mirror of
https://source.quilibrium.com/quilibrium/ceremonyclient.git
synced 2024-09-20 23:17:31 +00:00
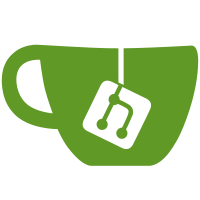
* add version label and trim repo url * add README for protobufs * add version to NodeInfoResponse * add docker login task * remove version from docker compose file * return version with GetNodeInfo response * add basic -node-info flag * print max frame as well with -node-info * expand protobuf README * update node command examples to use node binary and make log commands follow * return all of NodeInfo * extract FormatVersion * print version of running process
2382 lines
88 KiB
Go
2382 lines
88 KiB
Go
// Code generated by protoc-gen-go. DO NOT EDIT.
|
||
// versions:
|
||
// protoc-gen-go v1.30.0
|
||
// protoc v3.21.12
|
||
// source: node.proto
|
||
|
||
package protobufs
|
||
|
||
import (
|
||
protoreflect "google.golang.org/protobuf/reflect/protoreflect"
|
||
protoimpl "google.golang.org/protobuf/runtime/protoimpl"
|
||
reflect "reflect"
|
||
sync "sync"
|
||
)
|
||
|
||
const (
|
||
// Verify that this generated code is sufficiently up-to-date.
|
||
_ = protoimpl.EnforceVersion(20 - protoimpl.MinVersion)
|
||
// Verify that runtime/protoimpl is sufficiently up-to-date.
|
||
_ = protoimpl.EnforceVersion(protoimpl.MaxVersion - 20)
|
||
)
|
||
|
||
type GetFramesRequest struct {
|
||
state protoimpl.MessageState
|
||
sizeCache protoimpl.SizeCache
|
||
unknownFields protoimpl.UnknownFields
|
||
|
||
Filter []byte `protobuf:"bytes,1,opt,name=filter,proto3" json:"filter,omitempty"`
|
||
FromFrameNumber uint64 `protobuf:"varint,2,opt,name=from_frame_number,json=fromFrameNumber,proto3" json:"from_frame_number,omitempty"`
|
||
ToFrameNumber uint64 `protobuf:"varint,3,opt,name=to_frame_number,json=toFrameNumber,proto3" json:"to_frame_number,omitempty"`
|
||
IncludeCandidates bool `protobuf:"varint,4,opt,name=include_candidates,json=includeCandidates,proto3" json:"include_candidates,omitempty"`
|
||
}
|
||
|
||
func (x *GetFramesRequest) Reset() {
|
||
*x = GetFramesRequest{}
|
||
if protoimpl.UnsafeEnabled {
|
||
mi := &file_node_proto_msgTypes[0]
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
}
|
||
|
||
func (x *GetFramesRequest) String() string {
|
||
return protoimpl.X.MessageStringOf(x)
|
||
}
|
||
|
||
func (*GetFramesRequest) ProtoMessage() {}
|
||
|
||
func (x *GetFramesRequest) ProtoReflect() protoreflect.Message {
|
||
mi := &file_node_proto_msgTypes[0]
|
||
if protoimpl.UnsafeEnabled && x != nil {
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
if ms.LoadMessageInfo() == nil {
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
return ms
|
||
}
|
||
return mi.MessageOf(x)
|
||
}
|
||
|
||
// Deprecated: Use GetFramesRequest.ProtoReflect.Descriptor instead.
|
||
func (*GetFramesRequest) Descriptor() ([]byte, []int) {
|
||
return file_node_proto_rawDescGZIP(), []int{0}
|
||
}
|
||
|
||
func (x *GetFramesRequest) GetFilter() []byte {
|
||
if x != nil {
|
||
return x.Filter
|
||
}
|
||
return nil
|
||
}
|
||
|
||
func (x *GetFramesRequest) GetFromFrameNumber() uint64 {
|
||
if x != nil {
|
||
return x.FromFrameNumber
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *GetFramesRequest) GetToFrameNumber() uint64 {
|
||
if x != nil {
|
||
return x.ToFrameNumber
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *GetFramesRequest) GetIncludeCandidates() bool {
|
||
if x != nil {
|
||
return x.IncludeCandidates
|
||
}
|
||
return false
|
||
}
|
||
|
||
type GetFrameInfoRequest struct {
|
||
state protoimpl.MessageState
|
||
sizeCache protoimpl.SizeCache
|
||
unknownFields protoimpl.UnknownFields
|
||
|
||
Filter []byte `protobuf:"bytes,1,opt,name=filter,proto3" json:"filter,omitempty"`
|
||
FrameNumber uint64 `protobuf:"varint,2,opt,name=frame_number,json=frameNumber,proto3" json:"frame_number,omitempty"`
|
||
Selector []byte `protobuf:"bytes,3,opt,name=selector,proto3" json:"selector,omitempty"`
|
||
}
|
||
|
||
func (x *GetFrameInfoRequest) Reset() {
|
||
*x = GetFrameInfoRequest{}
|
||
if protoimpl.UnsafeEnabled {
|
||
mi := &file_node_proto_msgTypes[1]
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
}
|
||
|
||
func (x *GetFrameInfoRequest) String() string {
|
||
return protoimpl.X.MessageStringOf(x)
|
||
}
|
||
|
||
func (*GetFrameInfoRequest) ProtoMessage() {}
|
||
|
||
func (x *GetFrameInfoRequest) ProtoReflect() protoreflect.Message {
|
||
mi := &file_node_proto_msgTypes[1]
|
||
if protoimpl.UnsafeEnabled && x != nil {
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
if ms.LoadMessageInfo() == nil {
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
return ms
|
||
}
|
||
return mi.MessageOf(x)
|
||
}
|
||
|
||
// Deprecated: Use GetFrameInfoRequest.ProtoReflect.Descriptor instead.
|
||
func (*GetFrameInfoRequest) Descriptor() ([]byte, []int) {
|
||
return file_node_proto_rawDescGZIP(), []int{1}
|
||
}
|
||
|
||
func (x *GetFrameInfoRequest) GetFilter() []byte {
|
||
if x != nil {
|
||
return x.Filter
|
||
}
|
||
return nil
|
||
}
|
||
|
||
func (x *GetFrameInfoRequest) GetFrameNumber() uint64 {
|
||
if x != nil {
|
||
return x.FrameNumber
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *GetFrameInfoRequest) GetSelector() []byte {
|
||
if x != nil {
|
||
return x.Selector
|
||
}
|
||
return nil
|
||
}
|
||
|
||
type GetPeerInfoRequest struct {
|
||
state protoimpl.MessageState
|
||
sizeCache protoimpl.SizeCache
|
||
unknownFields protoimpl.UnknownFields
|
||
}
|
||
|
||
func (x *GetPeerInfoRequest) Reset() {
|
||
*x = GetPeerInfoRequest{}
|
||
if protoimpl.UnsafeEnabled {
|
||
mi := &file_node_proto_msgTypes[2]
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
}
|
||
|
||
func (x *GetPeerInfoRequest) String() string {
|
||
return protoimpl.X.MessageStringOf(x)
|
||
}
|
||
|
||
func (*GetPeerInfoRequest) ProtoMessage() {}
|
||
|
||
func (x *GetPeerInfoRequest) ProtoReflect() protoreflect.Message {
|
||
mi := &file_node_proto_msgTypes[2]
|
||
if protoimpl.UnsafeEnabled && x != nil {
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
if ms.LoadMessageInfo() == nil {
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
return ms
|
||
}
|
||
return mi.MessageOf(x)
|
||
}
|
||
|
||
// Deprecated: Use GetPeerInfoRequest.ProtoReflect.Descriptor instead.
|
||
func (*GetPeerInfoRequest) Descriptor() ([]byte, []int) {
|
||
return file_node_proto_rawDescGZIP(), []int{2}
|
||
}
|
||
|
||
type GetNodeInfoRequest struct {
|
||
state protoimpl.MessageState
|
||
sizeCache protoimpl.SizeCache
|
||
unknownFields protoimpl.UnknownFields
|
||
}
|
||
|
||
func (x *GetNodeInfoRequest) Reset() {
|
||
*x = GetNodeInfoRequest{}
|
||
if protoimpl.UnsafeEnabled {
|
||
mi := &file_node_proto_msgTypes[3]
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
}
|
||
|
||
func (x *GetNodeInfoRequest) String() string {
|
||
return protoimpl.X.MessageStringOf(x)
|
||
}
|
||
|
||
func (*GetNodeInfoRequest) ProtoMessage() {}
|
||
|
||
func (x *GetNodeInfoRequest) ProtoReflect() protoreflect.Message {
|
||
mi := &file_node_proto_msgTypes[3]
|
||
if protoimpl.UnsafeEnabled && x != nil {
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
if ms.LoadMessageInfo() == nil {
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
return ms
|
||
}
|
||
return mi.MessageOf(x)
|
||
}
|
||
|
||
// Deprecated: Use GetNodeInfoRequest.ProtoReflect.Descriptor instead.
|
||
func (*GetNodeInfoRequest) Descriptor() ([]byte, []int) {
|
||
return file_node_proto_rawDescGZIP(), []int{3}
|
||
}
|
||
|
||
type GetNetworkInfoRequest struct {
|
||
state protoimpl.MessageState
|
||
sizeCache protoimpl.SizeCache
|
||
unknownFields protoimpl.UnknownFields
|
||
}
|
||
|
||
func (x *GetNetworkInfoRequest) Reset() {
|
||
*x = GetNetworkInfoRequest{}
|
||
if protoimpl.UnsafeEnabled {
|
||
mi := &file_node_proto_msgTypes[4]
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
}
|
||
|
||
func (x *GetNetworkInfoRequest) String() string {
|
||
return protoimpl.X.MessageStringOf(x)
|
||
}
|
||
|
||
func (*GetNetworkInfoRequest) ProtoMessage() {}
|
||
|
||
func (x *GetNetworkInfoRequest) ProtoReflect() protoreflect.Message {
|
||
mi := &file_node_proto_msgTypes[4]
|
||
if protoimpl.UnsafeEnabled && x != nil {
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
if ms.LoadMessageInfo() == nil {
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
return ms
|
||
}
|
||
return mi.MessageOf(x)
|
||
}
|
||
|
||
// Deprecated: Use GetNetworkInfoRequest.ProtoReflect.Descriptor instead.
|
||
func (*GetNetworkInfoRequest) Descriptor() ([]byte, []int) {
|
||
return file_node_proto_rawDescGZIP(), []int{4}
|
||
}
|
||
|
||
type FramesResponse struct {
|
||
state protoimpl.MessageState
|
||
sizeCache protoimpl.SizeCache
|
||
unknownFields protoimpl.UnknownFields
|
||
|
||
TruncatedClockFrames []*ClockFrame `protobuf:"bytes,1,rep,name=truncated_clock_frames,json=truncatedClockFrames,proto3" json:"truncated_clock_frames,omitempty"`
|
||
}
|
||
|
||
func (x *FramesResponse) Reset() {
|
||
*x = FramesResponse{}
|
||
if protoimpl.UnsafeEnabled {
|
||
mi := &file_node_proto_msgTypes[5]
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
}
|
||
|
||
func (x *FramesResponse) String() string {
|
||
return protoimpl.X.MessageStringOf(x)
|
||
}
|
||
|
||
func (*FramesResponse) ProtoMessage() {}
|
||
|
||
func (x *FramesResponse) ProtoReflect() protoreflect.Message {
|
||
mi := &file_node_proto_msgTypes[5]
|
||
if protoimpl.UnsafeEnabled && x != nil {
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
if ms.LoadMessageInfo() == nil {
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
return ms
|
||
}
|
||
return mi.MessageOf(x)
|
||
}
|
||
|
||
// Deprecated: Use FramesResponse.ProtoReflect.Descriptor instead.
|
||
func (*FramesResponse) Descriptor() ([]byte, []int) {
|
||
return file_node_proto_rawDescGZIP(), []int{5}
|
||
}
|
||
|
||
func (x *FramesResponse) GetTruncatedClockFrames() []*ClockFrame {
|
||
if x != nil {
|
||
return x.TruncatedClockFrames
|
||
}
|
||
return nil
|
||
}
|
||
|
||
type FrameInfoResponse struct {
|
||
state protoimpl.MessageState
|
||
sizeCache protoimpl.SizeCache
|
||
unknownFields protoimpl.UnknownFields
|
||
|
||
ClockFrame *ClockFrame `protobuf:"bytes,1,opt,name=clock_frame,json=clockFrame,proto3" json:"clock_frame,omitempty"`
|
||
}
|
||
|
||
func (x *FrameInfoResponse) Reset() {
|
||
*x = FrameInfoResponse{}
|
||
if protoimpl.UnsafeEnabled {
|
||
mi := &file_node_proto_msgTypes[6]
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
}
|
||
|
||
func (x *FrameInfoResponse) String() string {
|
||
return protoimpl.X.MessageStringOf(x)
|
||
}
|
||
|
||
func (*FrameInfoResponse) ProtoMessage() {}
|
||
|
||
func (x *FrameInfoResponse) ProtoReflect() protoreflect.Message {
|
||
mi := &file_node_proto_msgTypes[6]
|
||
if protoimpl.UnsafeEnabled && x != nil {
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
if ms.LoadMessageInfo() == nil {
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
return ms
|
||
}
|
||
return mi.MessageOf(x)
|
||
}
|
||
|
||
// Deprecated: Use FrameInfoResponse.ProtoReflect.Descriptor instead.
|
||
func (*FrameInfoResponse) Descriptor() ([]byte, []int) {
|
||
return file_node_proto_rawDescGZIP(), []int{6}
|
||
}
|
||
|
||
func (x *FrameInfoResponse) GetClockFrame() *ClockFrame {
|
||
if x != nil {
|
||
return x.ClockFrame
|
||
}
|
||
return nil
|
||
}
|
||
|
||
type PeerInfo struct {
|
||
state protoimpl.MessageState
|
||
sizeCache protoimpl.SizeCache
|
||
unknownFields protoimpl.UnknownFields
|
||
|
||
PeerId []byte `protobuf:"bytes,1,opt,name=peer_id,json=peerId,proto3" json:"peer_id,omitempty"`
|
||
Multiaddrs []string `protobuf:"bytes,2,rep,name=multiaddrs,proto3" json:"multiaddrs,omitempty"`
|
||
MaxFrame uint64 `protobuf:"varint,3,opt,name=max_frame,json=maxFrame,proto3" json:"max_frame,omitempty"`
|
||
Timestamp int64 `protobuf:"varint,4,opt,name=timestamp,proto3" json:"timestamp,omitempty"`
|
||
Version []byte `protobuf:"bytes,5,opt,name=version,proto3" json:"version,omitempty"`
|
||
Signature []byte `protobuf:"bytes,6,opt,name=signature,proto3" json:"signature,omitempty"`
|
||
PublicKey []byte `protobuf:"bytes,7,opt,name=public_key,json=publicKey,proto3" json:"public_key,omitempty"`
|
||
TotalDistance []byte `protobuf:"bytes,8,opt,name=total_distance,json=totalDistance,proto3" json:"total_distance,omitempty"`
|
||
}
|
||
|
||
func (x *PeerInfo) Reset() {
|
||
*x = PeerInfo{}
|
||
if protoimpl.UnsafeEnabled {
|
||
mi := &file_node_proto_msgTypes[7]
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
}
|
||
|
||
func (x *PeerInfo) String() string {
|
||
return protoimpl.X.MessageStringOf(x)
|
||
}
|
||
|
||
func (*PeerInfo) ProtoMessage() {}
|
||
|
||
func (x *PeerInfo) ProtoReflect() protoreflect.Message {
|
||
mi := &file_node_proto_msgTypes[7]
|
||
if protoimpl.UnsafeEnabled && x != nil {
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
if ms.LoadMessageInfo() == nil {
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
return ms
|
||
}
|
||
return mi.MessageOf(x)
|
||
}
|
||
|
||
// Deprecated: Use PeerInfo.ProtoReflect.Descriptor instead.
|
||
func (*PeerInfo) Descriptor() ([]byte, []int) {
|
||
return file_node_proto_rawDescGZIP(), []int{7}
|
||
}
|
||
|
||
func (x *PeerInfo) GetPeerId() []byte {
|
||
if x != nil {
|
||
return x.PeerId
|
||
}
|
||
return nil
|
||
}
|
||
|
||
func (x *PeerInfo) GetMultiaddrs() []string {
|
||
if x != nil {
|
||
return x.Multiaddrs
|
||
}
|
||
return nil
|
||
}
|
||
|
||
func (x *PeerInfo) GetMaxFrame() uint64 {
|
||
if x != nil {
|
||
return x.MaxFrame
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *PeerInfo) GetTimestamp() int64 {
|
||
if x != nil {
|
||
return x.Timestamp
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *PeerInfo) GetVersion() []byte {
|
||
if x != nil {
|
||
return x.Version
|
||
}
|
||
return nil
|
||
}
|
||
|
||
func (x *PeerInfo) GetSignature() []byte {
|
||
if x != nil {
|
||
return x.Signature
|
||
}
|
||
return nil
|
||
}
|
||
|
||
func (x *PeerInfo) GetPublicKey() []byte {
|
||
if x != nil {
|
||
return x.PublicKey
|
||
}
|
||
return nil
|
||
}
|
||
|
||
func (x *PeerInfo) GetTotalDistance() []byte {
|
||
if x != nil {
|
||
return x.TotalDistance
|
||
}
|
||
return nil
|
||
}
|
||
|
||
type PeerInfoResponse struct {
|
||
state protoimpl.MessageState
|
||
sizeCache protoimpl.SizeCache
|
||
unknownFields protoimpl.UnknownFields
|
||
|
||
PeerInfo []*PeerInfo `protobuf:"bytes,1,rep,name=peer_info,json=peerInfo,proto3" json:"peer_info,omitempty"`
|
||
UncooperativePeerInfo []*PeerInfo `protobuf:"bytes,2,rep,name=uncooperative_peer_info,json=uncooperativePeerInfo,proto3" json:"uncooperative_peer_info,omitempty"`
|
||
}
|
||
|
||
func (x *PeerInfoResponse) Reset() {
|
||
*x = PeerInfoResponse{}
|
||
if protoimpl.UnsafeEnabled {
|
||
mi := &file_node_proto_msgTypes[8]
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
}
|
||
|
||
func (x *PeerInfoResponse) String() string {
|
||
return protoimpl.X.MessageStringOf(x)
|
||
}
|
||
|
||
func (*PeerInfoResponse) ProtoMessage() {}
|
||
|
||
func (x *PeerInfoResponse) ProtoReflect() protoreflect.Message {
|
||
mi := &file_node_proto_msgTypes[8]
|
||
if protoimpl.UnsafeEnabled && x != nil {
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
if ms.LoadMessageInfo() == nil {
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
return ms
|
||
}
|
||
return mi.MessageOf(x)
|
||
}
|
||
|
||
// Deprecated: Use PeerInfoResponse.ProtoReflect.Descriptor instead.
|
||
func (*PeerInfoResponse) Descriptor() ([]byte, []int) {
|
||
return file_node_proto_rawDescGZIP(), []int{8}
|
||
}
|
||
|
||
func (x *PeerInfoResponse) GetPeerInfo() []*PeerInfo {
|
||
if x != nil {
|
||
return x.PeerInfo
|
||
}
|
||
return nil
|
||
}
|
||
|
||
func (x *PeerInfoResponse) GetUncooperativePeerInfo() []*PeerInfo {
|
||
if x != nil {
|
||
return x.UncooperativePeerInfo
|
||
}
|
||
return nil
|
||
}
|
||
|
||
type NetworkInfo struct {
|
||
state protoimpl.MessageState
|
||
sizeCache protoimpl.SizeCache
|
||
unknownFields protoimpl.UnknownFields
|
||
|
||
PeerId []byte `protobuf:"bytes,1,opt,name=peer_id,json=peerId,proto3" json:"peer_id,omitempty"`
|
||
Multiaddrs []string `protobuf:"bytes,2,rep,name=multiaddrs,proto3" json:"multiaddrs,omitempty"`
|
||
PeerScore float64 `protobuf:"fixed64,3,opt,name=peer_score,json=peerScore,proto3" json:"peer_score,omitempty"`
|
||
}
|
||
|
||
func (x *NetworkInfo) Reset() {
|
||
*x = NetworkInfo{}
|
||
if protoimpl.UnsafeEnabled {
|
||
mi := &file_node_proto_msgTypes[9]
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
}
|
||
|
||
func (x *NetworkInfo) String() string {
|
||
return protoimpl.X.MessageStringOf(x)
|
||
}
|
||
|
||
func (*NetworkInfo) ProtoMessage() {}
|
||
|
||
func (x *NetworkInfo) ProtoReflect() protoreflect.Message {
|
||
mi := &file_node_proto_msgTypes[9]
|
||
if protoimpl.UnsafeEnabled && x != nil {
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
if ms.LoadMessageInfo() == nil {
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
return ms
|
||
}
|
||
return mi.MessageOf(x)
|
||
}
|
||
|
||
// Deprecated: Use NetworkInfo.ProtoReflect.Descriptor instead.
|
||
func (*NetworkInfo) Descriptor() ([]byte, []int) {
|
||
return file_node_proto_rawDescGZIP(), []int{9}
|
||
}
|
||
|
||
func (x *NetworkInfo) GetPeerId() []byte {
|
||
if x != nil {
|
||
return x.PeerId
|
||
}
|
||
return nil
|
||
}
|
||
|
||
func (x *NetworkInfo) GetMultiaddrs() []string {
|
||
if x != nil {
|
||
return x.Multiaddrs
|
||
}
|
||
return nil
|
||
}
|
||
|
||
func (x *NetworkInfo) GetPeerScore() float64 {
|
||
if x != nil {
|
||
return x.PeerScore
|
||
}
|
||
return 0
|
||
}
|
||
|
||
type NodeInfoResponse struct {
|
||
state protoimpl.MessageState
|
||
sizeCache protoimpl.SizeCache
|
||
unknownFields protoimpl.UnknownFields
|
||
|
||
PeerId string `protobuf:"bytes,1,opt,name=peer_id,json=peerId,proto3" json:"peer_id,omitempty"`
|
||
MaxFrame uint64 `protobuf:"varint,2,opt,name=max_frame,json=maxFrame,proto3" json:"max_frame,omitempty"`
|
||
PeerScore uint64 `protobuf:"varint,3,opt,name=peer_score,json=peerScore,proto3" json:"peer_score,omitempty"`
|
||
Version []byte `protobuf:"bytes,4,opt,name=version,proto3" json:"version,omitempty"`
|
||
}
|
||
|
||
func (x *NodeInfoResponse) Reset() {
|
||
*x = NodeInfoResponse{}
|
||
if protoimpl.UnsafeEnabled {
|
||
mi := &file_node_proto_msgTypes[10]
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
}
|
||
|
||
func (x *NodeInfoResponse) String() string {
|
||
return protoimpl.X.MessageStringOf(x)
|
||
}
|
||
|
||
func (*NodeInfoResponse) ProtoMessage() {}
|
||
|
||
func (x *NodeInfoResponse) ProtoReflect() protoreflect.Message {
|
||
mi := &file_node_proto_msgTypes[10]
|
||
if protoimpl.UnsafeEnabled && x != nil {
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
if ms.LoadMessageInfo() == nil {
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
return ms
|
||
}
|
||
return mi.MessageOf(x)
|
||
}
|
||
|
||
// Deprecated: Use NodeInfoResponse.ProtoReflect.Descriptor instead.
|
||
func (*NodeInfoResponse) Descriptor() ([]byte, []int) {
|
||
return file_node_proto_rawDescGZIP(), []int{10}
|
||
}
|
||
|
||
func (x *NodeInfoResponse) GetPeerId() string {
|
||
if x != nil {
|
||
return x.PeerId
|
||
}
|
||
return ""
|
||
}
|
||
|
||
func (x *NodeInfoResponse) GetMaxFrame() uint64 {
|
||
if x != nil {
|
||
return x.MaxFrame
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *NodeInfoResponse) GetPeerScore() uint64 {
|
||
if x != nil {
|
||
return x.PeerScore
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *NodeInfoResponse) GetVersion() []byte {
|
||
if x != nil {
|
||
return x.Version
|
||
}
|
||
return nil
|
||
}
|
||
|
||
type PutPeerInfoRequest struct {
|
||
state protoimpl.MessageState
|
||
sizeCache protoimpl.SizeCache
|
||
unknownFields protoimpl.UnknownFields
|
||
|
||
PeerInfo []*PeerInfo `protobuf:"bytes,1,rep,name=peer_info,json=peerInfo,proto3" json:"peer_info,omitempty"`
|
||
UncooperativePeerInfo []*PeerInfo `protobuf:"bytes,2,rep,name=uncooperative_peer_info,json=uncooperativePeerInfo,proto3" json:"uncooperative_peer_info,omitempty"`
|
||
}
|
||
|
||
func (x *PutPeerInfoRequest) Reset() {
|
||
*x = PutPeerInfoRequest{}
|
||
if protoimpl.UnsafeEnabled {
|
||
mi := &file_node_proto_msgTypes[11]
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
}
|
||
|
||
func (x *PutPeerInfoRequest) String() string {
|
||
return protoimpl.X.MessageStringOf(x)
|
||
}
|
||
|
||
func (*PutPeerInfoRequest) ProtoMessage() {}
|
||
|
||
func (x *PutPeerInfoRequest) ProtoReflect() protoreflect.Message {
|
||
mi := &file_node_proto_msgTypes[11]
|
||
if protoimpl.UnsafeEnabled && x != nil {
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
if ms.LoadMessageInfo() == nil {
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
return ms
|
||
}
|
||
return mi.MessageOf(x)
|
||
}
|
||
|
||
// Deprecated: Use PutPeerInfoRequest.ProtoReflect.Descriptor instead.
|
||
func (*PutPeerInfoRequest) Descriptor() ([]byte, []int) {
|
||
return file_node_proto_rawDescGZIP(), []int{11}
|
||
}
|
||
|
||
func (x *PutPeerInfoRequest) GetPeerInfo() []*PeerInfo {
|
||
if x != nil {
|
||
return x.PeerInfo
|
||
}
|
||
return nil
|
||
}
|
||
|
||
func (x *PutPeerInfoRequest) GetUncooperativePeerInfo() []*PeerInfo {
|
||
if x != nil {
|
||
return x.UncooperativePeerInfo
|
||
}
|
||
return nil
|
||
}
|
||
|
||
type PutNodeInfoRequest struct {
|
||
state protoimpl.MessageState
|
||
sizeCache protoimpl.SizeCache
|
||
unknownFields protoimpl.UnknownFields
|
||
|
||
PeerId string `protobuf:"bytes,1,opt,name=peer_id,json=peerId,proto3" json:"peer_id,omitempty"`
|
||
MaxFrame uint64 `protobuf:"varint,2,opt,name=max_frame,json=maxFrame,proto3" json:"max_frame,omitempty"`
|
||
PeerScore uint64 `protobuf:"varint,3,opt,name=peer_score,json=peerScore,proto3" json:"peer_score,omitempty"`
|
||
Signature []byte `protobuf:"bytes,4,opt,name=signature,proto3" json:"signature,omitempty"`
|
||
}
|
||
|
||
func (x *PutNodeInfoRequest) Reset() {
|
||
*x = PutNodeInfoRequest{}
|
||
if protoimpl.UnsafeEnabled {
|
||
mi := &file_node_proto_msgTypes[12]
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
}
|
||
|
||
func (x *PutNodeInfoRequest) String() string {
|
||
return protoimpl.X.MessageStringOf(x)
|
||
}
|
||
|
||
func (*PutNodeInfoRequest) ProtoMessage() {}
|
||
|
||
func (x *PutNodeInfoRequest) ProtoReflect() protoreflect.Message {
|
||
mi := &file_node_proto_msgTypes[12]
|
||
if protoimpl.UnsafeEnabled && x != nil {
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
if ms.LoadMessageInfo() == nil {
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
return ms
|
||
}
|
||
return mi.MessageOf(x)
|
||
}
|
||
|
||
// Deprecated: Use PutNodeInfoRequest.ProtoReflect.Descriptor instead.
|
||
func (*PutNodeInfoRequest) Descriptor() ([]byte, []int) {
|
||
return file_node_proto_rawDescGZIP(), []int{12}
|
||
}
|
||
|
||
func (x *PutNodeInfoRequest) GetPeerId() string {
|
||
if x != nil {
|
||
return x.PeerId
|
||
}
|
||
return ""
|
||
}
|
||
|
||
func (x *PutNodeInfoRequest) GetMaxFrame() uint64 {
|
||
if x != nil {
|
||
return x.MaxFrame
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *PutNodeInfoRequest) GetPeerScore() uint64 {
|
||
if x != nil {
|
||
return x.PeerScore
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *PutNodeInfoRequest) GetSignature() []byte {
|
||
if x != nil {
|
||
return x.Signature
|
||
}
|
||
return nil
|
||
}
|
||
|
||
type PutResponse struct {
|
||
state protoimpl.MessageState
|
||
sizeCache protoimpl.SizeCache
|
||
unknownFields protoimpl.UnknownFields
|
||
}
|
||
|
||
func (x *PutResponse) Reset() {
|
||
*x = PutResponse{}
|
||
if protoimpl.UnsafeEnabled {
|
||
mi := &file_node_proto_msgTypes[13]
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
}
|
||
|
||
func (x *PutResponse) String() string {
|
||
return protoimpl.X.MessageStringOf(x)
|
||
}
|
||
|
||
func (*PutResponse) ProtoMessage() {}
|
||
|
||
func (x *PutResponse) ProtoReflect() protoreflect.Message {
|
||
mi := &file_node_proto_msgTypes[13]
|
||
if protoimpl.UnsafeEnabled && x != nil {
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
if ms.LoadMessageInfo() == nil {
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
return ms
|
||
}
|
||
return mi.MessageOf(x)
|
||
}
|
||
|
||
// Deprecated: Use PutResponse.ProtoReflect.Descriptor instead.
|
||
func (*PutResponse) Descriptor() ([]byte, []int) {
|
||
return file_node_proto_rawDescGZIP(), []int{13}
|
||
}
|
||
|
||
type NetworkInfoResponse struct {
|
||
state protoimpl.MessageState
|
||
sizeCache protoimpl.SizeCache
|
||
unknownFields protoimpl.UnknownFields
|
||
|
||
NetworkInfo []*NetworkInfo `protobuf:"bytes,1,rep,name=network_info,json=networkInfo,proto3" json:"network_info,omitempty"`
|
||
}
|
||
|
||
func (x *NetworkInfoResponse) Reset() {
|
||
*x = NetworkInfoResponse{}
|
||
if protoimpl.UnsafeEnabled {
|
||
mi := &file_node_proto_msgTypes[14]
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
}
|
||
|
||
func (x *NetworkInfoResponse) String() string {
|
||
return protoimpl.X.MessageStringOf(x)
|
||
}
|
||
|
||
func (*NetworkInfoResponse) ProtoMessage() {}
|
||
|
||
func (x *NetworkInfoResponse) ProtoReflect() protoreflect.Message {
|
||
mi := &file_node_proto_msgTypes[14]
|
||
if protoimpl.UnsafeEnabled && x != nil {
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
if ms.LoadMessageInfo() == nil {
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
return ms
|
||
}
|
||
return mi.MessageOf(x)
|
||
}
|
||
|
||
// Deprecated: Use NetworkInfoResponse.ProtoReflect.Descriptor instead.
|
||
func (*NetworkInfoResponse) Descriptor() ([]byte, []int) {
|
||
return file_node_proto_rawDescGZIP(), []int{14}
|
||
}
|
||
|
||
func (x *NetworkInfoResponse) GetNetworkInfo() []*NetworkInfo {
|
||
if x != nil {
|
||
return x.NetworkInfo
|
||
}
|
||
return nil
|
||
}
|
||
|
||
type GetTokenInfoRequest struct {
|
||
state protoimpl.MessageState
|
||
sizeCache protoimpl.SizeCache
|
||
unknownFields protoimpl.UnknownFields
|
||
}
|
||
|
||
func (x *GetTokenInfoRequest) Reset() {
|
||
*x = GetTokenInfoRequest{}
|
||
if protoimpl.UnsafeEnabled {
|
||
mi := &file_node_proto_msgTypes[15]
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
}
|
||
|
||
func (x *GetTokenInfoRequest) String() string {
|
||
return protoimpl.X.MessageStringOf(x)
|
||
}
|
||
|
||
func (*GetTokenInfoRequest) ProtoMessage() {}
|
||
|
||
func (x *GetTokenInfoRequest) ProtoReflect() protoreflect.Message {
|
||
mi := &file_node_proto_msgTypes[15]
|
||
if protoimpl.UnsafeEnabled && x != nil {
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
if ms.LoadMessageInfo() == nil {
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
return ms
|
||
}
|
||
return mi.MessageOf(x)
|
||
}
|
||
|
||
// Deprecated: Use GetTokenInfoRequest.ProtoReflect.Descriptor instead.
|
||
func (*GetTokenInfoRequest) Descriptor() ([]byte, []int) {
|
||
return file_node_proto_rawDescGZIP(), []int{15}
|
||
}
|
||
|
||
type TokenInfoResponse struct {
|
||
state protoimpl.MessageState
|
||
sizeCache protoimpl.SizeCache
|
||
unknownFields protoimpl.UnknownFields
|
||
|
||
// Total active token supply, as a 256 bit integer representing maximum
|
||
// divisble units. 1 QUIL = 8000000000 units, 50 QUIL would be represented by
|
||
// 0x0000000000000000000000000000000000000000000000000000005D21DBA000
|
||
ConfirmedTokenSupply []byte `protobuf:"bytes,1,opt,name=confirmed_token_supply,json=confirmedTokenSupply,proto3" json:"confirmed_token_supply,omitempty"`
|
||
// Total token supply, including unconfirmed frame data, as a 256 bit integer.
|
||
UnconfirmedTokenSupply []byte `protobuf:"bytes,2,opt,name=unconfirmed_token_supply,json=unconfirmedTokenSupply,proto3" json:"unconfirmed_token_supply,omitempty"`
|
||
// The total number of tokens owned by the prover address associated with the
|
||
// node.
|
||
OwnedTokens []byte `protobuf:"bytes,3,opt,name=owned_tokens,json=ownedTokens,proto3" json:"owned_tokens,omitempty"`
|
||
// The total number of tokens owned by the prover address associated with the
|
||
// node, including unconfirmed frame data.
|
||
UnconfirmedOwnedTokens []byte `protobuf:"bytes,4,opt,name=unconfirmed_owned_tokens,json=unconfirmedOwnedTokens,proto3" json:"unconfirmed_owned_tokens,omitempty"`
|
||
}
|
||
|
||
func (x *TokenInfoResponse) Reset() {
|
||
*x = TokenInfoResponse{}
|
||
if protoimpl.UnsafeEnabled {
|
||
mi := &file_node_proto_msgTypes[16]
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
}
|
||
|
||
func (x *TokenInfoResponse) String() string {
|
||
return protoimpl.X.MessageStringOf(x)
|
||
}
|
||
|
||
func (*TokenInfoResponse) ProtoMessage() {}
|
||
|
||
func (x *TokenInfoResponse) ProtoReflect() protoreflect.Message {
|
||
mi := &file_node_proto_msgTypes[16]
|
||
if protoimpl.UnsafeEnabled && x != nil {
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
if ms.LoadMessageInfo() == nil {
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
return ms
|
||
}
|
||
return mi.MessageOf(x)
|
||
}
|
||
|
||
// Deprecated: Use TokenInfoResponse.ProtoReflect.Descriptor instead.
|
||
func (*TokenInfoResponse) Descriptor() ([]byte, []int) {
|
||
return file_node_proto_rawDescGZIP(), []int{16}
|
||
}
|
||
|
||
func (x *TokenInfoResponse) GetConfirmedTokenSupply() []byte {
|
||
if x != nil {
|
||
return x.ConfirmedTokenSupply
|
||
}
|
||
return nil
|
||
}
|
||
|
||
func (x *TokenInfoResponse) GetUnconfirmedTokenSupply() []byte {
|
||
if x != nil {
|
||
return x.UnconfirmedTokenSupply
|
||
}
|
||
return nil
|
||
}
|
||
|
||
func (x *TokenInfoResponse) GetOwnedTokens() []byte {
|
||
if x != nil {
|
||
return x.OwnedTokens
|
||
}
|
||
return nil
|
||
}
|
||
|
||
func (x *TokenInfoResponse) GetUnconfirmedOwnedTokens() []byte {
|
||
if x != nil {
|
||
return x.UnconfirmedOwnedTokens
|
||
}
|
||
return nil
|
||
}
|
||
|
||
type Capability struct {
|
||
state protoimpl.MessageState
|
||
sizeCache protoimpl.SizeCache
|
||
unknownFields protoimpl.UnknownFields
|
||
|
||
// A general protocol identifier as a uint32 – this is expected to rarely
|
||
// iterate, and should be uniquely identifying both protocol and version.
|
||
// Pragmatically speaking, this implies that the least significant byte
|
||
// specifies version (which should iterate most minimally), and the three most
|
||
// significant bytes should specify protocol. Recipients SHOULD ignore
|
||
// messages with incompatible protocol identifiers, but also SHOULD warn on
|
||
// identifiers with versions higher than the supported protocol. A large
|
||
// number of unsupported protocol messages may indicate spam/some other
|
||
// attack, whereas a large number of unsupported protocol versions may
|
||
// indicate an out of date client, respective to which side is the maximum of
|
||
// the version number.
|
||
ProtocolIdentifier uint32 `protobuf:"varint,1,opt,name=protocol_identifier,json=protocolIdentifier,proto3" json:"protocol_identifier,omitempty"`
|
||
// An optional bundle of information specific to the capability – used for
|
||
// extensibility in negotiating variations of a protocol – e.g. Triple-Ratchet
|
||
// but only for certain curve types.
|
||
AdditionalMetadata []byte `protobuf:"bytes,2,opt,name=additional_metadata,json=additionalMetadata,proto3" json:"additional_metadata,omitempty"`
|
||
}
|
||
|
||
func (x *Capability) Reset() {
|
||
*x = Capability{}
|
||
if protoimpl.UnsafeEnabled {
|
||
mi := &file_node_proto_msgTypes[17]
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
}
|
||
|
||
func (x *Capability) String() string {
|
||
return protoimpl.X.MessageStringOf(x)
|
||
}
|
||
|
||
func (*Capability) ProtoMessage() {}
|
||
|
||
func (x *Capability) ProtoReflect() protoreflect.Message {
|
||
mi := &file_node_proto_msgTypes[17]
|
||
if protoimpl.UnsafeEnabled && x != nil {
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
if ms.LoadMessageInfo() == nil {
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
return ms
|
||
}
|
||
return mi.MessageOf(x)
|
||
}
|
||
|
||
// Deprecated: Use Capability.ProtoReflect.Descriptor instead.
|
||
func (*Capability) Descriptor() ([]byte, []int) {
|
||
return file_node_proto_rawDescGZIP(), []int{17}
|
||
}
|
||
|
||
func (x *Capability) GetProtocolIdentifier() uint32 {
|
||
if x != nil {
|
||
return x.ProtocolIdentifier
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *Capability) GetAdditionalMetadata() []byte {
|
||
if x != nil {
|
||
return x.AdditionalMetadata
|
||
}
|
||
return nil
|
||
}
|
||
|
||
type SelfTestReport struct {
|
||
state protoimpl.MessageState
|
||
sizeCache protoimpl.SizeCache
|
||
unknownFields protoimpl.UnknownFields
|
||
|
||
// The difficulty the self test was conducted under
|
||
Difficulty uint32 `protobuf:"varint,1,opt,name=difficulty,proto3" json:"difficulty,omitempty"`
|
||
// The resulting local time of computing the VDF test under the difficulty
|
||
DifficultyMetric int64 `protobuf:"varint,2,opt,name=difficulty_metric,json=difficultyMetric,proto3" json:"difficulty_metric,omitempty"`
|
||
// The resulting local time of computing a KZG commitment for a 16 degree
|
||
// polynomial
|
||
Commit_16Metric int64 `protobuf:"varint,3,opt,name=commit_16_metric,json=commit16Metric,proto3" json:"commit_16_metric,omitempty"`
|
||
// The resulting local time of computing a KZG commitment for a 128 degree
|
||
// polynomial
|
||
Commit_128Metric int64 `protobuf:"varint,4,opt,name=commit_128_metric,json=commit128Metric,proto3" json:"commit_128_metric,omitempty"`
|
||
// The resulting local time of computing a KZG commitment for a 1024 degree
|
||
// polynomial
|
||
Commit_1024Metric int64 `protobuf:"varint,5,opt,name=commit_1024_metric,json=commit1024Metric,proto3" json:"commit_1024_metric,omitempty"`
|
||
// The resulting local time of computing a KZG commitment for a 65536 degree
|
||
// polynomial
|
||
Commit_65536Metric int64 `protobuf:"varint,6,opt,name=commit_65536_metric,json=commit65536Metric,proto3" json:"commit_65536_metric,omitempty"`
|
||
// The resulting local time of computing a KZG proof for a 16 degree
|
||
// polynomial
|
||
Proof_16Metric int64 `protobuf:"varint,7,opt,name=proof_16_metric,json=proof16Metric,proto3" json:"proof_16_metric,omitempty"`
|
||
// The resulting local time of computing a KZG proof for a 128 degree
|
||
// polynomial
|
||
Proof_128Metric int64 `protobuf:"varint,8,opt,name=proof_128_metric,json=proof128Metric,proto3" json:"proof_128_metric,omitempty"`
|
||
// The resulting local time of computing a KZG proof for a 1024 degree
|
||
// polynomial
|
||
Proof_1024Metric int64 `protobuf:"varint,9,opt,name=proof_1024_metric,json=proof1024Metric,proto3" json:"proof_1024_metric,omitempty"`
|
||
// The resulting local time of computing a KZG proof for a 65536 degree
|
||
// polynomial
|
||
Proof_65536Metric int64 `protobuf:"varint,10,opt,name=proof_65536_metric,json=proof65536Metric,proto3" json:"proof_65536_metric,omitempty"`
|
||
// The number of reported accessible cores
|
||
Cores uint32 `protobuf:"varint,11,opt,name=cores,proto3" json:"cores,omitempty"`
|
||
// The total available memory
|
||
Memory []byte `protobuf:"bytes,12,opt,name=memory,proto3" json:"memory,omitempty"`
|
||
// The total available storage
|
||
Storage []byte `protobuf:"bytes,13,opt,name=storage,proto3" json:"storage,omitempty"`
|
||
// The list of supported capabilities
|
||
Capabilities []*Capability `protobuf:"bytes,14,rep,name=capabilities,proto3" json:"capabilities,omitempty"`
|
||
// The highest master frame the node has
|
||
MasterHeadFrame uint64 `protobuf:"varint,15,opt,name=master_head_frame,json=masterHeadFrame,proto3" json:"master_head_frame,omitempty"`
|
||
}
|
||
|
||
func (x *SelfTestReport) Reset() {
|
||
*x = SelfTestReport{}
|
||
if protoimpl.UnsafeEnabled {
|
||
mi := &file_node_proto_msgTypes[18]
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
}
|
||
|
||
func (x *SelfTestReport) String() string {
|
||
return protoimpl.X.MessageStringOf(x)
|
||
}
|
||
|
||
func (*SelfTestReport) ProtoMessage() {}
|
||
|
||
func (x *SelfTestReport) ProtoReflect() protoreflect.Message {
|
||
mi := &file_node_proto_msgTypes[18]
|
||
if protoimpl.UnsafeEnabled && x != nil {
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
if ms.LoadMessageInfo() == nil {
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
return ms
|
||
}
|
||
return mi.MessageOf(x)
|
||
}
|
||
|
||
// Deprecated: Use SelfTestReport.ProtoReflect.Descriptor instead.
|
||
func (*SelfTestReport) Descriptor() ([]byte, []int) {
|
||
return file_node_proto_rawDescGZIP(), []int{18}
|
||
}
|
||
|
||
func (x *SelfTestReport) GetDifficulty() uint32 {
|
||
if x != nil {
|
||
return x.Difficulty
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *SelfTestReport) GetDifficultyMetric() int64 {
|
||
if x != nil {
|
||
return x.DifficultyMetric
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *SelfTestReport) GetCommit_16Metric() int64 {
|
||
if x != nil {
|
||
return x.Commit_16Metric
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *SelfTestReport) GetCommit_128Metric() int64 {
|
||
if x != nil {
|
||
return x.Commit_128Metric
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *SelfTestReport) GetCommit_1024Metric() int64 {
|
||
if x != nil {
|
||
return x.Commit_1024Metric
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *SelfTestReport) GetCommit_65536Metric() int64 {
|
||
if x != nil {
|
||
return x.Commit_65536Metric
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *SelfTestReport) GetProof_16Metric() int64 {
|
||
if x != nil {
|
||
return x.Proof_16Metric
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *SelfTestReport) GetProof_128Metric() int64 {
|
||
if x != nil {
|
||
return x.Proof_128Metric
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *SelfTestReport) GetProof_1024Metric() int64 {
|
||
if x != nil {
|
||
return x.Proof_1024Metric
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *SelfTestReport) GetProof_65536Metric() int64 {
|
||
if x != nil {
|
||
return x.Proof_65536Metric
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *SelfTestReport) GetCores() uint32 {
|
||
if x != nil {
|
||
return x.Cores
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *SelfTestReport) GetMemory() []byte {
|
||
if x != nil {
|
||
return x.Memory
|
||
}
|
||
return nil
|
||
}
|
||
|
||
func (x *SelfTestReport) GetStorage() []byte {
|
||
if x != nil {
|
||
return x.Storage
|
||
}
|
||
return nil
|
||
}
|
||
|
||
func (x *SelfTestReport) GetCapabilities() []*Capability {
|
||
if x != nil {
|
||
return x.Capabilities
|
||
}
|
||
return nil
|
||
}
|
||
|
||
func (x *SelfTestReport) GetMasterHeadFrame() uint64 {
|
||
if x != nil {
|
||
return x.MasterHeadFrame
|
||
}
|
||
return 0
|
||
}
|
||
|
||
type ValidationMessage struct {
|
||
state protoimpl.MessageState
|
||
sizeCache protoimpl.SizeCache
|
||
unknownFields protoimpl.UnknownFields
|
||
|
||
Validation []byte `protobuf:"bytes,1,opt,name=validation,proto3" json:"validation,omitempty"`
|
||
}
|
||
|
||
func (x *ValidationMessage) Reset() {
|
||
*x = ValidationMessage{}
|
||
if protoimpl.UnsafeEnabled {
|
||
mi := &file_node_proto_msgTypes[19]
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
}
|
||
|
||
func (x *ValidationMessage) String() string {
|
||
return protoimpl.X.MessageStringOf(x)
|
||
}
|
||
|
||
func (*ValidationMessage) ProtoMessage() {}
|
||
|
||
func (x *ValidationMessage) ProtoReflect() protoreflect.Message {
|
||
mi := &file_node_proto_msgTypes[19]
|
||
if protoimpl.UnsafeEnabled && x != nil {
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
if ms.LoadMessageInfo() == nil {
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
return ms
|
||
}
|
||
return mi.MessageOf(x)
|
||
}
|
||
|
||
// Deprecated: Use ValidationMessage.ProtoReflect.Descriptor instead.
|
||
func (*ValidationMessage) Descriptor() ([]byte, []int) {
|
||
return file_node_proto_rawDescGZIP(), []int{19}
|
||
}
|
||
|
||
func (x *ValidationMessage) GetValidation() []byte {
|
||
if x != nil {
|
||
return x.Validation
|
||
}
|
||
return nil
|
||
}
|
||
|
||
type SyncRequest struct {
|
||
state protoimpl.MessageState
|
||
sizeCache protoimpl.SizeCache
|
||
unknownFields protoimpl.UnknownFields
|
||
|
||
FramesRequest *ClockFramesRequest `protobuf:"bytes,1,opt,name=frames_request,json=framesRequest,proto3" json:"frames_request,omitempty"`
|
||
}
|
||
|
||
func (x *SyncRequest) Reset() {
|
||
*x = SyncRequest{}
|
||
if protoimpl.UnsafeEnabled {
|
||
mi := &file_node_proto_msgTypes[20]
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
}
|
||
|
||
func (x *SyncRequest) String() string {
|
||
return protoimpl.X.MessageStringOf(x)
|
||
}
|
||
|
||
func (*SyncRequest) ProtoMessage() {}
|
||
|
||
func (x *SyncRequest) ProtoReflect() protoreflect.Message {
|
||
mi := &file_node_proto_msgTypes[20]
|
||
if protoimpl.UnsafeEnabled && x != nil {
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
if ms.LoadMessageInfo() == nil {
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
return ms
|
||
}
|
||
return mi.MessageOf(x)
|
||
}
|
||
|
||
// Deprecated: Use SyncRequest.ProtoReflect.Descriptor instead.
|
||
func (*SyncRequest) Descriptor() ([]byte, []int) {
|
||
return file_node_proto_rawDescGZIP(), []int{20}
|
||
}
|
||
|
||
func (x *SyncRequest) GetFramesRequest() *ClockFramesRequest {
|
||
if x != nil {
|
||
return x.FramesRequest
|
||
}
|
||
return nil
|
||
}
|
||
|
||
type SyncResponse struct {
|
||
state protoimpl.MessageState
|
||
sizeCache protoimpl.SizeCache
|
||
unknownFields protoimpl.UnknownFields
|
||
|
||
FramesResponse *ClockFramesResponse `protobuf:"bytes,1,opt,name=frames_response,json=framesResponse,proto3" json:"frames_response,omitempty"`
|
||
}
|
||
|
||
func (x *SyncResponse) Reset() {
|
||
*x = SyncResponse{}
|
||
if protoimpl.UnsafeEnabled {
|
||
mi := &file_node_proto_msgTypes[21]
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
}
|
||
|
||
func (x *SyncResponse) String() string {
|
||
return protoimpl.X.MessageStringOf(x)
|
||
}
|
||
|
||
func (*SyncResponse) ProtoMessage() {}
|
||
|
||
func (x *SyncResponse) ProtoReflect() protoreflect.Message {
|
||
mi := &file_node_proto_msgTypes[21]
|
||
if protoimpl.UnsafeEnabled && x != nil {
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
if ms.LoadMessageInfo() == nil {
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
return ms
|
||
}
|
||
return mi.MessageOf(x)
|
||
}
|
||
|
||
// Deprecated: Use SyncResponse.ProtoReflect.Descriptor instead.
|
||
func (*SyncResponse) Descriptor() ([]byte, []int) {
|
||
return file_node_proto_rawDescGZIP(), []int{21}
|
||
}
|
||
|
||
func (x *SyncResponse) GetFramesResponse() *ClockFramesResponse {
|
||
if x != nil {
|
||
return x.FramesResponse
|
||
}
|
||
return nil
|
||
}
|
||
|
||
type GetPeerManifestsRequest struct {
|
||
state protoimpl.MessageState
|
||
sizeCache protoimpl.SizeCache
|
||
unknownFields protoimpl.UnknownFields
|
||
}
|
||
|
||
func (x *GetPeerManifestsRequest) Reset() {
|
||
*x = GetPeerManifestsRequest{}
|
||
if protoimpl.UnsafeEnabled {
|
||
mi := &file_node_proto_msgTypes[22]
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
}
|
||
|
||
func (x *GetPeerManifestsRequest) String() string {
|
||
return protoimpl.X.MessageStringOf(x)
|
||
}
|
||
|
||
func (*GetPeerManifestsRequest) ProtoMessage() {}
|
||
|
||
func (x *GetPeerManifestsRequest) ProtoReflect() protoreflect.Message {
|
||
mi := &file_node_proto_msgTypes[22]
|
||
if protoimpl.UnsafeEnabled && x != nil {
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
if ms.LoadMessageInfo() == nil {
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
return ms
|
||
}
|
||
return mi.MessageOf(x)
|
||
}
|
||
|
||
// Deprecated: Use GetPeerManifestsRequest.ProtoReflect.Descriptor instead.
|
||
func (*GetPeerManifestsRequest) Descriptor() ([]byte, []int) {
|
||
return file_node_proto_rawDescGZIP(), []int{22}
|
||
}
|
||
|
||
type PeerManifest struct {
|
||
state protoimpl.MessageState
|
||
sizeCache protoimpl.SizeCache
|
||
unknownFields protoimpl.UnknownFields
|
||
|
||
PeerId []byte `protobuf:"bytes,1,opt,name=peer_id,json=peerId,proto3" json:"peer_id,omitempty"`
|
||
// The difficulty the self test was conducted under
|
||
Difficulty uint32 `protobuf:"varint,2,opt,name=difficulty,proto3" json:"difficulty,omitempty"`
|
||
// The resulting local time of computing the VDF test under the difficulty
|
||
DifficultyMetric int64 `protobuf:"varint,3,opt,name=difficulty_metric,json=difficultyMetric,proto3" json:"difficulty_metric,omitempty"`
|
||
// The resulting local time of computing a KZG commitment for a 16 degree
|
||
// polynomial
|
||
Commit_16Metric int64 `protobuf:"varint,4,opt,name=commit_16_metric,json=commit16Metric,proto3" json:"commit_16_metric,omitempty"`
|
||
// The resulting local time of computing a KZG commitment for a 128 degree
|
||
// polynomial
|
||
Commit_128Metric int64 `protobuf:"varint,5,opt,name=commit_128_metric,json=commit128Metric,proto3" json:"commit_128_metric,omitempty"`
|
||
// The resulting local time of computing a KZG commitment for a 1024 degree
|
||
// polynomial
|
||
Commit_1024Metric int64 `protobuf:"varint,6,opt,name=commit_1024_metric,json=commit1024Metric,proto3" json:"commit_1024_metric,omitempty"`
|
||
// The resulting local time of computing a KZG commitment for a 65536 degree
|
||
// polynomial
|
||
Commit_65536Metric int64 `protobuf:"varint,7,opt,name=commit_65536_metric,json=commit65536Metric,proto3" json:"commit_65536_metric,omitempty"`
|
||
// The resulting local time of computing a KZG proof for a 16 degree
|
||
// polynomial
|
||
Proof_16Metric int64 `protobuf:"varint,8,opt,name=proof_16_metric,json=proof16Metric,proto3" json:"proof_16_metric,omitempty"`
|
||
// The resulting local time of computing a KZG proof for a 128 degree
|
||
// polynomial
|
||
Proof_128Metric int64 `protobuf:"varint,9,opt,name=proof_128_metric,json=proof128Metric,proto3" json:"proof_128_metric,omitempty"`
|
||
// The resulting local time of computing a KZG proof for a 1024 degree
|
||
// polynomial
|
||
Proof_1024Metric int64 `protobuf:"varint,10,opt,name=proof_1024_metric,json=proof1024Metric,proto3" json:"proof_1024_metric,omitempty"`
|
||
// The resulting local time of computing a KZG proof for a 65536 degree
|
||
// polynomial
|
||
Proof_65536Metric int64 `protobuf:"varint,11,opt,name=proof_65536_metric,json=proof65536Metric,proto3" json:"proof_65536_metric,omitempty"`
|
||
// The number of reported accessible cores
|
||
Cores uint32 `protobuf:"varint,12,opt,name=cores,proto3" json:"cores,omitempty"`
|
||
// The total available memory
|
||
Memory []byte `protobuf:"bytes,13,opt,name=memory,proto3" json:"memory,omitempty"`
|
||
// The total available storage
|
||
Storage []byte `protobuf:"bytes,14,opt,name=storage,proto3" json:"storage,omitempty"`
|
||
// The list of supported capabilities
|
||
Capabilities []*Capability `protobuf:"bytes,15,rep,name=capabilities,proto3" json:"capabilities,omitempty"`
|
||
// The highest master frame the node has
|
||
MasterHeadFrame uint64 `protobuf:"varint,16,opt,name=master_head_frame,json=masterHeadFrame,proto3" json:"master_head_frame,omitempty"`
|
||
}
|
||
|
||
func (x *PeerManifest) Reset() {
|
||
*x = PeerManifest{}
|
||
if protoimpl.UnsafeEnabled {
|
||
mi := &file_node_proto_msgTypes[23]
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
}
|
||
|
||
func (x *PeerManifest) String() string {
|
||
return protoimpl.X.MessageStringOf(x)
|
||
}
|
||
|
||
func (*PeerManifest) ProtoMessage() {}
|
||
|
||
func (x *PeerManifest) ProtoReflect() protoreflect.Message {
|
||
mi := &file_node_proto_msgTypes[23]
|
||
if protoimpl.UnsafeEnabled && x != nil {
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
if ms.LoadMessageInfo() == nil {
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
return ms
|
||
}
|
||
return mi.MessageOf(x)
|
||
}
|
||
|
||
// Deprecated: Use PeerManifest.ProtoReflect.Descriptor instead.
|
||
func (*PeerManifest) Descriptor() ([]byte, []int) {
|
||
return file_node_proto_rawDescGZIP(), []int{23}
|
||
}
|
||
|
||
func (x *PeerManifest) GetPeerId() []byte {
|
||
if x != nil {
|
||
return x.PeerId
|
||
}
|
||
return nil
|
||
}
|
||
|
||
func (x *PeerManifest) GetDifficulty() uint32 {
|
||
if x != nil {
|
||
return x.Difficulty
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *PeerManifest) GetDifficultyMetric() int64 {
|
||
if x != nil {
|
||
return x.DifficultyMetric
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *PeerManifest) GetCommit_16Metric() int64 {
|
||
if x != nil {
|
||
return x.Commit_16Metric
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *PeerManifest) GetCommit_128Metric() int64 {
|
||
if x != nil {
|
||
return x.Commit_128Metric
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *PeerManifest) GetCommit_1024Metric() int64 {
|
||
if x != nil {
|
||
return x.Commit_1024Metric
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *PeerManifest) GetCommit_65536Metric() int64 {
|
||
if x != nil {
|
||
return x.Commit_65536Metric
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *PeerManifest) GetProof_16Metric() int64 {
|
||
if x != nil {
|
||
return x.Proof_16Metric
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *PeerManifest) GetProof_128Metric() int64 {
|
||
if x != nil {
|
||
return x.Proof_128Metric
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *PeerManifest) GetProof_1024Metric() int64 {
|
||
if x != nil {
|
||
return x.Proof_1024Metric
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *PeerManifest) GetProof_65536Metric() int64 {
|
||
if x != nil {
|
||
return x.Proof_65536Metric
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *PeerManifest) GetCores() uint32 {
|
||
if x != nil {
|
||
return x.Cores
|
||
}
|
||
return 0
|
||
}
|
||
|
||
func (x *PeerManifest) GetMemory() []byte {
|
||
if x != nil {
|
||
return x.Memory
|
||
}
|
||
return nil
|
||
}
|
||
|
||
func (x *PeerManifest) GetStorage() []byte {
|
||
if x != nil {
|
||
return x.Storage
|
||
}
|
||
return nil
|
||
}
|
||
|
||
func (x *PeerManifest) GetCapabilities() []*Capability {
|
||
if x != nil {
|
||
return x.Capabilities
|
||
}
|
||
return nil
|
||
}
|
||
|
||
func (x *PeerManifest) GetMasterHeadFrame() uint64 {
|
||
if x != nil {
|
||
return x.MasterHeadFrame
|
||
}
|
||
return 0
|
||
}
|
||
|
||
type PeerManifestsResponse struct {
|
||
state protoimpl.MessageState
|
||
sizeCache protoimpl.SizeCache
|
||
unknownFields protoimpl.UnknownFields
|
||
|
||
PeerManifests []*PeerManifest `protobuf:"bytes,1,rep,name=peer_manifests,json=peerManifests,proto3" json:"peer_manifests,omitempty"`
|
||
}
|
||
|
||
func (x *PeerManifestsResponse) Reset() {
|
||
*x = PeerManifestsResponse{}
|
||
if protoimpl.UnsafeEnabled {
|
||
mi := &file_node_proto_msgTypes[24]
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
}
|
||
|
||
func (x *PeerManifestsResponse) String() string {
|
||
return protoimpl.X.MessageStringOf(x)
|
||
}
|
||
|
||
func (*PeerManifestsResponse) ProtoMessage() {}
|
||
|
||
func (x *PeerManifestsResponse) ProtoReflect() protoreflect.Message {
|
||
mi := &file_node_proto_msgTypes[24]
|
||
if protoimpl.UnsafeEnabled && x != nil {
|
||
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
||
if ms.LoadMessageInfo() == nil {
|
||
ms.StoreMessageInfo(mi)
|
||
}
|
||
return ms
|
||
}
|
||
return mi.MessageOf(x)
|
||
}
|
||
|
||
// Deprecated: Use PeerManifestsResponse.ProtoReflect.Descriptor instead.
|
||
func (*PeerManifestsResponse) Descriptor() ([]byte, []int) {
|
||
return file_node_proto_rawDescGZIP(), []int{24}
|
||
}
|
||
|
||
func (x *PeerManifestsResponse) GetPeerManifests() []*PeerManifest {
|
||
if x != nil {
|
||
return x.PeerManifests
|
||
}
|
||
return nil
|
||
}
|
||
|
||
var File_node_proto protoreflect.FileDescriptor
|
||
|
||
var file_node_proto_rawDesc = []byte{
|
||
0x0a, 0x0a, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x12, 0x17, 0x71, 0x75,
|
||
0x69, 0x6c, 0x69, 0x62, 0x72, 0x69, 0x75, 0x6d, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x6e, 0x6f,
|
||
0x64, 0x65, 0x2e, 0x70, 0x62, 0x1a, 0x0b, 0x63, 0x6c, 0x6f, 0x63, 0x6b, 0x2e, 0x70, 0x72, 0x6f,
|
||
0x74, 0x6f, 0x22, 0xad, 0x01, 0x0a, 0x10, 0x47, 0x65, 0x74, 0x46, 0x72, 0x61, 0x6d, 0x65, 0x73,
|
||
0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x12, 0x16, 0x0a, 0x06, 0x66, 0x69, 0x6c, 0x74, 0x65,
|
||
0x72, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0c, 0x52, 0x06, 0x66, 0x69, 0x6c, 0x74, 0x65, 0x72, 0x12,
|
||
0x2a, 0x0a, 0x11, 0x66, 0x72, 0x6f, 0x6d, 0x5f, 0x66, 0x72, 0x61, 0x6d, 0x65, 0x5f, 0x6e, 0x75,
|
||
0x6d, 0x62, 0x65, 0x72, 0x18, 0x02, 0x20, 0x01, 0x28, 0x04, 0x52, 0x0f, 0x66, 0x72, 0x6f, 0x6d,
|
||
0x46, 0x72, 0x61, 0x6d, 0x65, 0x4e, 0x75, 0x6d, 0x62, 0x65, 0x72, 0x12, 0x26, 0x0a, 0x0f, 0x74,
|
||
0x6f, 0x5f, 0x66, 0x72, 0x61, 0x6d, 0x65, 0x5f, 0x6e, 0x75, 0x6d, 0x62, 0x65, 0x72, 0x18, 0x03,
|
||
0x20, 0x01, 0x28, 0x04, 0x52, 0x0d, 0x74, 0x6f, 0x46, 0x72, 0x61, 0x6d, 0x65, 0x4e, 0x75, 0x6d,
|
||
0x62, 0x65, 0x72, 0x12, 0x2d, 0x0a, 0x12, 0x69, 0x6e, 0x63, 0x6c, 0x75, 0x64, 0x65, 0x5f, 0x63,
|
||
0x61, 0x6e, 0x64, 0x69, 0x64, 0x61, 0x74, 0x65, 0x73, 0x18, 0x04, 0x20, 0x01, 0x28, 0x08, 0x52,
|
||
0x11, 0x69, 0x6e, 0x63, 0x6c, 0x75, 0x64, 0x65, 0x43, 0x61, 0x6e, 0x64, 0x69, 0x64, 0x61, 0x74,
|
||
0x65, 0x73, 0x22, 0x6c, 0x0a, 0x13, 0x47, 0x65, 0x74, 0x46, 0x72, 0x61, 0x6d, 0x65, 0x49, 0x6e,
|
||
0x66, 0x6f, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x12, 0x16, 0x0a, 0x06, 0x66, 0x69, 0x6c,
|
||
0x74, 0x65, 0x72, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0c, 0x52, 0x06, 0x66, 0x69, 0x6c, 0x74, 0x65,
|
||
0x72, 0x12, 0x21, 0x0a, 0x0c, 0x66, 0x72, 0x61, 0x6d, 0x65, 0x5f, 0x6e, 0x75, 0x6d, 0x62, 0x65,
|
||
0x72, 0x18, 0x02, 0x20, 0x01, 0x28, 0x04, 0x52, 0x0b, 0x66, 0x72, 0x61, 0x6d, 0x65, 0x4e, 0x75,
|
||
0x6d, 0x62, 0x65, 0x72, 0x12, 0x1a, 0x0a, 0x08, 0x73, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x6f, 0x72,
|
||
0x18, 0x03, 0x20, 0x01, 0x28, 0x0c, 0x52, 0x08, 0x73, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x6f, 0x72,
|
||
0x22, 0x14, 0x0a, 0x12, 0x47, 0x65, 0x74, 0x50, 0x65, 0x65, 0x72, 0x49, 0x6e, 0x66, 0x6f, 0x52,
|
||
0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x22, 0x14, 0x0a, 0x12, 0x47, 0x65, 0x74, 0x4e, 0x6f, 0x64,
|
||
0x65, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x22, 0x17, 0x0a, 0x15,
|
||
0x47, 0x65, 0x74, 0x4e, 0x65, 0x74, 0x77, 0x6f, 0x72, 0x6b, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65,
|
||
0x71, 0x75, 0x65, 0x73, 0x74, 0x22, 0x6c, 0x0a, 0x0e, 0x46, 0x72, 0x61, 0x6d, 0x65, 0x73, 0x52,
|
||
0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x5a, 0x0a, 0x16, 0x74, 0x72, 0x75, 0x6e, 0x63,
|
||
0x61, 0x74, 0x65, 0x64, 0x5f, 0x63, 0x6c, 0x6f, 0x63, 0x6b, 0x5f, 0x66, 0x72, 0x61, 0x6d, 0x65,
|
||
0x73, 0x18, 0x01, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x24, 0x2e, 0x71, 0x75, 0x69, 0x6c, 0x69, 0x62,
|
||
0x72, 0x69, 0x75, 0x6d, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x63, 0x6c, 0x6f, 0x63, 0x6b, 0x2e,
|
||
0x70, 0x62, 0x2e, 0x43, 0x6c, 0x6f, 0x63, 0x6b, 0x46, 0x72, 0x61, 0x6d, 0x65, 0x52, 0x14, 0x74,
|
||
0x72, 0x75, 0x6e, 0x63, 0x61, 0x74, 0x65, 0x64, 0x43, 0x6c, 0x6f, 0x63, 0x6b, 0x46, 0x72, 0x61,
|
||
0x6d, 0x65, 0x73, 0x22, 0x5a, 0x0a, 0x11, 0x46, 0x72, 0x61, 0x6d, 0x65, 0x49, 0x6e, 0x66, 0x6f,
|
||
0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x45, 0x0a, 0x0b, 0x63, 0x6c, 0x6f, 0x63,
|
||
0x6b, 0x5f, 0x66, 0x72, 0x61, 0x6d, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x24, 0x2e,
|
||
0x71, 0x75, 0x69, 0x6c, 0x69, 0x62, 0x72, 0x69, 0x75, 0x6d, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e,
|
||
0x63, 0x6c, 0x6f, 0x63, 0x6b, 0x2e, 0x70, 0x62, 0x2e, 0x43, 0x6c, 0x6f, 0x63, 0x6b, 0x46, 0x72,
|
||
0x61, 0x6d, 0x65, 0x52, 0x0a, 0x63, 0x6c, 0x6f, 0x63, 0x6b, 0x46, 0x72, 0x61, 0x6d, 0x65, 0x22,
|
||
0xfc, 0x01, 0x0a, 0x08, 0x50, 0x65, 0x65, 0x72, 0x49, 0x6e, 0x66, 0x6f, 0x12, 0x17, 0x0a, 0x07,
|
||
0x70, 0x65, 0x65, 0x72, 0x5f, 0x69, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0c, 0x52, 0x06, 0x70,
|
||
0x65, 0x65, 0x72, 0x49, 0x64, 0x12, 0x1e, 0x0a, 0x0a, 0x6d, 0x75, 0x6c, 0x74, 0x69, 0x61, 0x64,
|
||
0x64, 0x72, 0x73, 0x18, 0x02, 0x20, 0x03, 0x28, 0x09, 0x52, 0x0a, 0x6d, 0x75, 0x6c, 0x74, 0x69,
|
||
0x61, 0x64, 0x64, 0x72, 0x73, 0x12, 0x1b, 0x0a, 0x09, 0x6d, 0x61, 0x78, 0x5f, 0x66, 0x72, 0x61,
|
||
0x6d, 0x65, 0x18, 0x03, 0x20, 0x01, 0x28, 0x04, 0x52, 0x08, 0x6d, 0x61, 0x78, 0x46, 0x72, 0x61,
|
||
0x6d, 0x65, 0x12, 0x1c, 0x0a, 0x09, 0x74, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x18,
|
||
0x04, 0x20, 0x01, 0x28, 0x03, 0x52, 0x09, 0x74, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70,
|
||
0x12, 0x18, 0x0a, 0x07, 0x76, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x18, 0x05, 0x20, 0x01, 0x28,
|
||
0x0c, 0x52, 0x07, 0x76, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x12, 0x1c, 0x0a, 0x09, 0x73, 0x69,
|
||
0x67, 0x6e, 0x61, 0x74, 0x75, 0x72, 0x65, 0x18, 0x06, 0x20, 0x01, 0x28, 0x0c, 0x52, 0x09, 0x73,
|
||
0x69, 0x67, 0x6e, 0x61, 0x74, 0x75, 0x72, 0x65, 0x12, 0x1d, 0x0a, 0x0a, 0x70, 0x75, 0x62, 0x6c,
|
||
0x69, 0x63, 0x5f, 0x6b, 0x65, 0x79, 0x18, 0x07, 0x20, 0x01, 0x28, 0x0c, 0x52, 0x09, 0x70, 0x75,
|
||
0x62, 0x6c, 0x69, 0x63, 0x4b, 0x65, 0x79, 0x12, 0x25, 0x0a, 0x0e, 0x74, 0x6f, 0x74, 0x61, 0x6c,
|
||
0x5f, 0x64, 0x69, 0x73, 0x74, 0x61, 0x6e, 0x63, 0x65, 0x18, 0x08, 0x20, 0x01, 0x28, 0x0c, 0x52,
|
||
0x0d, 0x74, 0x6f, 0x74, 0x61, 0x6c, 0x44, 0x69, 0x73, 0x74, 0x61, 0x6e, 0x63, 0x65, 0x22, 0xad,
|
||
0x01, 0x0a, 0x10, 0x50, 0x65, 0x65, 0x72, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x73, 0x70, 0x6f,
|
||
0x6e, 0x73, 0x65, 0x12, 0x3e, 0x0a, 0x09, 0x70, 0x65, 0x65, 0x72, 0x5f, 0x69, 0x6e, 0x66, 0x6f,
|
||
0x18, 0x01, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x21, 0x2e, 0x71, 0x75, 0x69, 0x6c, 0x69, 0x62, 0x72,
|
||
0x69, 0x75, 0x6d, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x70, 0x62,
|
||
0x2e, 0x50, 0x65, 0x65, 0x72, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x08, 0x70, 0x65, 0x65, 0x72, 0x49,
|
||
0x6e, 0x66, 0x6f, 0x12, 0x59, 0x0a, 0x17, 0x75, 0x6e, 0x63, 0x6f, 0x6f, 0x70, 0x65, 0x72, 0x61,
|
||
0x74, 0x69, 0x76, 0x65, 0x5f, 0x70, 0x65, 0x65, 0x72, 0x5f, 0x69, 0x6e, 0x66, 0x6f, 0x18, 0x02,
|
||
0x20, 0x03, 0x28, 0x0b, 0x32, 0x21, 0x2e, 0x71, 0x75, 0x69, 0x6c, 0x69, 0x62, 0x72, 0x69, 0x75,
|
||
0x6d, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x70, 0x62, 0x2e, 0x50,
|
||
0x65, 0x65, 0x72, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x15, 0x75, 0x6e, 0x63, 0x6f, 0x6f, 0x70, 0x65,
|
||
0x72, 0x61, 0x74, 0x69, 0x76, 0x65, 0x50, 0x65, 0x65, 0x72, 0x49, 0x6e, 0x66, 0x6f, 0x22, 0x65,
|
||
0x0a, 0x0b, 0x4e, 0x65, 0x74, 0x77, 0x6f, 0x72, 0x6b, 0x49, 0x6e, 0x66, 0x6f, 0x12, 0x17, 0x0a,
|
||
0x07, 0x70, 0x65, 0x65, 0x72, 0x5f, 0x69, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0c, 0x52, 0x06,
|
||
0x70, 0x65, 0x65, 0x72, 0x49, 0x64, 0x12, 0x1e, 0x0a, 0x0a, 0x6d, 0x75, 0x6c, 0x74, 0x69, 0x61,
|
||
0x64, 0x64, 0x72, 0x73, 0x18, 0x02, 0x20, 0x03, 0x28, 0x09, 0x52, 0x0a, 0x6d, 0x75, 0x6c, 0x74,
|
||
0x69, 0x61, 0x64, 0x64, 0x72, 0x73, 0x12, 0x1d, 0x0a, 0x0a, 0x70, 0x65, 0x65, 0x72, 0x5f, 0x73,
|
||
0x63, 0x6f, 0x72, 0x65, 0x18, 0x03, 0x20, 0x01, 0x28, 0x01, 0x52, 0x09, 0x70, 0x65, 0x65, 0x72,
|
||
0x53, 0x63, 0x6f, 0x72, 0x65, 0x22, 0x81, 0x01, 0x0a, 0x10, 0x4e, 0x6f, 0x64, 0x65, 0x49, 0x6e,
|
||
0x66, 0x6f, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x17, 0x0a, 0x07, 0x70, 0x65,
|
||
0x65, 0x72, 0x5f, 0x69, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x06, 0x70, 0x65, 0x65,
|
||
0x72, 0x49, 0x64, 0x12, 0x1b, 0x0a, 0x09, 0x6d, 0x61, 0x78, 0x5f, 0x66, 0x72, 0x61, 0x6d, 0x65,
|
||
0x18, 0x02, 0x20, 0x01, 0x28, 0x04, 0x52, 0x08, 0x6d, 0x61, 0x78, 0x46, 0x72, 0x61, 0x6d, 0x65,
|
||
0x12, 0x1d, 0x0a, 0x0a, 0x70, 0x65, 0x65, 0x72, 0x5f, 0x73, 0x63, 0x6f, 0x72, 0x65, 0x18, 0x03,
|
||
0x20, 0x01, 0x28, 0x04, 0x52, 0x09, 0x70, 0x65, 0x65, 0x72, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x12,
|
||
0x18, 0x0a, 0x07, 0x76, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x18, 0x04, 0x20, 0x01, 0x28, 0x0c,
|
||
0x52, 0x07, 0x76, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x22, 0xaf, 0x01, 0x0a, 0x12, 0x50, 0x75,
|
||
0x74, 0x50, 0x65, 0x65, 0x72, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74,
|
||
0x12, 0x3e, 0x0a, 0x09, 0x70, 0x65, 0x65, 0x72, 0x5f, 0x69, 0x6e, 0x66, 0x6f, 0x18, 0x01, 0x20,
|
||
0x03, 0x28, 0x0b, 0x32, 0x21, 0x2e, 0x71, 0x75, 0x69, 0x6c, 0x69, 0x62, 0x72, 0x69, 0x75, 0x6d,
|
||
0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x70, 0x62, 0x2e, 0x50, 0x65,
|
||
0x65, 0x72, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x08, 0x70, 0x65, 0x65, 0x72, 0x49, 0x6e, 0x66, 0x6f,
|
||
0x12, 0x59, 0x0a, 0x17, 0x75, 0x6e, 0x63, 0x6f, 0x6f, 0x70, 0x65, 0x72, 0x61, 0x74, 0x69, 0x76,
|
||
0x65, 0x5f, 0x70, 0x65, 0x65, 0x72, 0x5f, 0x69, 0x6e, 0x66, 0x6f, 0x18, 0x02, 0x20, 0x03, 0x28,
|
||
0x0b, 0x32, 0x21, 0x2e, 0x71, 0x75, 0x69, 0x6c, 0x69, 0x62, 0x72, 0x69, 0x75, 0x6d, 0x2e, 0x6e,
|
||
0x6f, 0x64, 0x65, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x70, 0x62, 0x2e, 0x50, 0x65, 0x65, 0x72,
|
||
0x49, 0x6e, 0x66, 0x6f, 0x52, 0x15, 0x75, 0x6e, 0x63, 0x6f, 0x6f, 0x70, 0x65, 0x72, 0x61, 0x74,
|
||
0x69, 0x76, 0x65, 0x50, 0x65, 0x65, 0x72, 0x49, 0x6e, 0x66, 0x6f, 0x22, 0x87, 0x01, 0x0a, 0x12,
|
||
0x50, 0x75, 0x74, 0x4e, 0x6f, 0x64, 0x65, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x71, 0x75, 0x65,
|
||
0x73, 0x74, 0x12, 0x17, 0x0a, 0x07, 0x70, 0x65, 0x65, 0x72, 0x5f, 0x69, 0x64, 0x18, 0x01, 0x20,
|
||
0x01, 0x28, 0x09, 0x52, 0x06, 0x70, 0x65, 0x65, 0x72, 0x49, 0x64, 0x12, 0x1b, 0x0a, 0x09, 0x6d,
|
||
0x61, 0x78, 0x5f, 0x66, 0x72, 0x61, 0x6d, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x04, 0x52, 0x08,
|
||
0x6d, 0x61, 0x78, 0x46, 0x72, 0x61, 0x6d, 0x65, 0x12, 0x1d, 0x0a, 0x0a, 0x70, 0x65, 0x65, 0x72,
|
||
0x5f, 0x73, 0x63, 0x6f, 0x72, 0x65, 0x18, 0x03, 0x20, 0x01, 0x28, 0x04, 0x52, 0x09, 0x70, 0x65,
|
||
0x65, 0x72, 0x53, 0x63, 0x6f, 0x72, 0x65, 0x12, 0x1c, 0x0a, 0x09, 0x73, 0x69, 0x67, 0x6e, 0x61,
|
||
0x74, 0x75, 0x72, 0x65, 0x18, 0x04, 0x20, 0x01, 0x28, 0x0c, 0x52, 0x09, 0x73, 0x69, 0x67, 0x6e,
|
||
0x61, 0x74, 0x75, 0x72, 0x65, 0x22, 0x0d, 0x0a, 0x0b, 0x50, 0x75, 0x74, 0x52, 0x65, 0x73, 0x70,
|
||
0x6f, 0x6e, 0x73, 0x65, 0x22, 0x5e, 0x0a, 0x13, 0x4e, 0x65, 0x74, 0x77, 0x6f, 0x72, 0x6b, 0x49,
|
||
0x6e, 0x66, 0x6f, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x47, 0x0a, 0x0c, 0x6e,
|
||
0x65, 0x74, 0x77, 0x6f, 0x72, 0x6b, 0x5f, 0x69, 0x6e, 0x66, 0x6f, 0x18, 0x01, 0x20, 0x03, 0x28,
|
||
0x0b, 0x32, 0x24, 0x2e, 0x71, 0x75, 0x69, 0x6c, 0x69, 0x62, 0x72, 0x69, 0x75, 0x6d, 0x2e, 0x6e,
|
||
0x6f, 0x64, 0x65, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x70, 0x62, 0x2e, 0x4e, 0x65, 0x74, 0x77,
|
||
0x6f, 0x72, 0x6b, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x0b, 0x6e, 0x65, 0x74, 0x77, 0x6f, 0x72, 0x6b,
|
||
0x49, 0x6e, 0x66, 0x6f, 0x22, 0x15, 0x0a, 0x13, 0x47, 0x65, 0x74, 0x54, 0x6f, 0x6b, 0x65, 0x6e,
|
||
0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x22, 0xe0, 0x01, 0x0a, 0x11,
|
||
0x54, 0x6f, 0x6b, 0x65, 0x6e, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73,
|
||
0x65, 0x12, 0x34, 0x0a, 0x16, 0x63, 0x6f, 0x6e, 0x66, 0x69, 0x72, 0x6d, 0x65, 0x64, 0x5f, 0x74,
|
||
0x6f, 0x6b, 0x65, 0x6e, 0x5f, 0x73, 0x75, 0x70, 0x70, 0x6c, 0x79, 0x18, 0x01, 0x20, 0x01, 0x28,
|
||
0x0c, 0x52, 0x14, 0x63, 0x6f, 0x6e, 0x66, 0x69, 0x72, 0x6d, 0x65, 0x64, 0x54, 0x6f, 0x6b, 0x65,
|
||
0x6e, 0x53, 0x75, 0x70, 0x70, 0x6c, 0x79, 0x12, 0x38, 0x0a, 0x18, 0x75, 0x6e, 0x63, 0x6f, 0x6e,
|
||
0x66, 0x69, 0x72, 0x6d, 0x65, 0x64, 0x5f, 0x74, 0x6f, 0x6b, 0x65, 0x6e, 0x5f, 0x73, 0x75, 0x70,
|
||
0x70, 0x6c, 0x79, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0c, 0x52, 0x16, 0x75, 0x6e, 0x63, 0x6f, 0x6e,
|
||
0x66, 0x69, 0x72, 0x6d, 0x65, 0x64, 0x54, 0x6f, 0x6b, 0x65, 0x6e, 0x53, 0x75, 0x70, 0x70, 0x6c,
|
||
0x79, 0x12, 0x21, 0x0a, 0x0c, 0x6f, 0x77, 0x6e, 0x65, 0x64, 0x5f, 0x74, 0x6f, 0x6b, 0x65, 0x6e,
|
||
0x73, 0x18, 0x03, 0x20, 0x01, 0x28, 0x0c, 0x52, 0x0b, 0x6f, 0x77, 0x6e, 0x65, 0x64, 0x54, 0x6f,
|
||
0x6b, 0x65, 0x6e, 0x73, 0x12, 0x38, 0x0a, 0x18, 0x75, 0x6e, 0x63, 0x6f, 0x6e, 0x66, 0x69, 0x72,
|
||
0x6d, 0x65, 0x64, 0x5f, 0x6f, 0x77, 0x6e, 0x65, 0x64, 0x5f, 0x74, 0x6f, 0x6b, 0x65, 0x6e, 0x73,
|
||
0x18, 0x04, 0x20, 0x01, 0x28, 0x0c, 0x52, 0x16, 0x75, 0x6e, 0x63, 0x6f, 0x6e, 0x66, 0x69, 0x72,
|
||
0x6d, 0x65, 0x64, 0x4f, 0x77, 0x6e, 0x65, 0x64, 0x54, 0x6f, 0x6b, 0x65, 0x6e, 0x73, 0x22, 0x6e,
|
||
0x0a, 0x0a, 0x43, 0x61, 0x70, 0x61, 0x62, 0x69, 0x6c, 0x69, 0x74, 0x79, 0x12, 0x2f, 0x0a, 0x13,
|
||
0x70, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x5f, 0x69, 0x64, 0x65, 0x6e, 0x74, 0x69, 0x66,
|
||
0x69, 0x65, 0x72, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0d, 0x52, 0x12, 0x70, 0x72, 0x6f, 0x74, 0x6f,
|
||
0x63, 0x6f, 0x6c, 0x49, 0x64, 0x65, 0x6e, 0x74, 0x69, 0x66, 0x69, 0x65, 0x72, 0x12, 0x2f, 0x0a,
|
||
0x13, 0x61, 0x64, 0x64, 0x69, 0x74, 0x69, 0x6f, 0x6e, 0x61, 0x6c, 0x5f, 0x6d, 0x65, 0x74, 0x61,
|
||
0x64, 0x61, 0x74, 0x61, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0c, 0x52, 0x12, 0x61, 0x64, 0x64, 0x69,
|
||
0x74, 0x69, 0x6f, 0x6e, 0x61, 0x6c, 0x4d, 0x65, 0x74, 0x61, 0x64, 0x61, 0x74, 0x61, 0x22, 0xfa,
|
||
0x04, 0x0a, 0x0e, 0x53, 0x65, 0x6c, 0x66, 0x54, 0x65, 0x73, 0x74, 0x52, 0x65, 0x70, 0x6f, 0x72,
|
||
0x74, 0x12, 0x1e, 0x0a, 0x0a, 0x64, 0x69, 0x66, 0x66, 0x69, 0x63, 0x75, 0x6c, 0x74, 0x79, 0x18,
|
||
0x01, 0x20, 0x01, 0x28, 0x0d, 0x52, 0x0a, 0x64, 0x69, 0x66, 0x66, 0x69, 0x63, 0x75, 0x6c, 0x74,
|
||
0x79, 0x12, 0x2b, 0x0a, 0x11, 0x64, 0x69, 0x66, 0x66, 0x69, 0x63, 0x75, 0x6c, 0x74, 0x79, 0x5f,
|
||
0x6d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x18, 0x02, 0x20, 0x01, 0x28, 0x03, 0x52, 0x10, 0x64, 0x69,
|
||
0x66, 0x66, 0x69, 0x63, 0x75, 0x6c, 0x74, 0x79, 0x4d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x12, 0x28,
|
||
0x0a, 0x10, 0x63, 0x6f, 0x6d, 0x6d, 0x69, 0x74, 0x5f, 0x31, 0x36, 0x5f, 0x6d, 0x65, 0x74, 0x72,
|
||
0x69, 0x63, 0x18, 0x03, 0x20, 0x01, 0x28, 0x03, 0x52, 0x0e, 0x63, 0x6f, 0x6d, 0x6d, 0x69, 0x74,
|
||
0x31, 0x36, 0x4d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x12, 0x2a, 0x0a, 0x11, 0x63, 0x6f, 0x6d, 0x6d,
|
||
0x69, 0x74, 0x5f, 0x31, 0x32, 0x38, 0x5f, 0x6d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x18, 0x04, 0x20,
|
||
0x01, 0x28, 0x03, 0x52, 0x0f, 0x63, 0x6f, 0x6d, 0x6d, 0x69, 0x74, 0x31, 0x32, 0x38, 0x4d, 0x65,
|
||
0x74, 0x72, 0x69, 0x63, 0x12, 0x2c, 0x0a, 0x12, 0x63, 0x6f, 0x6d, 0x6d, 0x69, 0x74, 0x5f, 0x31,
|
||
0x30, 0x32, 0x34, 0x5f, 0x6d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x18, 0x05, 0x20, 0x01, 0x28, 0x03,
|
||
0x52, 0x10, 0x63, 0x6f, 0x6d, 0x6d, 0x69, 0x74, 0x31, 0x30, 0x32, 0x34, 0x4d, 0x65, 0x74, 0x72,
|
||
0x69, 0x63, 0x12, 0x2e, 0x0a, 0x13, 0x63, 0x6f, 0x6d, 0x6d, 0x69, 0x74, 0x5f, 0x36, 0x35, 0x35,
|
||
0x33, 0x36, 0x5f, 0x6d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x18, 0x06, 0x20, 0x01, 0x28, 0x03, 0x52,
|
||
0x11, 0x63, 0x6f, 0x6d, 0x6d, 0x69, 0x74, 0x36, 0x35, 0x35, 0x33, 0x36, 0x4d, 0x65, 0x74, 0x72,
|
||
0x69, 0x63, 0x12, 0x26, 0x0a, 0x0f, 0x70, 0x72, 0x6f, 0x6f, 0x66, 0x5f, 0x31, 0x36, 0x5f, 0x6d,
|
||
0x65, 0x74, 0x72, 0x69, 0x63, 0x18, 0x07, 0x20, 0x01, 0x28, 0x03, 0x52, 0x0d, 0x70, 0x72, 0x6f,
|
||
0x6f, 0x66, 0x31, 0x36, 0x4d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x12, 0x28, 0x0a, 0x10, 0x70, 0x72,
|
||
0x6f, 0x6f, 0x66, 0x5f, 0x31, 0x32, 0x38, 0x5f, 0x6d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x18, 0x08,
|
||
0x20, 0x01, 0x28, 0x03, 0x52, 0x0e, 0x70, 0x72, 0x6f, 0x6f, 0x66, 0x31, 0x32, 0x38, 0x4d, 0x65,
|
||
0x74, 0x72, 0x69, 0x63, 0x12, 0x2a, 0x0a, 0x11, 0x70, 0x72, 0x6f, 0x6f, 0x66, 0x5f, 0x31, 0x30,
|
||
0x32, 0x34, 0x5f, 0x6d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x18, 0x09, 0x20, 0x01, 0x28, 0x03, 0x52,
|
||
0x0f, 0x70, 0x72, 0x6f, 0x6f, 0x66, 0x31, 0x30, 0x32, 0x34, 0x4d, 0x65, 0x74, 0x72, 0x69, 0x63,
|
||
0x12, 0x2c, 0x0a, 0x12, 0x70, 0x72, 0x6f, 0x6f, 0x66, 0x5f, 0x36, 0x35, 0x35, 0x33, 0x36, 0x5f,
|
||
0x6d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x18, 0x0a, 0x20, 0x01, 0x28, 0x03, 0x52, 0x10, 0x70, 0x72,
|
||
0x6f, 0x6f, 0x66, 0x36, 0x35, 0x35, 0x33, 0x36, 0x4d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x12, 0x14,
|
||
0x0a, 0x05, 0x63, 0x6f, 0x72, 0x65, 0x73, 0x18, 0x0b, 0x20, 0x01, 0x28, 0x0d, 0x52, 0x05, 0x63,
|
||
0x6f, 0x72, 0x65, 0x73, 0x12, 0x16, 0x0a, 0x06, 0x6d, 0x65, 0x6d, 0x6f, 0x72, 0x79, 0x18, 0x0c,
|
||
0x20, 0x01, 0x28, 0x0c, 0x52, 0x06, 0x6d, 0x65, 0x6d, 0x6f, 0x72, 0x79, 0x12, 0x18, 0x0a, 0x07,
|
||
0x73, 0x74, 0x6f, 0x72, 0x61, 0x67, 0x65, 0x18, 0x0d, 0x20, 0x01, 0x28, 0x0c, 0x52, 0x07, 0x73,
|
||
0x74, 0x6f, 0x72, 0x61, 0x67, 0x65, 0x12, 0x47, 0x0a, 0x0c, 0x63, 0x61, 0x70, 0x61, 0x62, 0x69,
|
||
0x6c, 0x69, 0x74, 0x69, 0x65, 0x73, 0x18, 0x0e, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x23, 0x2e, 0x71,
|
||
0x75, 0x69, 0x6c, 0x69, 0x62, 0x72, 0x69, 0x75, 0x6d, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x6e,
|
||
0x6f, 0x64, 0x65, 0x2e, 0x70, 0x62, 0x2e, 0x43, 0x61, 0x70, 0x61, 0x62, 0x69, 0x6c, 0x69, 0x74,
|
||
0x79, 0x52, 0x0c, 0x63, 0x61, 0x70, 0x61, 0x62, 0x69, 0x6c, 0x69, 0x74, 0x69, 0x65, 0x73, 0x12,
|
||
0x2a, 0x0a, 0x11, 0x6d, 0x61, 0x73, 0x74, 0x65, 0x72, 0x5f, 0x68, 0x65, 0x61, 0x64, 0x5f, 0x66,
|
||
0x72, 0x61, 0x6d, 0x65, 0x18, 0x0f, 0x20, 0x01, 0x28, 0x04, 0x52, 0x0f, 0x6d, 0x61, 0x73, 0x74,
|
||
0x65, 0x72, 0x48, 0x65, 0x61, 0x64, 0x46, 0x72, 0x61, 0x6d, 0x65, 0x22, 0x33, 0x0a, 0x11, 0x56,
|
||
0x61, 0x6c, 0x69, 0x64, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65,
|
||
0x12, 0x1e, 0x0a, 0x0a, 0x76, 0x61, 0x6c, 0x69, 0x64, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x18, 0x01,
|
||
0x20, 0x01, 0x28, 0x0c, 0x52, 0x0a, 0x76, 0x61, 0x6c, 0x69, 0x64, 0x61, 0x74, 0x69, 0x6f, 0x6e,
|
||
0x22, 0x62, 0x0a, 0x0b, 0x53, 0x79, 0x6e, 0x63, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x12,
|
||
0x53, 0x0a, 0x0e, 0x66, 0x72, 0x61, 0x6d, 0x65, 0x73, 0x5f, 0x72, 0x65, 0x71, 0x75, 0x65, 0x73,
|
||
0x74, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x2c, 0x2e, 0x71, 0x75, 0x69, 0x6c, 0x69, 0x62,
|
||
0x72, 0x69, 0x75, 0x6d, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x63, 0x6c, 0x6f, 0x63, 0x6b, 0x2e,
|
||
0x70, 0x62, 0x2e, 0x43, 0x6c, 0x6f, 0x63, 0x6b, 0x46, 0x72, 0x61, 0x6d, 0x65, 0x73, 0x52, 0x65,
|
||
0x71, 0x75, 0x65, 0x73, 0x74, 0x52, 0x0d, 0x66, 0x72, 0x61, 0x6d, 0x65, 0x73, 0x52, 0x65, 0x71,
|
||
0x75, 0x65, 0x73, 0x74, 0x22, 0x66, 0x0a, 0x0c, 0x53, 0x79, 0x6e, 0x63, 0x52, 0x65, 0x73, 0x70,
|
||
0x6f, 0x6e, 0x73, 0x65, 0x12, 0x56, 0x0a, 0x0f, 0x66, 0x72, 0x61, 0x6d, 0x65, 0x73, 0x5f, 0x72,
|
||
0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x2d, 0x2e,
|
||
0x71, 0x75, 0x69, 0x6c, 0x69, 0x62, 0x72, 0x69, 0x75, 0x6d, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e,
|
||
0x63, 0x6c, 0x6f, 0x63, 0x6b, 0x2e, 0x70, 0x62, 0x2e, 0x43, 0x6c, 0x6f, 0x63, 0x6b, 0x46, 0x72,
|
||
0x61, 0x6d, 0x65, 0x73, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x52, 0x0e, 0x66, 0x72,
|
||
0x61, 0x6d, 0x65, 0x73, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x22, 0x19, 0x0a, 0x17,
|
||
0x47, 0x65, 0x74, 0x50, 0x65, 0x65, 0x72, 0x4d, 0x61, 0x6e, 0x69, 0x66, 0x65, 0x73, 0x74, 0x73,
|
||
0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x22, 0x91, 0x05, 0x0a, 0x0c, 0x50, 0x65, 0x65, 0x72,
|
||
0x4d, 0x61, 0x6e, 0x69, 0x66, 0x65, 0x73, 0x74, 0x12, 0x17, 0x0a, 0x07, 0x70, 0x65, 0x65, 0x72,
|
||
0x5f, 0x69, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0c, 0x52, 0x06, 0x70, 0x65, 0x65, 0x72, 0x49,
|
||
0x64, 0x12, 0x1e, 0x0a, 0x0a, 0x64, 0x69, 0x66, 0x66, 0x69, 0x63, 0x75, 0x6c, 0x74, 0x79, 0x18,
|
||
0x02, 0x20, 0x01, 0x28, 0x0d, 0x52, 0x0a, 0x64, 0x69, 0x66, 0x66, 0x69, 0x63, 0x75, 0x6c, 0x74,
|
||
0x79, 0x12, 0x2b, 0x0a, 0x11, 0x64, 0x69, 0x66, 0x66, 0x69, 0x63, 0x75, 0x6c, 0x74, 0x79, 0x5f,
|
||
0x6d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x18, 0x03, 0x20, 0x01, 0x28, 0x03, 0x52, 0x10, 0x64, 0x69,
|
||
0x66, 0x66, 0x69, 0x63, 0x75, 0x6c, 0x74, 0x79, 0x4d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x12, 0x28,
|
||
0x0a, 0x10, 0x63, 0x6f, 0x6d, 0x6d, 0x69, 0x74, 0x5f, 0x31, 0x36, 0x5f, 0x6d, 0x65, 0x74, 0x72,
|
||
0x69, 0x63, 0x18, 0x04, 0x20, 0x01, 0x28, 0x03, 0x52, 0x0e, 0x63, 0x6f, 0x6d, 0x6d, 0x69, 0x74,
|
||
0x31, 0x36, 0x4d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x12, 0x2a, 0x0a, 0x11, 0x63, 0x6f, 0x6d, 0x6d,
|
||
0x69, 0x74, 0x5f, 0x31, 0x32, 0x38, 0x5f, 0x6d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x18, 0x05, 0x20,
|
||
0x01, 0x28, 0x03, 0x52, 0x0f, 0x63, 0x6f, 0x6d, 0x6d, 0x69, 0x74, 0x31, 0x32, 0x38, 0x4d, 0x65,
|
||
0x74, 0x72, 0x69, 0x63, 0x12, 0x2c, 0x0a, 0x12, 0x63, 0x6f, 0x6d, 0x6d, 0x69, 0x74, 0x5f, 0x31,
|
||
0x30, 0x32, 0x34, 0x5f, 0x6d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x18, 0x06, 0x20, 0x01, 0x28, 0x03,
|
||
0x52, 0x10, 0x63, 0x6f, 0x6d, 0x6d, 0x69, 0x74, 0x31, 0x30, 0x32, 0x34, 0x4d, 0x65, 0x74, 0x72,
|
||
0x69, 0x63, 0x12, 0x2e, 0x0a, 0x13, 0x63, 0x6f, 0x6d, 0x6d, 0x69, 0x74, 0x5f, 0x36, 0x35, 0x35,
|
||
0x33, 0x36, 0x5f, 0x6d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x18, 0x07, 0x20, 0x01, 0x28, 0x03, 0x52,
|
||
0x11, 0x63, 0x6f, 0x6d, 0x6d, 0x69, 0x74, 0x36, 0x35, 0x35, 0x33, 0x36, 0x4d, 0x65, 0x74, 0x72,
|
||
0x69, 0x63, 0x12, 0x26, 0x0a, 0x0f, 0x70, 0x72, 0x6f, 0x6f, 0x66, 0x5f, 0x31, 0x36, 0x5f, 0x6d,
|
||
0x65, 0x74, 0x72, 0x69, 0x63, 0x18, 0x08, 0x20, 0x01, 0x28, 0x03, 0x52, 0x0d, 0x70, 0x72, 0x6f,
|
||
0x6f, 0x66, 0x31, 0x36, 0x4d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x12, 0x28, 0x0a, 0x10, 0x70, 0x72,
|
||
0x6f, 0x6f, 0x66, 0x5f, 0x31, 0x32, 0x38, 0x5f, 0x6d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x18, 0x09,
|
||
0x20, 0x01, 0x28, 0x03, 0x52, 0x0e, 0x70, 0x72, 0x6f, 0x6f, 0x66, 0x31, 0x32, 0x38, 0x4d, 0x65,
|
||
0x74, 0x72, 0x69, 0x63, 0x12, 0x2a, 0x0a, 0x11, 0x70, 0x72, 0x6f, 0x6f, 0x66, 0x5f, 0x31, 0x30,
|
||
0x32, 0x34, 0x5f, 0x6d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x18, 0x0a, 0x20, 0x01, 0x28, 0x03, 0x52,
|
||
0x0f, 0x70, 0x72, 0x6f, 0x6f, 0x66, 0x31, 0x30, 0x32, 0x34, 0x4d, 0x65, 0x74, 0x72, 0x69, 0x63,
|
||
0x12, 0x2c, 0x0a, 0x12, 0x70, 0x72, 0x6f, 0x6f, 0x66, 0x5f, 0x36, 0x35, 0x35, 0x33, 0x36, 0x5f,
|
||
0x6d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x18, 0x0b, 0x20, 0x01, 0x28, 0x03, 0x52, 0x10, 0x70, 0x72,
|
||
0x6f, 0x6f, 0x66, 0x36, 0x35, 0x35, 0x33, 0x36, 0x4d, 0x65, 0x74, 0x72, 0x69, 0x63, 0x12, 0x14,
|
||
0x0a, 0x05, 0x63, 0x6f, 0x72, 0x65, 0x73, 0x18, 0x0c, 0x20, 0x01, 0x28, 0x0d, 0x52, 0x05, 0x63,
|
||
0x6f, 0x72, 0x65, 0x73, 0x12, 0x16, 0x0a, 0x06, 0x6d, 0x65, 0x6d, 0x6f, 0x72, 0x79, 0x18, 0x0d,
|
||
0x20, 0x01, 0x28, 0x0c, 0x52, 0x06, 0x6d, 0x65, 0x6d, 0x6f, 0x72, 0x79, 0x12, 0x18, 0x0a, 0x07,
|
||
0x73, 0x74, 0x6f, 0x72, 0x61, 0x67, 0x65, 0x18, 0x0e, 0x20, 0x01, 0x28, 0x0c, 0x52, 0x07, 0x73,
|
||
0x74, 0x6f, 0x72, 0x61, 0x67, 0x65, 0x12, 0x47, 0x0a, 0x0c, 0x63, 0x61, 0x70, 0x61, 0x62, 0x69,
|
||
0x6c, 0x69, 0x74, 0x69, 0x65, 0x73, 0x18, 0x0f, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x23, 0x2e, 0x71,
|
||
0x75, 0x69, 0x6c, 0x69, 0x62, 0x72, 0x69, 0x75, 0x6d, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x6e,
|
||
0x6f, 0x64, 0x65, 0x2e, 0x70, 0x62, 0x2e, 0x43, 0x61, 0x70, 0x61, 0x62, 0x69, 0x6c, 0x69, 0x74,
|
||
0x79, 0x52, 0x0c, 0x63, 0x61, 0x70, 0x61, 0x62, 0x69, 0x6c, 0x69, 0x74, 0x69, 0x65, 0x73, 0x12,
|
||
0x2a, 0x0a, 0x11, 0x6d, 0x61, 0x73, 0x74, 0x65, 0x72, 0x5f, 0x68, 0x65, 0x61, 0x64, 0x5f, 0x66,
|
||
0x72, 0x61, 0x6d, 0x65, 0x18, 0x10, 0x20, 0x01, 0x28, 0x04, 0x52, 0x0f, 0x6d, 0x61, 0x73, 0x74,
|
||
0x65, 0x72, 0x48, 0x65, 0x61, 0x64, 0x46, 0x72, 0x61, 0x6d, 0x65, 0x22, 0x65, 0x0a, 0x15, 0x50,
|
||
0x65, 0x65, 0x72, 0x4d, 0x61, 0x6e, 0x69, 0x66, 0x65, 0x73, 0x74, 0x73, 0x52, 0x65, 0x73, 0x70,
|
||
0x6f, 0x6e, 0x73, 0x65, 0x12, 0x4c, 0x0a, 0x0e, 0x70, 0x65, 0x65, 0x72, 0x5f, 0x6d, 0x61, 0x6e,
|
||
0x69, 0x66, 0x65, 0x73, 0x74, 0x73, 0x18, 0x01, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x25, 0x2e, 0x71,
|
||
0x75, 0x69, 0x6c, 0x69, 0x62, 0x72, 0x69, 0x75, 0x6d, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x6e,
|
||
0x6f, 0x64, 0x65, 0x2e, 0x70, 0x62, 0x2e, 0x50, 0x65, 0x65, 0x72, 0x4d, 0x61, 0x6e, 0x69, 0x66,
|
||
0x65, 0x73, 0x74, 0x52, 0x0d, 0x70, 0x65, 0x65, 0x72, 0x4d, 0x61, 0x6e, 0x69, 0x66, 0x65, 0x73,
|
||
0x74, 0x73, 0x32, 0xd7, 0x01, 0x0a, 0x11, 0x56, 0x61, 0x6c, 0x69, 0x64, 0x61, 0x74, 0x69, 0x6f,
|
||
0x6e, 0x53, 0x65, 0x72, 0x76, 0x69, 0x63, 0x65, 0x12, 0x6b, 0x0a, 0x11, 0x50, 0x65, 0x72, 0x66,
|
||
0x6f, 0x72, 0x6d, 0x56, 0x61, 0x6c, 0x69, 0x64, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x12, 0x2a, 0x2e,
|
||
0x71, 0x75, 0x69, 0x6c, 0x69, 0x62, 0x72, 0x69, 0x75, 0x6d, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e,
|
||
0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x70, 0x62, 0x2e, 0x56, 0x61, 0x6c, 0x69, 0x64, 0x61, 0x74, 0x69,
|
||
0x6f, 0x6e, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x1a, 0x2a, 0x2e, 0x71, 0x75, 0x69, 0x6c,
|
||
0x69, 0x62, 0x72, 0x69, 0x75, 0x6d, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x6e, 0x6f, 0x64, 0x65,
|
||
0x2e, 0x70, 0x62, 0x2e, 0x56, 0x61, 0x6c, 0x69, 0x64, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x4d, 0x65,
|
||
0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x55, 0x0a, 0x04, 0x53, 0x79, 0x6e, 0x63, 0x12, 0x24, 0x2e,
|
||
0x71, 0x75, 0x69, 0x6c, 0x69, 0x62, 0x72, 0x69, 0x75, 0x6d, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e,
|
||
0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x70, 0x62, 0x2e, 0x53, 0x79, 0x6e, 0x63, 0x52, 0x65, 0x71, 0x75,
|
||
0x65, 0x73, 0x74, 0x1a, 0x25, 0x2e, 0x71, 0x75, 0x69, 0x6c, 0x69, 0x62, 0x72, 0x69, 0x75, 0x6d,
|
||
0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x70, 0x62, 0x2e, 0x53, 0x79,
|
||
0x6e, 0x63, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x30, 0x01, 0x32, 0xf6, 0x05, 0x0a,
|
||
0x0b, 0x4e, 0x6f, 0x64, 0x65, 0x53, 0x65, 0x72, 0x76, 0x69, 0x63, 0x65, 0x12, 0x5f, 0x0a, 0x09,
|
||
0x47, 0x65, 0x74, 0x46, 0x72, 0x61, 0x6d, 0x65, 0x73, 0x12, 0x29, 0x2e, 0x71, 0x75, 0x69, 0x6c,
|
||
0x69, 0x62, 0x72, 0x69, 0x75, 0x6d, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x6e, 0x6f, 0x64, 0x65,
|
||
0x2e, 0x70, 0x62, 0x2e, 0x47, 0x65, 0x74, 0x46, 0x72, 0x61, 0x6d, 0x65, 0x73, 0x52, 0x65, 0x71,
|
||
0x75, 0x65, 0x73, 0x74, 0x1a, 0x27, 0x2e, 0x71, 0x75, 0x69, 0x6c, 0x69, 0x62, 0x72, 0x69, 0x75,
|
||
0x6d, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x70, 0x62, 0x2e, 0x46,
|
||
0x72, 0x61, 0x6d, 0x65, 0x73, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x68, 0x0a,
|
||
0x0c, 0x47, 0x65, 0x74, 0x46, 0x72, 0x61, 0x6d, 0x65, 0x49, 0x6e, 0x66, 0x6f, 0x12, 0x2c, 0x2e,
|
||
0x71, 0x75, 0x69, 0x6c, 0x69, 0x62, 0x72, 0x69, 0x75, 0x6d, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e,
|
||
0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x70, 0x62, 0x2e, 0x47, 0x65, 0x74, 0x46, 0x72, 0x61, 0x6d, 0x65,
|
||
0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x2a, 0x2e, 0x71, 0x75,
|
||
0x69, 0x6c, 0x69, 0x62, 0x72, 0x69, 0x75, 0x6d, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x6e, 0x6f,
|
||
0x64, 0x65, 0x2e, 0x70, 0x62, 0x2e, 0x46, 0x72, 0x61, 0x6d, 0x65, 0x49, 0x6e, 0x66, 0x6f, 0x52,
|
||
0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x65, 0x0a, 0x0b, 0x47, 0x65, 0x74, 0x50, 0x65,
|
||
0x65, 0x72, 0x49, 0x6e, 0x66, 0x6f, 0x12, 0x2b, 0x2e, 0x71, 0x75, 0x69, 0x6c, 0x69, 0x62, 0x72,
|
||
0x69, 0x75, 0x6d, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x70, 0x62,
|
||
0x2e, 0x47, 0x65, 0x74, 0x50, 0x65, 0x65, 0x72, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x71, 0x75,
|
||
0x65, 0x73, 0x74, 0x1a, 0x29, 0x2e, 0x71, 0x75, 0x69, 0x6c, 0x69, 0x62, 0x72, 0x69, 0x75, 0x6d,
|
||
0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x70, 0x62, 0x2e, 0x50, 0x65,
|
||
0x65, 0x72, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x65,
|
||
0x0a, 0x0b, 0x47, 0x65, 0x74, 0x4e, 0x6f, 0x64, 0x65, 0x49, 0x6e, 0x66, 0x6f, 0x12, 0x2b, 0x2e,
|
||
0x71, 0x75, 0x69, 0x6c, 0x69, 0x62, 0x72, 0x69, 0x75, 0x6d, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e,
|
||
0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x70, 0x62, 0x2e, 0x47, 0x65, 0x74, 0x4e, 0x6f, 0x64, 0x65, 0x49,
|
||
0x6e, 0x66, 0x6f, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x29, 0x2e, 0x71, 0x75, 0x69,
|
||
0x6c, 0x69, 0x62, 0x72, 0x69, 0x75, 0x6d, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x6e, 0x6f, 0x64,
|
||
0x65, 0x2e, 0x70, 0x62, 0x2e, 0x4e, 0x6f, 0x64, 0x65, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x73,
|
||
0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x6e, 0x0a, 0x0e, 0x47, 0x65, 0x74, 0x4e, 0x65, 0x74, 0x77,
|
||
0x6f, 0x72, 0x6b, 0x49, 0x6e, 0x66, 0x6f, 0x12, 0x2e, 0x2e, 0x71, 0x75, 0x69, 0x6c, 0x69, 0x62,
|
||
0x72, 0x69, 0x75, 0x6d, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x70,
|
||
0x62, 0x2e, 0x47, 0x65, 0x74, 0x4e, 0x65, 0x74, 0x77, 0x6f, 0x72, 0x6b, 0x49, 0x6e, 0x66, 0x6f,
|
||
0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x2c, 0x2e, 0x71, 0x75, 0x69, 0x6c, 0x69, 0x62,
|
||
0x72, 0x69, 0x75, 0x6d, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x70,
|
||
0x62, 0x2e, 0x4e, 0x65, 0x74, 0x77, 0x6f, 0x72, 0x6b, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x73,
|
||
0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x68, 0x0a, 0x0c, 0x47, 0x65, 0x74, 0x54, 0x6f, 0x6b, 0x65,
|
||
0x6e, 0x49, 0x6e, 0x66, 0x6f, 0x12, 0x2c, 0x2e, 0x71, 0x75, 0x69, 0x6c, 0x69, 0x62, 0x72, 0x69,
|
||
0x75, 0x6d, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x70, 0x62, 0x2e,
|
||
0x47, 0x65, 0x74, 0x54, 0x6f, 0x6b, 0x65, 0x6e, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x71, 0x75,
|
||
0x65, 0x73, 0x74, 0x1a, 0x2a, 0x2e, 0x71, 0x75, 0x69, 0x6c, 0x69, 0x62, 0x72, 0x69, 0x75, 0x6d,
|
||
0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x70, 0x62, 0x2e, 0x54, 0x6f,
|
||
0x6b, 0x65, 0x6e, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12,
|
||
0x74, 0x0a, 0x10, 0x47, 0x65, 0x74, 0x50, 0x65, 0x65, 0x72, 0x4d, 0x61, 0x6e, 0x69, 0x66, 0x65,
|
||
0x73, 0x74, 0x73, 0x12, 0x30, 0x2e, 0x71, 0x75, 0x69, 0x6c, 0x69, 0x62, 0x72, 0x69, 0x75, 0x6d,
|
||
0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x70, 0x62, 0x2e, 0x47, 0x65,
|
||
0x74, 0x50, 0x65, 0x65, 0x72, 0x4d, 0x61, 0x6e, 0x69, 0x66, 0x65, 0x73, 0x74, 0x73, 0x52, 0x65,
|
||
0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x2e, 0x2e, 0x71, 0x75, 0x69, 0x6c, 0x69, 0x62, 0x72, 0x69,
|
||
0x75, 0x6d, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x70, 0x62, 0x2e,
|
||
0x50, 0x65, 0x65, 0x72, 0x4d, 0x61, 0x6e, 0x69, 0x66, 0x65, 0x73, 0x74, 0x73, 0x52, 0x65, 0x73,
|
||
0x70, 0x6f, 0x6e, 0x73, 0x65, 0x32, 0xcf, 0x01, 0x0a, 0x09, 0x4e, 0x6f, 0x64, 0x65, 0x53, 0x74,
|
||
0x61, 0x74, 0x73, 0x12, 0x60, 0x0a, 0x0b, 0x50, 0x75, 0x74, 0x4e, 0x6f, 0x64, 0x65, 0x49, 0x6e,
|
||
0x66, 0x6f, 0x12, 0x2b, 0x2e, 0x71, 0x75, 0x69, 0x6c, 0x69, 0x62, 0x72, 0x69, 0x75, 0x6d, 0x2e,
|
||
0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x70, 0x62, 0x2e, 0x50, 0x75, 0x74,
|
||
0x4e, 0x6f, 0x64, 0x65, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x1a,
|
||
0x24, 0x2e, 0x71, 0x75, 0x69, 0x6c, 0x69, 0x62, 0x72, 0x69, 0x75, 0x6d, 0x2e, 0x6e, 0x6f, 0x64,
|
||
0x65, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x70, 0x62, 0x2e, 0x50, 0x75, 0x74, 0x52, 0x65, 0x73,
|
||
0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x60, 0x0a, 0x0b, 0x50, 0x75, 0x74, 0x50, 0x65, 0x65, 0x72,
|
||
0x49, 0x6e, 0x66, 0x6f, 0x12, 0x2b, 0x2e, 0x71, 0x75, 0x69, 0x6c, 0x69, 0x62, 0x72, 0x69, 0x75,
|
||
0x6d, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x70, 0x62, 0x2e, 0x50,
|
||
0x75, 0x74, 0x50, 0x65, 0x65, 0x72, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73,
|
||
0x74, 0x1a, 0x24, 0x2e, 0x71, 0x75, 0x69, 0x6c, 0x69, 0x62, 0x72, 0x69, 0x75, 0x6d, 0x2e, 0x6e,
|
||
0x6f, 0x64, 0x65, 0x2e, 0x6e, 0x6f, 0x64, 0x65, 0x2e, 0x70, 0x62, 0x2e, 0x50, 0x75, 0x74, 0x52,
|
||
0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x42, 0x3a, 0x5a, 0x38, 0x73, 0x6f, 0x75, 0x72, 0x63,
|
||
0x65, 0x2e, 0x71, 0x75, 0x69, 0x6c, 0x69, 0x62, 0x72, 0x69, 0x75, 0x6d, 0x2e, 0x63, 0x6f, 0x6d,
|
||
0x2f, 0x71, 0x75, 0x69, 0x6c, 0x69, 0x62, 0x72, 0x69, 0x75, 0x6d, 0x2f, 0x6d, 0x6f, 0x6e, 0x6f,
|
||
0x72, 0x65, 0x70, 0x6f, 0x2f, 0x6e, 0x6f, 0x64, 0x65, 0x2f, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62,
|
||
0x75, 0x66, 0x73, 0x62, 0x06, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x33,
|
||
}
|
||
|
||
var (
|
||
file_node_proto_rawDescOnce sync.Once
|
||
file_node_proto_rawDescData = file_node_proto_rawDesc
|
||
)
|
||
|
||
func file_node_proto_rawDescGZIP() []byte {
|
||
file_node_proto_rawDescOnce.Do(func() {
|
||
file_node_proto_rawDescData = protoimpl.X.CompressGZIP(file_node_proto_rawDescData)
|
||
})
|
||
return file_node_proto_rawDescData
|
||
}
|
||
|
||
var file_node_proto_msgTypes = make([]protoimpl.MessageInfo, 25)
|
||
var file_node_proto_goTypes = []interface{}{
|
||
(*GetFramesRequest)(nil), // 0: quilibrium.node.node.pb.GetFramesRequest
|
||
(*GetFrameInfoRequest)(nil), // 1: quilibrium.node.node.pb.GetFrameInfoRequest
|
||
(*GetPeerInfoRequest)(nil), // 2: quilibrium.node.node.pb.GetPeerInfoRequest
|
||
(*GetNodeInfoRequest)(nil), // 3: quilibrium.node.node.pb.GetNodeInfoRequest
|
||
(*GetNetworkInfoRequest)(nil), // 4: quilibrium.node.node.pb.GetNetworkInfoRequest
|
||
(*FramesResponse)(nil), // 5: quilibrium.node.node.pb.FramesResponse
|
||
(*FrameInfoResponse)(nil), // 6: quilibrium.node.node.pb.FrameInfoResponse
|
||
(*PeerInfo)(nil), // 7: quilibrium.node.node.pb.PeerInfo
|
||
(*PeerInfoResponse)(nil), // 8: quilibrium.node.node.pb.PeerInfoResponse
|
||
(*NetworkInfo)(nil), // 9: quilibrium.node.node.pb.NetworkInfo
|
||
(*NodeInfoResponse)(nil), // 10: quilibrium.node.node.pb.NodeInfoResponse
|
||
(*PutPeerInfoRequest)(nil), // 11: quilibrium.node.node.pb.PutPeerInfoRequest
|
||
(*PutNodeInfoRequest)(nil), // 12: quilibrium.node.node.pb.PutNodeInfoRequest
|
||
(*PutResponse)(nil), // 13: quilibrium.node.node.pb.PutResponse
|
||
(*NetworkInfoResponse)(nil), // 14: quilibrium.node.node.pb.NetworkInfoResponse
|
||
(*GetTokenInfoRequest)(nil), // 15: quilibrium.node.node.pb.GetTokenInfoRequest
|
||
(*TokenInfoResponse)(nil), // 16: quilibrium.node.node.pb.TokenInfoResponse
|
||
(*Capability)(nil), // 17: quilibrium.node.node.pb.Capability
|
||
(*SelfTestReport)(nil), // 18: quilibrium.node.node.pb.SelfTestReport
|
||
(*ValidationMessage)(nil), // 19: quilibrium.node.node.pb.ValidationMessage
|
||
(*SyncRequest)(nil), // 20: quilibrium.node.node.pb.SyncRequest
|
||
(*SyncResponse)(nil), // 21: quilibrium.node.node.pb.SyncResponse
|
||
(*GetPeerManifestsRequest)(nil), // 22: quilibrium.node.node.pb.GetPeerManifestsRequest
|
||
(*PeerManifest)(nil), // 23: quilibrium.node.node.pb.PeerManifest
|
||
(*PeerManifestsResponse)(nil), // 24: quilibrium.node.node.pb.PeerManifestsResponse
|
||
(*ClockFrame)(nil), // 25: quilibrium.node.clock.pb.ClockFrame
|
||
(*ClockFramesRequest)(nil), // 26: quilibrium.node.clock.pb.ClockFramesRequest
|
||
(*ClockFramesResponse)(nil), // 27: quilibrium.node.clock.pb.ClockFramesResponse
|
||
}
|
||
var file_node_proto_depIdxs = []int32{
|
||
25, // 0: quilibrium.node.node.pb.FramesResponse.truncated_clock_frames:type_name -> quilibrium.node.clock.pb.ClockFrame
|
||
25, // 1: quilibrium.node.node.pb.FrameInfoResponse.clock_frame:type_name -> quilibrium.node.clock.pb.ClockFrame
|
||
7, // 2: quilibrium.node.node.pb.PeerInfoResponse.peer_info:type_name -> quilibrium.node.node.pb.PeerInfo
|
||
7, // 3: quilibrium.node.node.pb.PeerInfoResponse.uncooperative_peer_info:type_name -> quilibrium.node.node.pb.PeerInfo
|
||
7, // 4: quilibrium.node.node.pb.PutPeerInfoRequest.peer_info:type_name -> quilibrium.node.node.pb.PeerInfo
|
||
7, // 5: quilibrium.node.node.pb.PutPeerInfoRequest.uncooperative_peer_info:type_name -> quilibrium.node.node.pb.PeerInfo
|
||
9, // 6: quilibrium.node.node.pb.NetworkInfoResponse.network_info:type_name -> quilibrium.node.node.pb.NetworkInfo
|
||
17, // 7: quilibrium.node.node.pb.SelfTestReport.capabilities:type_name -> quilibrium.node.node.pb.Capability
|
||
26, // 8: quilibrium.node.node.pb.SyncRequest.frames_request:type_name -> quilibrium.node.clock.pb.ClockFramesRequest
|
||
27, // 9: quilibrium.node.node.pb.SyncResponse.frames_response:type_name -> quilibrium.node.clock.pb.ClockFramesResponse
|
||
17, // 10: quilibrium.node.node.pb.PeerManifest.capabilities:type_name -> quilibrium.node.node.pb.Capability
|
||
23, // 11: quilibrium.node.node.pb.PeerManifestsResponse.peer_manifests:type_name -> quilibrium.node.node.pb.PeerManifest
|
||
19, // 12: quilibrium.node.node.pb.ValidationService.PerformValidation:input_type -> quilibrium.node.node.pb.ValidationMessage
|
||
20, // 13: quilibrium.node.node.pb.ValidationService.Sync:input_type -> quilibrium.node.node.pb.SyncRequest
|
||
0, // 14: quilibrium.node.node.pb.NodeService.GetFrames:input_type -> quilibrium.node.node.pb.GetFramesRequest
|
||
1, // 15: quilibrium.node.node.pb.NodeService.GetFrameInfo:input_type -> quilibrium.node.node.pb.GetFrameInfoRequest
|
||
2, // 16: quilibrium.node.node.pb.NodeService.GetPeerInfo:input_type -> quilibrium.node.node.pb.GetPeerInfoRequest
|
||
3, // 17: quilibrium.node.node.pb.NodeService.GetNodeInfo:input_type -> quilibrium.node.node.pb.GetNodeInfoRequest
|
||
4, // 18: quilibrium.node.node.pb.NodeService.GetNetworkInfo:input_type -> quilibrium.node.node.pb.GetNetworkInfoRequest
|
||
15, // 19: quilibrium.node.node.pb.NodeService.GetTokenInfo:input_type -> quilibrium.node.node.pb.GetTokenInfoRequest
|
||
22, // 20: quilibrium.node.node.pb.NodeService.GetPeerManifests:input_type -> quilibrium.node.node.pb.GetPeerManifestsRequest
|
||
12, // 21: quilibrium.node.node.pb.NodeStats.PutNodeInfo:input_type -> quilibrium.node.node.pb.PutNodeInfoRequest
|
||
11, // 22: quilibrium.node.node.pb.NodeStats.PutPeerInfo:input_type -> quilibrium.node.node.pb.PutPeerInfoRequest
|
||
19, // 23: quilibrium.node.node.pb.ValidationService.PerformValidation:output_type -> quilibrium.node.node.pb.ValidationMessage
|
||
21, // 24: quilibrium.node.node.pb.ValidationService.Sync:output_type -> quilibrium.node.node.pb.SyncResponse
|
||
5, // 25: quilibrium.node.node.pb.NodeService.GetFrames:output_type -> quilibrium.node.node.pb.FramesResponse
|
||
6, // 26: quilibrium.node.node.pb.NodeService.GetFrameInfo:output_type -> quilibrium.node.node.pb.FrameInfoResponse
|
||
8, // 27: quilibrium.node.node.pb.NodeService.GetPeerInfo:output_type -> quilibrium.node.node.pb.PeerInfoResponse
|
||
10, // 28: quilibrium.node.node.pb.NodeService.GetNodeInfo:output_type -> quilibrium.node.node.pb.NodeInfoResponse
|
||
14, // 29: quilibrium.node.node.pb.NodeService.GetNetworkInfo:output_type -> quilibrium.node.node.pb.NetworkInfoResponse
|
||
16, // 30: quilibrium.node.node.pb.NodeService.GetTokenInfo:output_type -> quilibrium.node.node.pb.TokenInfoResponse
|
||
24, // 31: quilibrium.node.node.pb.NodeService.GetPeerManifests:output_type -> quilibrium.node.node.pb.PeerManifestsResponse
|
||
13, // 32: quilibrium.node.node.pb.NodeStats.PutNodeInfo:output_type -> quilibrium.node.node.pb.PutResponse
|
||
13, // 33: quilibrium.node.node.pb.NodeStats.PutPeerInfo:output_type -> quilibrium.node.node.pb.PutResponse
|
||
23, // [23:34] is the sub-list for method output_type
|
||
12, // [12:23] is the sub-list for method input_type
|
||
12, // [12:12] is the sub-list for extension type_name
|
||
12, // [12:12] is the sub-list for extension extendee
|
||
0, // [0:12] is the sub-list for field type_name
|
||
}
|
||
|
||
func init() { file_node_proto_init() }
|
||
func file_node_proto_init() {
|
||
if File_node_proto != nil {
|
||
return
|
||
}
|
||
file_clock_proto_init()
|
||
if !protoimpl.UnsafeEnabled {
|
||
file_node_proto_msgTypes[0].Exporter = func(v interface{}, i int) interface{} {
|
||
switch v := v.(*GetFramesRequest); i {
|
||
case 0:
|
||
return &v.state
|
||
case 1:
|
||
return &v.sizeCache
|
||
case 2:
|
||
return &v.unknownFields
|
||
default:
|
||
return nil
|
||
}
|
||
}
|
||
file_node_proto_msgTypes[1].Exporter = func(v interface{}, i int) interface{} {
|
||
switch v := v.(*GetFrameInfoRequest); i {
|
||
case 0:
|
||
return &v.state
|
||
case 1:
|
||
return &v.sizeCache
|
||
case 2:
|
||
return &v.unknownFields
|
||
default:
|
||
return nil
|
||
}
|
||
}
|
||
file_node_proto_msgTypes[2].Exporter = func(v interface{}, i int) interface{} {
|
||
switch v := v.(*GetPeerInfoRequest); i {
|
||
case 0:
|
||
return &v.state
|
||
case 1:
|
||
return &v.sizeCache
|
||
case 2:
|
||
return &v.unknownFields
|
||
default:
|
||
return nil
|
||
}
|
||
}
|
||
file_node_proto_msgTypes[3].Exporter = func(v interface{}, i int) interface{} {
|
||
switch v := v.(*GetNodeInfoRequest); i {
|
||
case 0:
|
||
return &v.state
|
||
case 1:
|
||
return &v.sizeCache
|
||
case 2:
|
||
return &v.unknownFields
|
||
default:
|
||
return nil
|
||
}
|
||
}
|
||
file_node_proto_msgTypes[4].Exporter = func(v interface{}, i int) interface{} {
|
||
switch v := v.(*GetNetworkInfoRequest); i {
|
||
case 0:
|
||
return &v.state
|
||
case 1:
|
||
return &v.sizeCache
|
||
case 2:
|
||
return &v.unknownFields
|
||
default:
|
||
return nil
|
||
}
|
||
}
|
||
file_node_proto_msgTypes[5].Exporter = func(v interface{}, i int) interface{} {
|
||
switch v := v.(*FramesResponse); i {
|
||
case 0:
|
||
return &v.state
|
||
case 1:
|
||
return &v.sizeCache
|
||
case 2:
|
||
return &v.unknownFields
|
||
default:
|
||
return nil
|
||
}
|
||
}
|
||
file_node_proto_msgTypes[6].Exporter = func(v interface{}, i int) interface{} {
|
||
switch v := v.(*FrameInfoResponse); i {
|
||
case 0:
|
||
return &v.state
|
||
case 1:
|
||
return &v.sizeCache
|
||
case 2:
|
||
return &v.unknownFields
|
||
default:
|
||
return nil
|
||
}
|
||
}
|
||
file_node_proto_msgTypes[7].Exporter = func(v interface{}, i int) interface{} {
|
||
switch v := v.(*PeerInfo); i {
|
||
case 0:
|
||
return &v.state
|
||
case 1:
|
||
return &v.sizeCache
|
||
case 2:
|
||
return &v.unknownFields
|
||
default:
|
||
return nil
|
||
}
|
||
}
|
||
file_node_proto_msgTypes[8].Exporter = func(v interface{}, i int) interface{} {
|
||
switch v := v.(*PeerInfoResponse); i {
|
||
case 0:
|
||
return &v.state
|
||
case 1:
|
||
return &v.sizeCache
|
||
case 2:
|
||
return &v.unknownFields
|
||
default:
|
||
return nil
|
||
}
|
||
}
|
||
file_node_proto_msgTypes[9].Exporter = func(v interface{}, i int) interface{} {
|
||
switch v := v.(*NetworkInfo); i {
|
||
case 0:
|
||
return &v.state
|
||
case 1:
|
||
return &v.sizeCache
|
||
case 2:
|
||
return &v.unknownFields
|
||
default:
|
||
return nil
|
||
}
|
||
}
|
||
file_node_proto_msgTypes[10].Exporter = func(v interface{}, i int) interface{} {
|
||
switch v := v.(*NodeInfoResponse); i {
|
||
case 0:
|
||
return &v.state
|
||
case 1:
|
||
return &v.sizeCache
|
||
case 2:
|
||
return &v.unknownFields
|
||
default:
|
||
return nil
|
||
}
|
||
}
|
||
file_node_proto_msgTypes[11].Exporter = func(v interface{}, i int) interface{} {
|
||
switch v := v.(*PutPeerInfoRequest); i {
|
||
case 0:
|
||
return &v.state
|
||
case 1:
|
||
return &v.sizeCache
|
||
case 2:
|
||
return &v.unknownFields
|
||
default:
|
||
return nil
|
||
}
|
||
}
|
||
file_node_proto_msgTypes[12].Exporter = func(v interface{}, i int) interface{} {
|
||
switch v := v.(*PutNodeInfoRequest); i {
|
||
case 0:
|
||
return &v.state
|
||
case 1:
|
||
return &v.sizeCache
|
||
case 2:
|
||
return &v.unknownFields
|
||
default:
|
||
return nil
|
||
}
|
||
}
|
||
file_node_proto_msgTypes[13].Exporter = func(v interface{}, i int) interface{} {
|
||
switch v := v.(*PutResponse); i {
|
||
case 0:
|
||
return &v.state
|
||
case 1:
|
||
return &v.sizeCache
|
||
case 2:
|
||
return &v.unknownFields
|
||
default:
|
||
return nil
|
||
}
|
||
}
|
||
file_node_proto_msgTypes[14].Exporter = func(v interface{}, i int) interface{} {
|
||
switch v := v.(*NetworkInfoResponse); i {
|
||
case 0:
|
||
return &v.state
|
||
case 1:
|
||
return &v.sizeCache
|
||
case 2:
|
||
return &v.unknownFields
|
||
default:
|
||
return nil
|
||
}
|
||
}
|
||
file_node_proto_msgTypes[15].Exporter = func(v interface{}, i int) interface{} {
|
||
switch v := v.(*GetTokenInfoRequest); i {
|
||
case 0:
|
||
return &v.state
|
||
case 1:
|
||
return &v.sizeCache
|
||
case 2:
|
||
return &v.unknownFields
|
||
default:
|
||
return nil
|
||
}
|
||
}
|
||
file_node_proto_msgTypes[16].Exporter = func(v interface{}, i int) interface{} {
|
||
switch v := v.(*TokenInfoResponse); i {
|
||
case 0:
|
||
return &v.state
|
||
case 1:
|
||
return &v.sizeCache
|
||
case 2:
|
||
return &v.unknownFields
|
||
default:
|
||
return nil
|
||
}
|
||
}
|
||
file_node_proto_msgTypes[17].Exporter = func(v interface{}, i int) interface{} {
|
||
switch v := v.(*Capability); i {
|
||
case 0:
|
||
return &v.state
|
||
case 1:
|
||
return &v.sizeCache
|
||
case 2:
|
||
return &v.unknownFields
|
||
default:
|
||
return nil
|
||
}
|
||
}
|
||
file_node_proto_msgTypes[18].Exporter = func(v interface{}, i int) interface{} {
|
||
switch v := v.(*SelfTestReport); i {
|
||
case 0:
|
||
return &v.state
|
||
case 1:
|
||
return &v.sizeCache
|
||
case 2:
|
||
return &v.unknownFields
|
||
default:
|
||
return nil
|
||
}
|
||
}
|
||
file_node_proto_msgTypes[19].Exporter = func(v interface{}, i int) interface{} {
|
||
switch v := v.(*ValidationMessage); i {
|
||
case 0:
|
||
return &v.state
|
||
case 1:
|
||
return &v.sizeCache
|
||
case 2:
|
||
return &v.unknownFields
|
||
default:
|
||
return nil
|
||
}
|
||
}
|
||
file_node_proto_msgTypes[20].Exporter = func(v interface{}, i int) interface{} {
|
||
switch v := v.(*SyncRequest); i {
|
||
case 0:
|
||
return &v.state
|
||
case 1:
|
||
return &v.sizeCache
|
||
case 2:
|
||
return &v.unknownFields
|
||
default:
|
||
return nil
|
||
}
|
||
}
|
||
file_node_proto_msgTypes[21].Exporter = func(v interface{}, i int) interface{} {
|
||
switch v := v.(*SyncResponse); i {
|
||
case 0:
|
||
return &v.state
|
||
case 1:
|
||
return &v.sizeCache
|
||
case 2:
|
||
return &v.unknownFields
|
||
default:
|
||
return nil
|
||
}
|
||
}
|
||
file_node_proto_msgTypes[22].Exporter = func(v interface{}, i int) interface{} {
|
||
switch v := v.(*GetPeerManifestsRequest); i {
|
||
case 0:
|
||
return &v.state
|
||
case 1:
|
||
return &v.sizeCache
|
||
case 2:
|
||
return &v.unknownFields
|
||
default:
|
||
return nil
|
||
}
|
||
}
|
||
file_node_proto_msgTypes[23].Exporter = func(v interface{}, i int) interface{} {
|
||
switch v := v.(*PeerManifest); i {
|
||
case 0:
|
||
return &v.state
|
||
case 1:
|
||
return &v.sizeCache
|
||
case 2:
|
||
return &v.unknownFields
|
||
default:
|
||
return nil
|
||
}
|
||
}
|
||
file_node_proto_msgTypes[24].Exporter = func(v interface{}, i int) interface{} {
|
||
switch v := v.(*PeerManifestsResponse); i {
|
||
case 0:
|
||
return &v.state
|
||
case 1:
|
||
return &v.sizeCache
|
||
case 2:
|
||
return &v.unknownFields
|
||
default:
|
||
return nil
|
||
}
|
||
}
|
||
}
|
||
type x struct{}
|
||
out := protoimpl.TypeBuilder{
|
||
File: protoimpl.DescBuilder{
|
||
GoPackagePath: reflect.TypeOf(x{}).PkgPath(),
|
||
RawDescriptor: file_node_proto_rawDesc,
|
||
NumEnums: 0,
|
||
NumMessages: 25,
|
||
NumExtensions: 0,
|
||
NumServices: 3,
|
||
},
|
||
GoTypes: file_node_proto_goTypes,
|
||
DependencyIndexes: file_node_proto_depIdxs,
|
||
MessageInfos: file_node_proto_msgTypes,
|
||
}.Build()
|
||
File_node_proto = out.File
|
||
file_node_proto_rawDesc = nil
|
||
file_node_proto_goTypes = nil
|
||
file_node_proto_depIdxs = nil
|
||
}
|