mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-11-10 10:05:18 +00:00
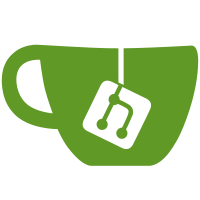
* Update cometbft, cosmos, ethermint, and ibc-go * Replace github.com/tendermint/tendermint by github.com/cometbft/cometbft * Replace github.com/tendermint/tm-db by github.com/cometbft/cometbft-db * Replace gogo/protobuf with cosmos/gogoproto & simapp replacement * Replace cosmos-sdk/simapp/helpers with cosmos-sdk/testutil/sims * Remove no longer used simulations * Replace ibchost with ibcexported See https://github.com/cosmos/ibc-go/blob/v7.2.2/docs/migrations/v6-to-v7.md#ibc-module-constants * Add new consensus params keeper * Add consensus keeper to blockers * Fix keeper and module issues in app.go * Add IsSendEnabledCoins and update SetParams interface changes * Fix protobuf build for cosmos 47 (#1800) * fix cp errors by using -f; fix lint by only linting our proto dir; and use proofs.proto directly from ics23 for ibc-go v7 * run proto-all; commit updated third party deps and swagger changes * regenerate proto files * use correct gocosmos build plugin for buf * re-gen all protobuf files to update paths for new gocosmos plugin * update protoc and buf to latest versions * fix staking keeper issues in app.go * update tally handler for gov changes * chain id fix and flag fixes * update deps for cometbft 47.7 upgrade * remove all module legacy queriers * update stakingKeeper to pointer * Replace ModuleCdc from govv1beta1 to govcodec * remove simulations * abci.LastCommitInfo → abci.CommitInfo * Remove unused code in keys.go * simapp.MakeTestEncodingConfig -> moduletestutil.MakeTestEncodingConfi * Fix chain id issues in tests * Fix remaining unit test issues * Update changelog for upgrade * Fix e2e tests using updated kvtool * Update protonet to v47 compatible genesis * Bump cometbft-db to v0.9.1-kava.1 * Update kvtool * Remove extra changelog * Fix merged rocksdb issues * go mod cleanup * Bump cometbft-db to v9 and go to 1.21 * Bump rocksdb version to v8.10.0 * Update kvtool to latest version * Update gin to v1.9.0 * Use ibctm.ModuleName in app_test * Fallback to genesis chain id instead of client toml * Remove all simulations * Fix cdp migrations issue with v47 * Update dependencies to correct tags --------- Co-authored-by: Nick DeLuca <nickdeluca08@gmail.com>
113 lines
5.4 KiB
Protocol Buffer
113 lines
5.4 KiB
Protocol Buffer
syntax = "proto3";
|
|
|
|
package ibc.applications.fee.v1;
|
|
|
|
option go_package = "github.com/cosmos/ibc-go/v7/modules/apps/29-fee/types";
|
|
|
|
import "gogoproto/gogo.proto";
|
|
import "ibc/applications/fee/v1/fee.proto";
|
|
import "ibc/core/channel/v1/channel.proto";
|
|
|
|
// Msg defines the ICS29 Msg service.
|
|
service Msg {
|
|
// RegisterPayee defines a rpc handler method for MsgRegisterPayee
|
|
// RegisterPayee is called by the relayer on each channelEnd and allows them to set an optional
|
|
// payee to which reverse and timeout relayer packet fees will be paid out. The payee should be registered on
|
|
// the source chain from which packets originate as this is where fee distribution takes place. This function may be
|
|
// called more than once by a relayer, in which case, the latest payee is always used.
|
|
rpc RegisterPayee(MsgRegisterPayee) returns (MsgRegisterPayeeResponse);
|
|
|
|
// RegisterCounterpartyPayee defines a rpc handler method for MsgRegisterCounterpartyPayee
|
|
// RegisterCounterpartyPayee is called by the relayer on each channelEnd and allows them to specify the counterparty
|
|
// payee address before relaying. This ensures they will be properly compensated for forward relaying since
|
|
// the destination chain must include the registered counterparty payee address in the acknowledgement. This function
|
|
// may be called more than once by a relayer, in which case, the latest counterparty payee address is always used.
|
|
rpc RegisterCounterpartyPayee(MsgRegisterCounterpartyPayee) returns (MsgRegisterCounterpartyPayeeResponse);
|
|
|
|
// PayPacketFee defines a rpc handler method for MsgPayPacketFee
|
|
// PayPacketFee is an open callback that may be called by any module/user that wishes to escrow funds in order to
|
|
// incentivize the relaying of the packet at the next sequence
|
|
// NOTE: This method is intended to be used within a multi msg transaction, where the subsequent msg that follows
|
|
// initiates the lifecycle of the incentivized packet
|
|
rpc PayPacketFee(MsgPayPacketFee) returns (MsgPayPacketFeeResponse);
|
|
|
|
// PayPacketFeeAsync defines a rpc handler method for MsgPayPacketFeeAsync
|
|
// PayPacketFeeAsync is an open callback that may be called by any module/user that wishes to escrow funds in order to
|
|
// incentivize the relaying of a known packet (i.e. at a particular sequence)
|
|
rpc PayPacketFeeAsync(MsgPayPacketFeeAsync) returns (MsgPayPacketFeeAsyncResponse);
|
|
}
|
|
|
|
// MsgRegisterPayee defines the request type for the RegisterPayee rpc
|
|
message MsgRegisterPayee {
|
|
option (gogoproto.equal) = false;
|
|
option (gogoproto.goproto_getters) = false;
|
|
|
|
// unique port identifier
|
|
string port_id = 1 [(gogoproto.moretags) = "yaml:\"port_id\""];
|
|
// unique channel identifier
|
|
string channel_id = 2 [(gogoproto.moretags) = "yaml:\"channel_id\""];
|
|
// the relayer address
|
|
string relayer = 3;
|
|
// the payee address
|
|
string payee = 4;
|
|
}
|
|
|
|
// MsgRegisterPayeeResponse defines the response type for the RegisterPayee rpc
|
|
message MsgRegisterPayeeResponse {}
|
|
|
|
// MsgRegisterCounterpartyPayee defines the request type for the RegisterCounterpartyPayee rpc
|
|
message MsgRegisterCounterpartyPayee {
|
|
option (gogoproto.equal) = false;
|
|
option (gogoproto.goproto_getters) = false;
|
|
|
|
// unique port identifier
|
|
string port_id = 1 [(gogoproto.moretags) = "yaml:\"port_id\""];
|
|
// unique channel identifier
|
|
string channel_id = 2 [(gogoproto.moretags) = "yaml:\"channel_id\""];
|
|
// the relayer address
|
|
string relayer = 3;
|
|
// the counterparty payee address
|
|
string counterparty_payee = 4 [(gogoproto.moretags) = "yaml:\"counterparty_payee\""];
|
|
}
|
|
|
|
// MsgRegisterCounterpartyPayeeResponse defines the response type for the RegisterCounterpartyPayee rpc
|
|
message MsgRegisterCounterpartyPayeeResponse {}
|
|
|
|
// MsgPayPacketFee defines the request type for the PayPacketFee rpc
|
|
// This Msg can be used to pay for a packet at the next sequence send & should be combined with the Msg that will be
|
|
// paid for
|
|
message MsgPayPacketFee {
|
|
option (gogoproto.equal) = false;
|
|
option (gogoproto.goproto_getters) = false;
|
|
|
|
// fee encapsulates the recv, ack and timeout fees associated with an IBC packet
|
|
ibc.applications.fee.v1.Fee fee = 1 [(gogoproto.nullable) = false];
|
|
// the source port unique identifier
|
|
string source_port_id = 2 [(gogoproto.moretags) = "yaml:\"source_port_id\""];
|
|
// the source channel unique identifer
|
|
string source_channel_id = 3 [(gogoproto.moretags) = "yaml:\"source_channel_id\""];
|
|
// account address to refund fee if necessary
|
|
string signer = 4;
|
|
// optional list of relayers permitted to the receive packet fees
|
|
repeated string relayers = 5;
|
|
}
|
|
|
|
// MsgPayPacketFeeResponse defines the response type for the PayPacketFee rpc
|
|
message MsgPayPacketFeeResponse {}
|
|
|
|
// MsgPayPacketFeeAsync defines the request type for the PayPacketFeeAsync rpc
|
|
// This Msg can be used to pay for a packet at a specified sequence (instead of the next sequence send)
|
|
message MsgPayPacketFeeAsync {
|
|
option (gogoproto.equal) = false;
|
|
option (gogoproto.goproto_getters) = false;
|
|
|
|
// unique packet identifier comprised of the channel ID, port ID and sequence
|
|
ibc.core.channel.v1.PacketId packet_id = 1
|
|
[(gogoproto.moretags) = "yaml:\"packet_id\"", (gogoproto.nullable) = false];
|
|
// the packet fee associated with a particular IBC packet
|
|
PacketFee packet_fee = 2 [(gogoproto.moretags) = "yaml:\"packet_fee\"", (gogoproto.nullable) = false];
|
|
}
|
|
|
|
// MsgPayPacketFeeAsyncResponse defines the response type for the PayPacketFeeAsync rpc
|
|
message MsgPayPacketFeeAsyncResponse {}
|