mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-11-10 10:05:18 +00:00
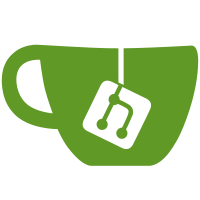
* wip: tpyes and keeper methods * wip: iterators * wip: types and keeper methods * wip: add msgs * wip: client methods * wip: rebase develop * wip: types tests * wip: keeper tests, small fixes * wip: add cdp tests * wip: deposit tests * wip: keeper tests * wip: tests and module methods * feat: error when fetching expired price * feat: conversion factor for external assets * feat: debt floor for new cdps * feat: save deposits on export genesis * feat: ensure messages implement msg * feat: index deposits by status * fix: stray comment * wip: address review comments * address review comments * wip: move liquidation to cdp module * wip: handle liquidations directly * wip: use new auction interface * feat: auction collateral in cdp begin block * feat: update param validation * feat: surplus and debt auctions * address review comments * address review comments * fix: auction multiple deposits * clean up netting function
84 lines
2.4 KiB
Go
84 lines
2.4 KiB
Go
package cdp
|
|
|
|
import (
|
|
"fmt"
|
|
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
)
|
|
|
|
// InitGenesis sets initial genesis state for cdp module
|
|
func InitGenesis(ctx sdk.Context, k Keeper, pk PricefeedKeeper, gs GenesisState) {
|
|
if err := gs.Validate(); err != nil {
|
|
panic(fmt.Sprintf("failed to validate %s genesis state: %s", ModuleName, err))
|
|
}
|
|
|
|
// validate denoms - check that any collaterals in the params are in the pricefeed,
|
|
// pricefeed MUST call InitGenesis before cdp
|
|
collateralMap := make(map[string]int)
|
|
ap := pk.GetParams(ctx)
|
|
for _, a := range ap.Markets {
|
|
collateralMap[a.MarketID] = 1
|
|
}
|
|
|
|
for _, col := range gs.Params.CollateralParams {
|
|
_, found := collateralMap[col.MarketID]
|
|
if !found {
|
|
panic(fmt.Sprintf("%s collateral not found in pricefeed", col.Denom))
|
|
}
|
|
}
|
|
|
|
k.SetParams(ctx, gs.Params)
|
|
|
|
// set the per second fee rate for each collateral type
|
|
for _, cp := range gs.Params.CollateralParams {
|
|
for _, dp := range gs.Params.DebtParams {
|
|
k.SetTotalPrincipal(ctx, cp.Denom, dp.Denom, sdk.ZeroInt())
|
|
}
|
|
k.SetFeeRate(ctx, cp.Denom, cp.StabilityFee)
|
|
}
|
|
|
|
// add cdps
|
|
for _, cdp := range gs.CDPs {
|
|
if cdp.ID == gs.StartingCdpID {
|
|
panic(fmt.Sprintf("starting cdp id is assigned to an existing cdp: %s", cdp))
|
|
}
|
|
k.SetCDP(ctx, cdp)
|
|
k.IndexCdpByOwner(ctx, cdp)
|
|
ratio := k.CalculateCollateralToDebtRatio(ctx, cdp.Collateral, cdp.Principal.Add(cdp.AccumulatedFees))
|
|
k.IndexCdpByCollateralRatio(ctx, cdp.Collateral[0].Denom, cdp.ID, ratio)
|
|
k.IncrementTotalPrincipal(ctx, cdp.Collateral[0].Denom, cdp.Principal)
|
|
}
|
|
|
|
k.SetNextCdpID(ctx, gs.StartingCdpID)
|
|
k.SetDebtDenom(ctx, gs.DebtDenom)
|
|
k.SetGovDenom(ctx, gs.GovDenom)
|
|
|
|
for _, d := range gs.Deposits {
|
|
k.SetDeposit(ctx, d)
|
|
}
|
|
// only set the previous block time if it's different than default
|
|
if !gs.PreviousBlockTime.Equal(DefaultPreviousBlockTime) {
|
|
k.SetPreviousBlockTime(ctx, gs.PreviousBlockTime)
|
|
}
|
|
}
|
|
|
|
// ExportGenesis export genesis state for cdp module
|
|
func ExportGenesis(ctx sdk.Context, k Keeper) GenesisState {
|
|
params := k.GetParams(ctx)
|
|
cdps := k.GetAllCdps(ctx)
|
|
cdpID := k.GetNextCdpID(ctx)
|
|
previousBlockTime, found := k.GetPreviousBlockTime(ctx)
|
|
if !found {
|
|
previousBlockTime = DefaultPreviousBlockTime
|
|
}
|
|
debtDenom := k.GetDebtDenom(ctx)
|
|
|
|
return GenesisState{
|
|
Params: params,
|
|
StartingCdpID: cdpID,
|
|
CDPs: cdps,
|
|
PreviousBlockTime: previousBlockTime,
|
|
DebtDenom: debtDenom,
|
|
}
|
|
}
|