mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-11-10 18:15:19 +00:00
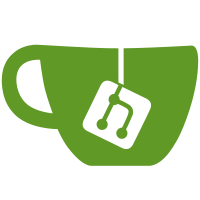
- Upgrade cosmos-sdk to v0.44.5 from v0.39.2 - Add Legacy Tx Endpoint for backwards compatibility - Add IBC v1.2.3 Support Co-authored-by: DracoLi <draco@dracoli.com> Co-authored-by: drklee3 <derrick@dlee.dev> Co-authored-by: denalimarsh <denalimarsh@gmail.com> Co-authored-by: Draco Li <draco@kava.io> Co-authored-by: Nick DeLuca <nickdeluca08@gmail.com> Co-authored-by: Kevin Davis <karzak@users.noreply.github.com> Co-authored-by: Denali Marsh <denali@kava.io>
52 lines
1.6 KiB
Go
52 lines
1.6 KiB
Go
package rest
|
|
|
|
import (
|
|
"net/http"
|
|
|
|
"github.com/gorilla/mux"
|
|
|
|
"github.com/cosmos/cosmos-sdk/client"
|
|
clientrest "github.com/cosmos/cosmos-sdk/client/rest"
|
|
"github.com/cosmos/cosmos-sdk/client/tx"
|
|
"github.com/cosmos/cosmos-sdk/types/rest"
|
|
govrest "github.com/cosmos/cosmos-sdk/x/gov/client/rest"
|
|
govtypes "github.com/cosmos/cosmos-sdk/x/gov/types"
|
|
|
|
"github.com/kava-labs/kava/x/kavadist/types"
|
|
)
|
|
|
|
// RegisterRoutes registers kavadist-related REST handlers to a router
|
|
func RegisterRoutes(cliCtx client.Context, rtr *mux.Router) {
|
|
r := clientrest.WithHTTPDeprecationHeaders(rtr)
|
|
registerQueryRoutes(cliCtx, r)
|
|
}
|
|
|
|
// ProposalRESTHandler returns a ProposalRESTHandler that exposes the community pool multi-spend REST handler with a given sub-route.
|
|
func ProposalRESTHandler(cliCtx client.Context) govrest.ProposalRESTHandler {
|
|
return govrest.ProposalRESTHandler{
|
|
SubRoute: types.ProposalTypeCommunityPoolMultiSpend,
|
|
Handler: postProposalHandlerFn(cliCtx),
|
|
}
|
|
}
|
|
func postProposalHandlerFn(cliCtx client.Context) http.HandlerFunc {
|
|
return func(w http.ResponseWriter, r *http.Request) {
|
|
var req CommunityPoolMultiSpendProposalReq
|
|
if !rest.ReadRESTReq(w, r, cliCtx.LegacyAmino, &req) {
|
|
return
|
|
}
|
|
req.BaseReq = req.BaseReq.Sanitize()
|
|
if !req.BaseReq.ValidateBasic(w) {
|
|
return
|
|
}
|
|
content := types.NewCommunityPoolMultiSpendProposal(req.Title, req.Description, req.RecipientList)
|
|
msg, err := govtypes.NewMsgSubmitProposal(content, req.Deposit, req.Proposer)
|
|
if rest.CheckBadRequestError(w, err) {
|
|
return
|
|
}
|
|
if rest.CheckBadRequestError(w, msg.ValidateBasic()) {
|
|
return
|
|
}
|
|
tx.WriteGeneratedTxResponse(cliCtx, w, req.BaseReq, msg)
|
|
}
|
|
}
|