mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 14:47:31 +00:00
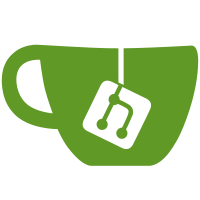
* add tx for CommunityPoolLendDepositProposal * add cli cmd for CommunityPoolWithdrawProposal
40 lines
1.0 KiB
Go
40 lines
1.0 KiB
Go
package utils
|
|
|
|
import (
|
|
"os"
|
|
|
|
"github.com/cosmos/cosmos-sdk/codec"
|
|
|
|
"github.com/kava-labs/kava/x/community/types"
|
|
)
|
|
|
|
// ParseCommunityPoolLendDepositProposal reads a JSON file and parses it to a CommunityPoolLendDepositProposal
|
|
func ParseCommunityPoolLendDepositProposal(
|
|
cdc codec.JSONCodec,
|
|
proposalFile string,
|
|
) (types.CommunityPoolLendDepositProposal, error) {
|
|
proposal := types.CommunityPoolLendDepositProposal{}
|
|
contents, err := os.ReadFile(proposalFile)
|
|
if err != nil {
|
|
return proposal, err
|
|
}
|
|
|
|
err = cdc.UnmarshalJSON(contents, &proposal)
|
|
return proposal, err
|
|
}
|
|
|
|
// ParseCommunityPoolLendWithdrawProposal reads a JSON file and parses it to a CommunityPoolLendWithdrawProposal
|
|
func ParseCommunityPoolLendWithdrawProposal(
|
|
cdc codec.JSONCodec,
|
|
proposalFile string,
|
|
) (types.CommunityPoolLendWithdrawProposal, error) {
|
|
proposal := types.CommunityPoolLendWithdrawProposal{}
|
|
contents, err := os.ReadFile(proposalFile)
|
|
if err != nil {
|
|
return proposal, err
|
|
}
|
|
|
|
err = cdc.UnmarshalJSON(contents, &proposal)
|
|
return proposal, err
|
|
}
|