mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-11-10 18:15:19 +00:00
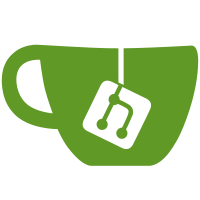
* Initial e2e setup * Fix inflation disable tests * Add upgrade handler * Add param tests for after upgrade * Replace deprecated grpc creds * Remove upgrade for e2e test * Update upgrade handler to set x/community params * Remove params check in upgrade * Update tests for switchover time and params check * wip inflation * Add attribute to disable inflation event * Add before/after switchover mint and dist checks * Add missing attribute to disable inflation test check * Check mint events are 0 * Check total supply doesn't change * Check inflation and events before switchover * Check staking reward payouts from x/community * move events funcs to util * Add keyring to chain, fetch keys from kvtool and test withdrawal * Remove duplicate KavaHomePath * Update subtest names to specify before/after switchover Co-authored-by: Draco <draco@dracoli.com> * Use blocktime for InflationStop event DisableTime * Test 5 blocks for staking rewards payout * Remove logging and unused lines * Check val claimed balance with queried * Enable and update consolidation tests * Update test for modified EventTypeInflationStop time attr * Test x/distribution community tax * Fix test names * Update e2e tests for better live network test support (#1749) * Update e2e tests to support mirrornet * Skip claim rewards on live network, require no errors for existing tests * Update readme with upgrade height * Update .env example with usdt contract address * Restore .env file to original local e2e * Log community params when set * Make AttributeKeyInflationDisableTime more precise * Add mainnet and testnet community params (#1753) * Re-enable ibc tests * Remove duplicate types.EventTypeInflationStop emit * feat: set validator minimum commissions to at least 5% in upgrade handler (#1761) * Update validator min commission in upgrade * Add min commission upgrade test * Update changelog * Set validator MaxRate, call BeforeValidatorModified hook * Check max commission and update time in tests * Update e2e test for max rate * Test val update time * Use SdkBlock instead of Block * Remove upgrade related handlers and tests Preserve any module and test util changes * Update e2e x/community params proposal test to work without upgrade handler --------- Co-authored-by: Draco <draco@dracoli.com>
197 lines
5.8 KiB
Go
197 lines
5.8 KiB
Go
package e2e_test
|
|
|
|
import (
|
|
"context"
|
|
"encoding/hex"
|
|
"time"
|
|
|
|
sdkmath "cosmossdk.io/math"
|
|
"github.com/cosmos/cosmos-sdk/codec"
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
authtypes "github.com/cosmos/cosmos-sdk/x/auth/types"
|
|
govtypes "github.com/cosmos/cosmos-sdk/x/gov/types"
|
|
govv1 "github.com/cosmos/cosmos-sdk/x/gov/types/v1"
|
|
|
|
"github.com/kava-labs/kava/tests/e2e/testutil"
|
|
"github.com/kava-labs/kava/tests/util"
|
|
communitytypes "github.com/kava-labs/kava/x/community/types"
|
|
)
|
|
|
|
func (suite *IntegrationTestSuite) TestCommunityUpdateParams_NonAuthority() {
|
|
// ARRANGE
|
|
// setup kava account
|
|
funds := ukava(1e5) // .1 KAVA
|
|
kavaAcc := suite.Kava.NewFundedAccount("community-non-authority", sdk.NewCoins(funds))
|
|
|
|
gasLimit := int64(2e5)
|
|
fee := ukava(200)
|
|
|
|
msg := communitytypes.NewMsgUpdateParams(
|
|
kavaAcc.SdkAddress,
|
|
communitytypes.DefaultParams(),
|
|
)
|
|
|
|
// ACT
|
|
req := util.KavaMsgRequest{
|
|
Msgs: []sdk.Msg{&msg},
|
|
GasLimit: uint64(gasLimit),
|
|
FeeAmount: sdk.NewCoins(fee),
|
|
Memo: "this is a failure!",
|
|
}
|
|
res := kavaAcc.SignAndBroadcastKavaTx(req)
|
|
|
|
// ASSERT
|
|
_, err := util.WaitForSdkTxCommit(suite.Kava.Tx, res.Result.TxHash, 6*time.Second)
|
|
suite.Require().Error(err)
|
|
suite.Require().ErrorContains(
|
|
err,
|
|
govtypes.ErrInvalidSigner.Error(),
|
|
"should return with authority check error",
|
|
)
|
|
}
|
|
|
|
func (suite *IntegrationTestSuite) TestCommunityUpdateParams_Authority() {
|
|
// ARRANGE
|
|
govParamsRes, err := suite.Kava.Gov.Params(context.Background(), &govv1.QueryParamsRequest{
|
|
ParamsType: govv1.ParamDeposit,
|
|
})
|
|
suite.NoError(err)
|
|
|
|
// Check initial params
|
|
communityParamsResInitial, err := suite.Kava.Community.Params(
|
|
context.Background(),
|
|
&communitytypes.QueryParamsRequest{},
|
|
)
|
|
suite.Require().NoError(err)
|
|
|
|
// setup kava account
|
|
// .1 KAVA + min deposit amount for proposal
|
|
funds := sdk.NewCoins(ukava(1e5)).Add(govParamsRes.DepositParams.MinDeposit...)
|
|
kavaAcc := suite.Kava.NewFundedAccount("community-update-params", funds)
|
|
|
|
gasLimit := int64(2e5)
|
|
fee := ukava(200)
|
|
|
|
// Wait until switchover actually happens - When testing without the upgrade
|
|
// handler that sets a relative switchover time, the switchover time in
|
|
// genesis should be set in the past so it runs immediately.
|
|
suite.Require().Eventually(
|
|
func() bool {
|
|
params, err := suite.Kava.Community.Params(
|
|
context.Background(),
|
|
&communitytypes.QueryParamsRequest{},
|
|
)
|
|
suite.Require().NoError(err)
|
|
|
|
return params.Params.UpgradeTimeDisableInflation.Equal(time.Time{})
|
|
},
|
|
20*time.Second,
|
|
1*time.Second,
|
|
"switchover should happen",
|
|
)
|
|
|
|
// Add 1 to the staking rewards per second
|
|
newStakingRewardsPerSecond := communityParamsResInitial.Params.
|
|
StakingRewardsPerSecond.
|
|
Add(sdkmath.LegacyNewDec(1))
|
|
|
|
// 1. Proposal
|
|
// Only modify stakingRewardsPerSecond, as to not re-run the switchover and
|
|
// to not influence other tests
|
|
updateParamsMsg := communitytypes.NewMsgUpdateParams(
|
|
authtypes.NewModuleAddress(govtypes.ModuleName), // authority
|
|
communitytypes.NewParams(
|
|
time.Time{}, // after switchover, is empty
|
|
newStakingRewardsPerSecond, // only modify stakingRewardsPerSecond
|
|
communityParamsResInitial.Params.UpgradeTimeSetStakingRewardsPerSecond,
|
|
),
|
|
)
|
|
|
|
// Make sure we're actually changing the params
|
|
suite.NotEqual(
|
|
updateParamsMsg.Params,
|
|
communityParamsResInitial.Params,
|
|
"new params should be different from existing",
|
|
)
|
|
|
|
proposalMsg, err := govv1.NewMsgSubmitProposal(
|
|
[]sdk.Msg{&updateParamsMsg},
|
|
govParamsRes.DepositParams.MinDeposit,
|
|
kavaAcc.SdkAddress.String(),
|
|
"community-update-params",
|
|
)
|
|
suite.NoError(err)
|
|
|
|
req := util.KavaMsgRequest{
|
|
Msgs: []sdk.Msg{proposalMsg},
|
|
GasLimit: uint64(gasLimit),
|
|
FeeAmount: sdk.NewCoins(fee),
|
|
Memo: "this is a proposal please accept me",
|
|
}
|
|
res := kavaAcc.SignAndBroadcastKavaTx(req)
|
|
suite.Require().NoError(res.Err)
|
|
|
|
// Wait for proposal to be submitted
|
|
txRes, err := util.WaitForSdkTxCommit(suite.Kava.Tx, res.Result.TxHash, 6*time.Second)
|
|
suite.Require().NoError(err)
|
|
|
|
// Parse tx response to get proposal id
|
|
var govRes govv1.MsgSubmitProposalResponse
|
|
suite.decodeTxMsgResponse(txRes, &govRes)
|
|
|
|
// 2. Vote for proposal from whale account
|
|
whale := suite.Kava.GetAccount(testutil.FundedAccountName)
|
|
voteMsg := govv1.NewMsgVote(
|
|
whale.SdkAddress,
|
|
govRes.ProposalId,
|
|
govv1.OptionYes,
|
|
"",
|
|
)
|
|
|
|
voteReq := util.KavaMsgRequest{
|
|
Msgs: []sdk.Msg{voteMsg},
|
|
GasLimit: uint64(gasLimit),
|
|
FeeAmount: sdk.NewCoins(fee),
|
|
Memo: "voting",
|
|
}
|
|
voteRes := whale.SignAndBroadcastKavaTx(voteReq)
|
|
suite.Require().NoError(voteRes.Err)
|
|
|
|
_, err = util.WaitForSdkTxCommit(suite.Kava.Tx, voteRes.Result.TxHash, 6*time.Second)
|
|
suite.Require().NoError(err)
|
|
|
|
// 3. Wait until proposal passes
|
|
suite.Require().Eventually(func() bool {
|
|
proposalRes, err := suite.Kava.Gov.Proposal(context.Background(), &govv1.QueryProposalRequest{
|
|
ProposalId: govRes.ProposalId,
|
|
})
|
|
suite.NoError(err)
|
|
|
|
return proposalRes.Proposal.Status == govv1.StatusPassed
|
|
}, 60*time.Second, 1*time.Second)
|
|
|
|
// Check parameters are updated
|
|
communityParamsRes, err := suite.Kava.Community.Params(
|
|
context.Background(),
|
|
&communitytypes.QueryParamsRequest{},
|
|
)
|
|
suite.Require().NoError(err)
|
|
|
|
suite.Equal(updateParamsMsg.Params, communityParamsRes.Params)
|
|
}
|
|
|
|
func (suite *IntegrationTestSuite) decodeTxMsgResponse(txRes *sdk.TxResponse, ptr codec.ProtoMarshaler) {
|
|
// convert txRes.Data hex string to bytes
|
|
txResBytes, err := hex.DecodeString(txRes.Data)
|
|
suite.Require().NoError(err)
|
|
|
|
// Unmarshal data to TxMsgData
|
|
var txMsgData sdk.TxMsgData
|
|
suite.Kava.EncodingConfig.Marshaler.MustUnmarshal(txResBytes, &txMsgData)
|
|
suite.T().Logf("txData.MsgResponses: %v", txMsgData.MsgResponses)
|
|
|
|
// Parse MsgResponse
|
|
suite.Kava.EncodingConfig.Marshaler.MustUnmarshal(txMsgData.MsgResponses[0].Value, ptr)
|
|
suite.Require().NoError(err)
|
|
}
|