mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 14:47:31 +00:00
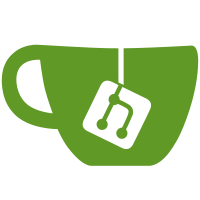
* Use cosmossdk.io/errors for deprecated error methods * Update error registration with cosmossdk.io/errors * Use cosmossdk.io/math for deprecated sdk.Int alias * Fix modified proto file * Update sdk.Int usage in swap hooks * Update e2e test deprecated method usage
128 lines
4.7 KiB
Markdown
128 lines
4.7 KiB
Markdown
<!--
|
|
order: 6
|
|
-->
|
|
|
|
# Hooks
|
|
|
|
This module implements the `Hooks` interface for the following modules:
|
|
|
|
- cdp
|
|
- hard
|
|
- swap
|
|
- staking (defined in cosmos-sdk)
|
|
|
|
CDP module hooks manage the creation and synchronization of USDX minting incentives.
|
|
|
|
```go
|
|
// ------------------- Cdp Module Hooks -------------------
|
|
|
|
// AfterCDPCreated function that runs after a cdp is created
|
|
func (h Hooks) AfterCDPCreated(ctx sdk.Context, cdp cdptypes.CDP) {
|
|
h.k.InitializeUSDXMintingClaim(ctx, cdp)
|
|
}
|
|
|
|
// BeforeCDPModified function that runs before a cdp is modified
|
|
// note that this is called immediately after interest is synchronized, and so could potentially
|
|
// be called AfterCDPInterestUpdated or something like that, if we we're to expand the scope of cdp hooks
|
|
func (h Hooks) BeforeCDPModified(ctx sdk.Context, cdp cdptypes.CDP) {
|
|
h.k.SynchronizeUSDXMintingReward(ctx, cdp)
|
|
}
|
|
```
|
|
|
|
Hard module hooks manage the creation and synchronization of hard supply and borrow rewards.
|
|
|
|
```go
|
|
// ------------------- Hard Module Hooks -------------------
|
|
|
|
// AfterDepositCreated function that runs after a deposit is created
|
|
func (h Hooks) AfterDepositCreated(ctx sdk.Context, deposit hardtypes.Deposit) {
|
|
h.k.InitializeHardSupplyReward(ctx, deposit)
|
|
}
|
|
|
|
// BeforeDepositModified function that runs before a deposit is modified
|
|
func (h Hooks) BeforeDepositModified(ctx sdk.Context, deposit hardtypes.Deposit) {
|
|
h.k.SynchronizeHardSupplyReward(ctx, deposit)
|
|
}
|
|
|
|
// AfterDepositModified function that runs after a deposit is modified
|
|
func (h Hooks) AfterDepositModified(ctx sdk.Context, deposit hardtypes.Deposit) {
|
|
h.k.UpdateHardSupplyIndexDenoms(ctx, deposit)
|
|
}
|
|
|
|
// AfterBorrowCreated function that runs after a borrow is created
|
|
func (h Hooks) AfterBorrowCreated(ctx sdk.Context, borrow hardtypes.Borrow) {
|
|
h.k.InitializeHardBorrowReward(ctx, borrow)
|
|
}
|
|
|
|
// BeforeBorrowModified function that runs before a borrow is modified
|
|
func (h Hooks) BeforeBorrowModified(ctx sdk.Context, borrow hardtypes.Borrow) {
|
|
h.k.SynchronizeHardBorrowReward(ctx, borrow)
|
|
}
|
|
|
|
// AfterBorrowModified function that runs after a borrow is modified
|
|
func (h Hooks) AfterBorrowModified(ctx sdk.Context, borrow hardtypes.Borrow) {
|
|
h.k.UpdateHardBorrowIndexDenoms(ctx, borrow)
|
|
}
|
|
```
|
|
|
|
Staking module hooks manage the creation and synchronization of hard delegator rewards.
|
|
|
|
```go
|
|
// ------------------- Staking Module Hooks -------------------
|
|
|
|
// BeforeDelegationCreated runs before a delegation is created
|
|
func (h Hooks) BeforeDelegationCreated(ctx sdk.Context, delAddr sdk.AccAddress, valAddr sdk.ValAddress) {
|
|
h.k.InitializeHardDelegatorReward(ctx, delAddr)
|
|
}
|
|
|
|
// BeforeDelegationSharesModified runs before an existing delegation is modified
|
|
func (h Hooks) BeforeDelegationSharesModified(ctx sdk.Context, delAddr sdk.AccAddress, valAddr sdk.ValAddress) {
|
|
h.k.SynchronizeHardDelegatorRewards(ctx, delAddr)
|
|
}
|
|
|
|
// NOTE: following hooks are just implemented to ensure StakingHooks interface compliance
|
|
|
|
// BeforeValidatorSlashed is called before a validator is slashed
|
|
func (h Hooks) BeforeValidatorSlashed(ctx sdk.Context, valAddr sdk.ValAddress, fraction sdk.Dec) {}
|
|
|
|
// AfterValidatorBeginUnbonding is called after a validator begins unbonding
|
|
func (h Hooks) AfterValidatorBeginUnbonding(ctx sdk.Context, consAddr sdk.ConsAddress, valAddr sdk.ValAddress) {
|
|
}
|
|
|
|
// AfterValidatorBonded is called after a validator is bonded
|
|
func (h Hooks) AfterValidatorBonded(ctx sdk.Context, consAddr sdk.ConsAddress, valAddr sdk.ValAddress) {
|
|
}
|
|
|
|
// AfterDelegationModified runs after a delegation is modified
|
|
func (h Hooks) AfterDelegationModified(ctx sdk.Context, delAddr sdk.AccAddress, valAddr sdk.ValAddress) {
|
|
}
|
|
|
|
// BeforeDelegationRemoved runs directly before a delegation is deleted
|
|
func (h Hooks) BeforeDelegationRemoved(ctx sdk.Context, delAddr sdk.AccAddress, valAddr sdk.ValAddress) {
|
|
}
|
|
|
|
// AfterValidatorCreated runs after a validator is created
|
|
func (h Hooks) AfterValidatorCreated(ctx sdk.Context, valAddr sdk.ValAddress) {}
|
|
|
|
// BeforeValidatorModified runs before a validator is modified
|
|
func (h Hooks) BeforeValidatorModified(ctx sdk.Context, valAddr sdk.ValAddress) {}
|
|
|
|
// AfterValidatorRemoved runs after a validator is removed
|
|
func (h Hooks) AfterValidatorRemoved(ctx sdk.Context, consAddr sdk.ConsAddress, valAddr sdk.ValAddress) {
|
|
}
|
|
```
|
|
|
|
Swap module hooks manage the creation and synchronization of Swap protocol liquidity provider rewards.
|
|
|
|
```go
|
|
// ------------------- Swap Module Hooks -------------------
|
|
|
|
func (h Hooks) AfterPoolDepositCreated(ctx sdk.Context, poolID string, depositor sdk.AccAddress, _ sdkmath.Int) {
|
|
h.k.InitializeSwapReward(ctx, poolID, depositor)
|
|
}
|
|
|
|
func (h Hooks) BeforePoolDepositModified(ctx sdk.Context, poolID string, depositor sdk.AccAddress, sharesOwned sdkmath.Int) {
|
|
h.k.SynchronizeSwapReward(ctx, poolID, depositor, sharesOwned)
|
|
}
|
|
```
|