mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-11-10 18:15:19 +00:00
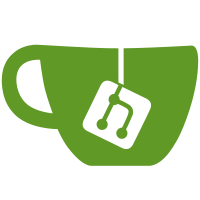
* add kvtool as a subrepo * use kvtool instead of hardcoded configurations * encapsulate chain port numbers * refactor query clients * include ibc in test suite * track & create accounts per chain * setup test suite env & toggle for IBC tests * support different denoms on different chains * add E2E_SKIP_SHUTDOWN flag * test ibc transfer of funds! * include submodules in necessary github checkout action * update readme w/ details about e2e git submodules
55 lines
1.5 KiB
Go
55 lines
1.5 KiB
Go
package testutil
|
|
|
|
import (
|
|
"fmt"
|
|
"os"
|
|
"strconv"
|
|
|
|
"github.com/subosito/gotenv"
|
|
)
|
|
|
|
func init() {
|
|
// read the .env file, if present
|
|
gotenv.Load()
|
|
}
|
|
|
|
type SuiteConfig struct {
|
|
// A funded account used to fnd all other accounts.
|
|
FundedAccountMnemonic string
|
|
// Whether or not to start an IBC chain. Use `suite.SkipIfIbcDisabled()` in IBC tests in IBC tests.
|
|
IncludeIbcTests bool
|
|
// When true, the chains will remain running after tests complete (pass or fail)
|
|
SkipShutdown bool
|
|
}
|
|
|
|
func ParseSuiteConfig() SuiteConfig {
|
|
// this mnemonic is expected to be a funded account that can seed the funds for all
|
|
// new accounts created during tests. it will be available under Accounts["whale"]
|
|
fundedAccountMnemonic := os.Getenv("E2E_KAVA_FUNDED_ACCOUNT_MNEMONIC")
|
|
if fundedAccountMnemonic == "" {
|
|
panic("no E2E_KAVA_FUNDED_ACCOUNT_MNEMONIC provided")
|
|
}
|
|
var skipShutdown bool
|
|
skipShutdownEnv := os.Getenv("E2E_SKIP_SHUTDOWN")
|
|
if skipShutdownEnv != "" {
|
|
skipShutdown = mustParseBool("E2E_SKIP_SHUTDOWN")
|
|
}
|
|
return SuiteConfig{
|
|
FundedAccountMnemonic: fundedAccountMnemonic,
|
|
IncludeIbcTests: mustParseBool("E2E_INCLUDE_IBC_TESTS"),
|
|
SkipShutdown: skipShutdown,
|
|
}
|
|
}
|
|
|
|
func mustParseBool(name string) bool {
|
|
envValue := os.Getenv(name)
|
|
if envValue == "" {
|
|
panic(fmt.Sprintf("%s is unset but expected a bool", name))
|
|
}
|
|
value, err := strconv.ParseBool(envValue)
|
|
if err != nil {
|
|
panic(fmt.Sprintf("%s (%s) cannot be parsed to a bool: %s", name, envValue, err))
|
|
}
|
|
return value
|
|
}
|