlockLock int64 `json:"min_block_lock" yaml:"min_block_lock"` // minimum swap expire height
MaxBlockLock int64 `json:"max_block_lock" yaml:"max_block_lock"` // maximum swap expire height
SupportedAssets AssetParams `json:"supported_assets" yaml:"supported_assets"` // array of supported asset
}
// AssetParam governance parameters for each asset within a supported chain
type AssetParam struct {
Denom string `json:"denom" yaml:"denom"` // name of the asset
CoinID int `json:"coin_id" yaml:"coin_id"` // internationally recognized coin ID
Limit sdk.Int `json:"limit" yaml:"limit"` // asset supply limit
Active bool `json:"active" yaml:"active"` // denotes if asset is active or paused
}
GenesisState
defines the state that must be persisted when the blockchain stops/restarts in order for normal function of the bep3 module to resume.
// GenesisState - all bep3 state that must be provided at genesis
type GenesisState struct {
Params Params `json:"params" yaml:"params"`
AtomicSwaps AtomicSwaps `json:"atomic_swaps" yaml:"atomic_swaps"`
AssetSupplies AssetSupplies `json:"assets_supplies" yaml:"assets_supplies"`
}
Types
// AtomicSwap contains the information for an atomic swap
type AtomicSwap struct {
Amount sdk.Coins `json:"amount" yaml:"amount"`
RandomNumberHash tmbytes.HexBytes `json:"random_number_hash" yaml:"random_number_hash"`
ExpireHeight int64 `json:"expire_height" yaml:"expire_height"`
Timestamp int64 `json:"timestamp" yaml:"timestamp"`
Sender sdk.AccAddress `json:"sender" yaml:"sender"`
Recipient sdk.AccAddress `json:"recipient" yaml:"recipient"`
SenderOtherChain string `json:"sender_other_chain" yaml:"sender_other_chain"`
RecipientOtherChain string `json:"recipient_other_chain" yaml:"recipient_other_chain"`
ClosedBlock int64 `json:"closed_block" yaml:"closed_block"`
Status SwapStatus `json:"status" yaml:"status"`
CrossChain bool `json:"cross_chain" yaml:"cross_chain"`
Direction SwapDirection `json:"direction" yaml:"direction"`
}
// SwapStatus is the status of an AtomicSwap
type SwapStatus byte
const (
NULL SwapStatus = 0x00
Open SwapStatus = 0x01
Completed SwapStatus = 0x02
Expired SwapStatus = 0x03
)
// SwapDirection is the direction of an AtomicSwap
type SwapDirection byte
const (
INVALID SwapDirection = 0x00
Incoming SwapDirection = 0x01
Outgoing SwapDirection = 0x02
)
// AssetSupply contains information about an asset's supply
type AssetSupply struct {
Denom string `json:"denom" yaml:"denom"`
IncomingSupply sdk.Coin `json:"incoming_supply" yaml:"incoming_supply"`
OutgoingSupply sdk.Coin `json:"outgoing_supply" yaml:"outgoing_supply"`
CurrentSupply sdk.Coin `json:"current_supply" yaml:"current_supply"`
Limit sdk.Coin `json:"limit" yaml:"limit"`
}