mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-11-10 10:05:18 +00:00
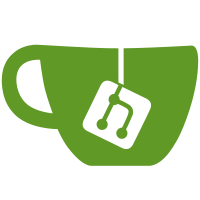
* add eip712 ante * minor cleanup * eip712 integration test with bridge conversion * fix issues * update bridge module * merge bridge module convert logic * update eip712 tests & update deps * remove v17 migrations * remove v17 migrations * fix genesis test * fix erc20 to coin tx * remove eth check * clean up imports * remove * fix evmutil cli * remove bridge comments * address feedback * rename mint method * add transfer checks for locking & unlocking funds * fix gas * increase gas even more * fix amount check Co-authored-by: Ruaridh <rhuairahrighairidh@users.noreply.github.com> Co-authored-by: Ruaridh <rhuairahrighairidh@users.noreply.github.com>
191 lines
6.7 KiB
Go
191 lines
6.7 KiB
Go
package ante
|
|
|
|
import (
|
|
"fmt"
|
|
"runtime/debug"
|
|
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
sdkerrors "github.com/cosmos/cosmos-sdk/types/errors"
|
|
authante "github.com/cosmos/cosmos-sdk/x/auth/ante"
|
|
authsigning "github.com/cosmos/cosmos-sdk/x/auth/signing"
|
|
vesting "github.com/cosmos/cosmos-sdk/x/auth/vesting/types"
|
|
ibcante "github.com/cosmos/ibc-go/v3/modules/core/ante"
|
|
ibckeeper "github.com/cosmos/ibc-go/v3/modules/core/keeper"
|
|
tmlog "github.com/tendermint/tendermint/libs/log"
|
|
evmante "github.com/tharsis/ethermint/app/ante"
|
|
evmtypes "github.com/tharsis/ethermint/x/evm/types"
|
|
)
|
|
|
|
// HandlerOptions extend the SDK's AnteHandler options by requiring the IBC
|
|
// channel keeper, EVM Keeper and Fee Market Keeper.
|
|
type HandlerOptions struct {
|
|
AccountKeeper evmtypes.AccountKeeper
|
|
BankKeeper evmtypes.BankKeeper
|
|
IBCKeeper *ibckeeper.Keeper
|
|
EvmKeeper evmante.EVMKeeper
|
|
FeegrantKeeper authante.FeegrantKeeper
|
|
SignModeHandler authsigning.SignModeHandler
|
|
SigGasConsumer authante.SignatureVerificationGasConsumer
|
|
FeeMarketKeeper evmtypes.FeeMarketKeeper
|
|
MaxTxGasWanted uint64
|
|
AddressFetchers []AddressFetcher
|
|
}
|
|
|
|
func (options HandlerOptions) Validate() error {
|
|
if options.AccountKeeper == nil {
|
|
return sdkerrors.Wrap(sdkerrors.ErrLogic, "account keeper is required for AnteHandler")
|
|
}
|
|
if options.BankKeeper == nil {
|
|
return sdkerrors.Wrap(sdkerrors.ErrLogic, "bank keeper is required for AnteHandler")
|
|
}
|
|
if options.SignModeHandler == nil {
|
|
return sdkerrors.Wrap(sdkerrors.ErrLogic, "sign mode handler is required for ante builder")
|
|
}
|
|
if options.EvmKeeper == nil {
|
|
return sdkerrors.Wrap(sdkerrors.ErrLogic, "evm keeper is required for AnteHandler")
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// cosmosHandlerOptions extends HandlerOptions to provide some Cosmos specific configurations
|
|
type cosmosHandlerOptions struct {
|
|
HandlerOptions
|
|
isEIP712 bool
|
|
}
|
|
|
|
// NewAnteHandler returns an 'AnteHandler' that will run actions before a tx is sent to a module's handler.
|
|
func NewAnteHandler(options HandlerOptions) (sdk.AnteHandler, error) {
|
|
if err := options.Validate(); err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return func(
|
|
ctx sdk.Context, tx sdk.Tx, sim bool,
|
|
) (newCtx sdk.Context, err error) {
|
|
var anteHandler sdk.AnteHandler
|
|
|
|
defer Recover(ctx.Logger(), &err)
|
|
|
|
txWithExtensions, ok := tx.(authante.HasExtensionOptionsTx)
|
|
if ok {
|
|
opts := txWithExtensions.GetExtensionOptions()
|
|
if len(opts) > 1 {
|
|
return ctx, sdkerrors.Wrap(
|
|
sdkerrors.ErrInvalidRequest,
|
|
"rejecting tx with more than 1 extension option",
|
|
)
|
|
}
|
|
|
|
if len(opts) == 1 {
|
|
switch typeURL := opts[0].GetTypeUrl(); typeURL {
|
|
case "/ethermint.evm.v1.ExtensionOptionsEthereumTx":
|
|
// handle as *evmtypes.MsgEthereumTx
|
|
anteHandler = newEthAnteHandler(options)
|
|
case "/ethermint.types.v1.ExtensionOptionsWeb3Tx":
|
|
// handle as normal Cosmos SDK tx, except signature is checked for EIP712 representation
|
|
anteHandler = newCosmosAnteHandler(cosmosHandlerOptions{
|
|
HandlerOptions: options,
|
|
isEIP712: true,
|
|
})
|
|
default:
|
|
return ctx, sdkerrors.Wrapf(
|
|
sdkerrors.ErrUnknownExtensionOptions,
|
|
"rejecting tx with unsupported extension option: %s", typeURL,
|
|
)
|
|
}
|
|
|
|
return anteHandler(ctx, tx, sim)
|
|
}
|
|
}
|
|
|
|
// handle as totally normal Cosmos SDK tx
|
|
switch tx.(type) {
|
|
case sdk.Tx:
|
|
anteHandler = newCosmosAnteHandler(cosmosHandlerOptions{
|
|
HandlerOptions: options,
|
|
isEIP712: false,
|
|
})
|
|
default:
|
|
return ctx, sdkerrors.Wrapf(sdkerrors.ErrUnknownRequest, "invalid transaction type: %T", tx)
|
|
}
|
|
|
|
return anteHandler(ctx, tx, sim)
|
|
}, nil
|
|
}
|
|
|
|
func newCosmosAnteHandler(options cosmosHandlerOptions) sdk.AnteHandler {
|
|
decorators := []sdk.AnteDecorator{}
|
|
|
|
decorators = append(decorators,
|
|
evmante.RejectMessagesDecorator{}, // reject MsgEthereumTxs
|
|
authante.NewSetUpContextDecorator(), // second decorator. SetUpContext must be called before other decorators
|
|
)
|
|
|
|
if !options.isEIP712 {
|
|
decorators = append(decorators, authante.NewRejectExtensionOptionsDecorator())
|
|
}
|
|
|
|
if len(options.AddressFetchers) > 0 {
|
|
decorators = append(decorators, NewAuthenticatedMempoolDecorator(options.AddressFetchers...))
|
|
}
|
|
|
|
var sigVerification sdk.AnteDecorator = authante.NewSigVerificationDecorator(options.AccountKeeper, options.SignModeHandler)
|
|
if options.isEIP712 {
|
|
sigVerification = evmante.NewEip712SigVerificationDecorator(options.AccountKeeper, options.SignModeHandler, options.EvmKeeper)
|
|
}
|
|
|
|
decorators = append(decorators,
|
|
NewEvmMinGasFilter(options.EvmKeeper), // filter out evm denom from min-gas-prices
|
|
authante.NewMempoolFeeDecorator(),
|
|
NewVestingAccountDecorator(),
|
|
NewAuthzLimiterDecorator(
|
|
sdk.MsgTypeURL(&evmtypes.MsgEthereumTx{}),
|
|
sdk.MsgTypeURL(&vesting.MsgCreateVestingAccount{}),
|
|
),
|
|
authante.NewValidateBasicDecorator(),
|
|
authante.NewTxTimeoutHeightDecorator(),
|
|
authante.NewValidateMemoDecorator(options.AccountKeeper),
|
|
authante.NewConsumeGasForTxSizeDecorator(options.AccountKeeper),
|
|
authante.NewDeductFeeDecorator(options.AccountKeeper, options.BankKeeper, options.FeegrantKeeper),
|
|
authante.NewSetPubKeyDecorator(options.AccountKeeper), // SetPubKeyDecorator must be called before all signature verification decorators
|
|
authante.NewValidateSigCountDecorator(options.AccountKeeper),
|
|
authante.NewSigGasConsumeDecorator(options.AccountKeeper, options.SigGasConsumer),
|
|
sigVerification,
|
|
authante.NewIncrementSequenceDecorator(options.AccountKeeper), // innermost AnteDecorator
|
|
ibcante.NewAnteDecorator(options.IBCKeeper),
|
|
)
|
|
return sdk.ChainAnteDecorators(decorators...)
|
|
}
|
|
|
|
func newEthAnteHandler(options HandlerOptions) sdk.AnteHandler {
|
|
return sdk.ChainAnteDecorators(
|
|
evmante.NewEthSetUpContextDecorator(options.EvmKeeper), // outermost AnteDecorator. SetUpContext must be called first
|
|
evmante.NewEthMempoolFeeDecorator(options.EvmKeeper), // Check eth effective gas price against minimal-gas-prices
|
|
evmante.NewEthValidateBasicDecorator(options.EvmKeeper),
|
|
evmante.NewEthSigVerificationDecorator(options.EvmKeeper),
|
|
evmante.NewEthAccountVerificationDecorator(options.AccountKeeper, options.BankKeeper, options.EvmKeeper),
|
|
evmante.NewEthGasConsumeDecorator(options.EvmKeeper, options.MaxTxGasWanted),
|
|
evmante.NewCanTransferDecorator(options.EvmKeeper),
|
|
evmante.NewEthIncrementSenderSequenceDecorator(options.AccountKeeper), // innermost AnteDecorator.
|
|
)
|
|
}
|
|
|
|
func Recover(logger tmlog.Logger, err *error) {
|
|
if r := recover(); r != nil {
|
|
*err = sdkerrors.Wrapf(sdkerrors.ErrPanic, "%v", r)
|
|
|
|
if e, ok := r.(error); ok {
|
|
logger.Error(
|
|
"ante handler panicked",
|
|
"error", e,
|
|
"stack trace", string(debug.Stack()),
|
|
)
|
|
} else {
|
|
logger.Error(
|
|
"ante handler panicked",
|
|
"recover", fmt.Sprintf("%v", r),
|
|
)
|
|
}
|
|
}
|
|
}
|