mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 22:57:32 +00:00
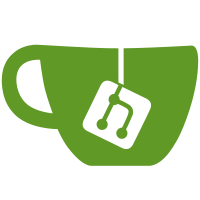
* Committee types (#899) * committee types * refactor to committee interface * include tokencommitee stringer method * add members to BaseCommittee * address revisions * update querier * update querier * fix compilation errors, tests, etc. * Update MsgVote with vote type (#900) * add vote to msg * update querier/rest * update example cli vote msg * remove incorrect comments * address revisions * update handler, stub keeper method * add vote type to vote struct * Committee module keeper logic for token holder governance (#902) * fix keeper/test compilation errors * fix keeper/test compilation errors pt 2 * add setters to committee interface * fix sims compilation errors * fix incentive tests compilation errors * update types, expected keepers * core keeper logic * don't allow bond denom * implement vote tallying * query proposal polling status * update module keepers in app.go * register committee interface * fix failing incentive test * commitee types tests * refactor GetProposalResult by committee types * update invariants * implement most proposal keeper tests * add nulls to custom enums * remove abstain vote type * add test for close proposal * remove outdated TODOs * update ProcessProposals * switch on committee type directly * reintroduce Abstain votes and update vote tallying * don't allow divide by 0 panics * delete unused setters on committee interface * clean up tally methods return values for querier * update enum validation to catch negative ints * reintroduce setters for sims compilation * address revisions * remove commented out test * implement ProcessProposals test * additional revisions * Committee migrations (#909) * add committee v14 legacy types * update migration imports for compile * addRegisterCodec() to committee v14 legacy types * migrate committee genesis state from v14 to v15 * set stability committee permissions properly * fix committee allowed params * migration test, kava-7 sample data * add concrete types to committees (#911) * revisions: migrate + tests * register msgs on legacy codec * Prepare Committee module for migrations (#906) * remove invariants * edits * fix abci test * fix keeper querier tests * add committee interface registration * use codec.Codec * don't allow null vote types * don't allow null tally option * minor spelling fixes * update example cli proposal * fix cli tally query * enable vote abstain from cli * include vote options in cli help text * call CloseProposal from handler * custom enum marshaling * committee: fix failing tests (#921) * fix failing tests * fix: spelling Co-authored-by: rhuairahrighairigh <ruaridh.odonnell@gmail.com> Co-authored-by: Ruaridh <rhuairahrighairidh@users.noreply.github.com> Co-authored-by: Kevin Davis <karzak@users.noreply.github.com>
243 lines
7.6 KiB
Go
243 lines
7.6 KiB
Go
package committee_test
|
|
|
|
import (
|
|
"testing"
|
|
"time"
|
|
|
|
"github.com/stretchr/testify/suite"
|
|
|
|
"github.com/cosmos/cosmos-sdk/codec"
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
"github.com/cosmos/cosmos-sdk/x/gov"
|
|
|
|
abci "github.com/tendermint/tendermint/abci/types"
|
|
|
|
"github.com/kava-labs/kava/app"
|
|
"github.com/kava-labs/kava/x/committee"
|
|
"github.com/kava-labs/kava/x/committee/types"
|
|
)
|
|
|
|
var testTime time.Time = time.Date(1998, time.January, 1, 0, 0, 0, 0, time.UTC)
|
|
|
|
func NewCommitteeGenState(cdc *codec.Codec, gs committee.GenesisState) app.GenesisState {
|
|
return app.GenesisState{committee.ModuleName: cdc.MustMarshalJSON(gs)}
|
|
}
|
|
|
|
type ProposalHandlerTestSuite struct {
|
|
suite.Suite
|
|
|
|
keeper committee.Keeper
|
|
app app.TestApp
|
|
ctx sdk.Context
|
|
|
|
addresses []sdk.AccAddress
|
|
testGenesis committee.GenesisState
|
|
}
|
|
|
|
func (suite *ProposalHandlerTestSuite) SetupTest() {
|
|
_, suite.addresses = app.GeneratePrivKeyAddressPairs(5)
|
|
suite.testGenesis = committee.NewGenesisState(
|
|
2,
|
|
[]committee.Committee{
|
|
committee.MemberCommittee{
|
|
BaseCommittee: committee.BaseCommittee{
|
|
ID: 1,
|
|
Description: "This committee is for testing.",
|
|
Members: suite.addresses[:3],
|
|
Permissions: []types.Permission{types.GodPermission{}},
|
|
VoteThreshold: d("0.667"),
|
|
ProposalDuration: time.Hour * 24 * 7,
|
|
TallyOption: types.FirstPastThePost,
|
|
},
|
|
},
|
|
committee.MemberCommittee{
|
|
BaseCommittee: committee.BaseCommittee{
|
|
ID: 2,
|
|
Members: suite.addresses[2:],
|
|
Permissions: nil,
|
|
VoteThreshold: d("0.667"),
|
|
ProposalDuration: time.Hour * 24 * 7,
|
|
TallyOption: types.FirstPastThePost,
|
|
},
|
|
},
|
|
},
|
|
[]committee.Proposal{
|
|
{ID: 1, CommitteeID: 1, PubProposal: gov.NewTextProposal("A Title", "A description of this proposal."), Deadline: testTime.Add(7 * 24 * time.Hour)},
|
|
},
|
|
[]committee.Vote{
|
|
{ProposalID: 1, Voter: suite.addresses[0], VoteType: types.Yes},
|
|
},
|
|
)
|
|
}
|
|
|
|
func (suite *ProposalHandlerTestSuite) TestProposalHandler_ChangeCommittee() {
|
|
testCases := []struct {
|
|
name string
|
|
proposal committee.CommitteeChangeProposal
|
|
expectPass bool
|
|
}{
|
|
{
|
|
name: "add new",
|
|
proposal: committee.NewCommitteeChangeProposal(
|
|
"A Title",
|
|
"A proposal description.",
|
|
committee.MemberCommittee{
|
|
BaseCommittee: committee.BaseCommittee{
|
|
ID: 34,
|
|
Members: suite.addresses[:1],
|
|
VoteThreshold: d("1"),
|
|
ProposalDuration: time.Hour * 24,
|
|
TallyOption: types.FirstPastThePost,
|
|
},
|
|
},
|
|
),
|
|
expectPass: true,
|
|
},
|
|
{
|
|
name: "update",
|
|
proposal: committee.NewCommitteeChangeProposal(
|
|
"A Title",
|
|
"A proposal description.",
|
|
committee.MemberCommittee{
|
|
BaseCommittee: committee.BaseCommittee{
|
|
ID: suite.testGenesis.Committees[0].GetID(),
|
|
Members: suite.addresses, // add new members
|
|
Permissions: suite.testGenesis.Committees[0].GetPermissions(),
|
|
VoteThreshold: suite.testGenesis.Committees[0].GetVoteThreshold(),
|
|
ProposalDuration: suite.testGenesis.Committees[0].GetProposalDuration(),
|
|
TallyOption: types.FirstPastThePost,
|
|
Type: types.MemberCommitteeType,
|
|
},
|
|
},
|
|
),
|
|
expectPass: true,
|
|
},
|
|
{
|
|
name: "invalid title",
|
|
proposal: committee.NewCommitteeChangeProposal(
|
|
"A Title That Is Much Too Long And Really Quite Unreasonable Given That It Is Trying To Fulfill The Roll Of An Acceptable Governance Proposal Title That Should Succinctly Communicate The Goal And Contents Of The Proposed Proposal To All Parties Involved",
|
|
"A proposal description.",
|
|
suite.testGenesis.Committees[0],
|
|
),
|
|
expectPass: false,
|
|
},
|
|
{
|
|
name: "invalid committee",
|
|
proposal: committee.NewCommitteeChangeProposal(
|
|
"A Title",
|
|
"A proposal description.",
|
|
committee.MemberCommittee{
|
|
BaseCommittee: committee.BaseCommittee{
|
|
ID: suite.testGenesis.Committees[0].GetID(),
|
|
Members: append(suite.addresses, suite.addresses[0]), // duplicate address
|
|
Permissions: suite.testGenesis.Committees[0].GetPermissions(),
|
|
VoteThreshold: suite.testGenesis.Committees[0].GetVoteThreshold(),
|
|
ProposalDuration: suite.testGenesis.Committees[0].GetProposalDuration(),
|
|
},
|
|
},
|
|
),
|
|
expectPass: false,
|
|
},
|
|
}
|
|
for _, tc := range testCases {
|
|
suite.Run(tc.name, func() {
|
|
// Setup
|
|
suite.app = app.NewTestApp()
|
|
suite.keeper = suite.app.GetCommitteeKeeper()
|
|
suite.app = suite.app.InitializeFromGenesisStates(
|
|
NewCommitteeGenState(suite.app.Codec(), suite.testGenesis),
|
|
)
|
|
suite.ctx = suite.app.NewContext(true, abci.Header{Height: 1, Time: testTime})
|
|
handler := committee.NewProposalHandler(suite.keeper)
|
|
|
|
oldProposals := suite.keeper.GetProposalsByCommittee(suite.ctx, tc.proposal.NewCommittee.GetID())
|
|
|
|
// Run
|
|
err := handler(suite.ctx, tc.proposal)
|
|
|
|
// Check
|
|
if tc.expectPass {
|
|
suite.NoError(err)
|
|
// check committee is accurate
|
|
actualCom, found := suite.keeper.GetCommittee(suite.ctx, tc.proposal.NewCommittee.GetID())
|
|
suite.True(found)
|
|
suite.Equal(tc.proposal.NewCommittee, actualCom)
|
|
|
|
// check proposals and votes for this committee have been removed
|
|
suite.Empty(suite.keeper.GetProposalsByCommittee(suite.ctx, tc.proposal.NewCommittee.GetID()))
|
|
for _, p := range oldProposals {
|
|
suite.Empty(suite.keeper.GetVotesByProposal(suite.ctx, p.ID))
|
|
}
|
|
} else {
|
|
suite.Error(err)
|
|
suite.Equal(suite.testGenesis, committee.ExportGenesis(suite.ctx, suite.keeper))
|
|
}
|
|
})
|
|
}
|
|
}
|
|
|
|
func (suite *ProposalHandlerTestSuite) TestProposalHandler_DeleteCommittee() {
|
|
testCases := []struct {
|
|
name string
|
|
proposal committee.CommitteeDeleteProposal
|
|
expectPass bool
|
|
}{
|
|
{
|
|
name: "normal",
|
|
proposal: committee.NewCommitteeDeleteProposal(
|
|
"A Title",
|
|
"A proposal description.",
|
|
suite.testGenesis.Committees[0].GetID(),
|
|
),
|
|
expectPass: true,
|
|
},
|
|
{
|
|
name: "invalid title",
|
|
proposal: committee.NewCommitteeDeleteProposal(
|
|
"A Title That Is Much Too Long And Really Quite Unreasonable Given That It Is Trying To Fulfill The Roll Of An Acceptable Governance Proposal Title That Should Succinctly Communicate The Goal And Contents Of The Proposed Proposal To All Parties Involved",
|
|
"A proposal description.",
|
|
suite.testGenesis.Committees[1].GetID(),
|
|
),
|
|
expectPass: false,
|
|
},
|
|
}
|
|
for _, tc := range testCases {
|
|
suite.Run(tc.name, func() {
|
|
// Setup
|
|
suite.app = app.NewTestApp()
|
|
suite.keeper = suite.app.GetCommitteeKeeper()
|
|
suite.app = suite.app.InitializeFromGenesisStates(
|
|
NewCommitteeGenState(suite.app.Codec(), suite.testGenesis),
|
|
)
|
|
suite.ctx = suite.app.NewContext(true, abci.Header{Height: 1, Time: testTime})
|
|
handler := committee.NewProposalHandler(suite.keeper)
|
|
|
|
oldProposals := suite.keeper.GetProposalsByCommittee(suite.ctx, tc.proposal.CommitteeID)
|
|
|
|
// Run
|
|
err := handler(suite.ctx, tc.proposal)
|
|
|
|
// Check
|
|
if tc.expectPass {
|
|
suite.NoError(err)
|
|
// check committee has been removed
|
|
_, found := suite.keeper.GetCommittee(suite.ctx, tc.proposal.CommitteeID)
|
|
suite.False(found)
|
|
|
|
// check proposals and votes for this committee have been removed
|
|
suite.Empty(suite.keeper.GetProposalsByCommittee(suite.ctx, tc.proposal.CommitteeID))
|
|
for _, p := range oldProposals {
|
|
suite.Empty(suite.keeper.GetVotesByProposal(suite.ctx, p.ID))
|
|
}
|
|
} else {
|
|
suite.Error(err)
|
|
suite.Equal(suite.testGenesis, committee.ExportGenesis(suite.ctx, suite.keeper))
|
|
}
|
|
})
|
|
}
|
|
}
|
|
|
|
func TestProposalHandlerTestSuite(t *testing.T) {
|
|
suite.Run(t, new(ProposalHandlerTestSuite))
|
|
}
|