mirror of
https://source.quilibrium.com/quilibrium/ceremonyclient.git
synced 2024-11-10 18:25:17 +00:00
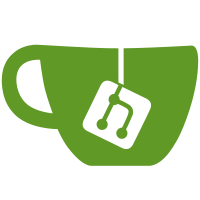
* add version label and trim repo url * add README for protobufs * add version to NodeInfoResponse * add docker login task * remove version from docker compose file * return version with GetNodeInfo response * add basic -node-info flag * print max frame as well with -node-info * expand protobuf README * update node command examples to use node binary and make log commands follow * return all of NodeInfo * extract FormatVersion * print version of running process
52 lines
1.2 KiB
Go
52 lines
1.2 KiB
Go
package config
|
|
|
|
import (
|
|
"github.com/stretchr/testify/assert"
|
|
"regexp"
|
|
"testing"
|
|
"time"
|
|
)
|
|
|
|
func TestGetMinimumVersion(t *testing.T) {
|
|
minVersion := GetMinimumVersion()
|
|
|
|
assert.Equal(t, 3, len(minVersion), "Expected version to have exactly 3 bytes; got %v", len(minVersion))
|
|
|
|
version := GetVersion()
|
|
|
|
assert.GreaterOrEqual(t, version[0], minVersion[0])
|
|
|
|
if minVersion[0] == version[0] {
|
|
assert.GreaterOrEqual(t, version[1], minVersion[1])
|
|
|
|
if minVersion[1] == version[1] {
|
|
assert.GreaterOrEqual(t, version[2], minVersion[2])
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestGetVersion(t *testing.T) {
|
|
version := GetVersion()
|
|
|
|
assert.Equal(t, 3, len(version), "Expected version to have exactly 3 bytes; got %v", len(version))
|
|
}
|
|
|
|
func TestGetMinimumVersionCutoff(t *testing.T) {
|
|
cutoff := GetMinimumVersionCutoff()
|
|
|
|
assert.True(t, cutoff.Before(time.Now()))
|
|
assert.True(t, cutoff.After(time.Date(2024, time.March, 1, 0, 0, 0, 0, time.UTC)))
|
|
}
|
|
|
|
func TestGetVersionString(t *testing.T) {
|
|
version := GetVersionString()
|
|
|
|
versionRegexp := regexp.MustCompile("[0-9]+\\.[0-9]+\\.[0-9]+")
|
|
|
|
assert.Regexp(t, versionRegexp, version)
|
|
}
|
|
|
|
func TestFormatVersion(t *testing.T) {
|
|
assert.Equal(t, "1.4.12", FormatVersion([]byte{1, 4, 12}))
|
|
}
|