mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-11-10 10:05:18 +00:00
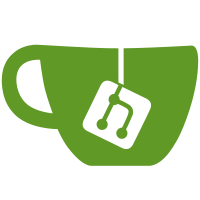
* split up payout.go file * extract genesis builders to new testutil package * move claim integration tests out of keeper * convert claim integration tests to handler tests * combine claim usdx minting keeper methods * combine hard claim keeper methods * combine delegator claim keeper methods * add multiply coins helper method * rename file to better match contents * add basic claiming unit tests * add claiming subset of delegator reward denoms * refactor msg tests * add msg ValidateBasic tests * connect swap hooks into keeper methods * tidy up delegator handler tests * add swap claiming msgs and keeper method * add swap claiming to client * add subset claiming to other msg types * split up handler test file * connect up subset claiming for swap * make multiplier name validation more strict * fix: struct tag typo in swap incentives * connect up subset claiming for hard * connect up subset claiming for delegator * fix: register cli tx routes for swp claiming * fix claim amount in claim event * fix token name in cli help docs * remove unused field in msg tests * tidy up swap and delegator handler tests * refactor hard handler tests * refactor usdx handler tests * remove unused constant Co-authored-by: karzak <kjydavis3@gmail.com>
196 lines
6.1 KiB
Go
196 lines
6.1 KiB
Go
package incentive_test
|
|
|
|
import (
|
|
"time"
|
|
|
|
"github.com/cosmos/cosmos-sdk/x/auth/vesting"
|
|
|
|
"github.com/kava-labs/kava/x/incentive/types"
|
|
)
|
|
|
|
func (suite *HandlerTestSuite) TestPayoutHardClaim() {
|
|
userAddr, receiverAddr := suite.addrs[0], suite.addrs[1]
|
|
|
|
authBulder := suite.authBuilder().
|
|
WithSimpleAccount(userAddr, cs(c("bnb", 1e12))).
|
|
WithSimpleAccount(receiverAddr, nil)
|
|
|
|
incentBuilder := suite.incentiveBuilder().
|
|
WithSimpleSupplyRewardPeriod("bnb", cs(c("hard", 1e6))).
|
|
WithSimpleBorrowRewardPeriod("bnb", cs(c("hard", 1e6)))
|
|
|
|
suite.SetupWithGenState(authBulder, incentBuilder)
|
|
|
|
// create a deposit and borrow
|
|
suite.NoError(suite.DeliverHardMsgDeposit(userAddr, cs(c("bnb", 1e11))))
|
|
suite.NoError(suite.DeliverHardMsgBorrow(userAddr, cs(c("bnb", 1e10))))
|
|
|
|
// accumulate some rewards
|
|
suite.NextBlockAfter(7 * time.Second)
|
|
|
|
preClaimBal := suite.GetBalance(userAddr)
|
|
|
|
// Check rewards cannot be claimed by vvesting claim msgs
|
|
err := suite.DeliverIncentiveMsg(
|
|
types.NewMsgClaimHardRewardVVesting(userAddr, receiverAddr, "large", nil),
|
|
)
|
|
suite.ErrorIs(err, types.ErrInvalidAccountType)
|
|
|
|
// Claim a single denom
|
|
err = suite.DeliverIncentiveMsg(
|
|
types.NewMsgClaimHardReward(userAddr, "large", nil),
|
|
)
|
|
suite.NoError(err)
|
|
|
|
// Check rewards were paid out
|
|
expectedRewards := cs(c("hard", 2*7*1e6))
|
|
suite.BalanceEquals(userAddr, preClaimBal.Add(expectedRewards...))
|
|
|
|
suite.VestingPeriodsEqual(userAddr, vesting.Periods{
|
|
{Length: 33004793, Amount: expectedRewards},
|
|
})
|
|
// Check that claimed coins have been removed from a claim's reward
|
|
suite.HardRewardEquals(userAddr, nil)
|
|
}
|
|
|
|
func (suite *HandlerTestSuite) TestPayoutHardClaimSingleDenom() {
|
|
userAddr := suite.addrs[0]
|
|
|
|
authBulder := suite.authBuilder().
|
|
WithSimpleAccount(userAddr, cs(c("bnb", 1e12)))
|
|
|
|
incentBuilder := suite.incentiveBuilder().
|
|
WithSimpleSupplyRewardPeriod("bnb", cs(c("hard", 1e6), c("swap", 1e6))).
|
|
WithSimpleBorrowRewardPeriod("bnb", cs(c("hard", 1e6), c("swap", 1e6)))
|
|
|
|
suite.SetupWithGenState(authBulder, incentBuilder)
|
|
|
|
// create a deposit and borrow
|
|
suite.NoError(suite.DeliverHardMsgDeposit(userAddr, cs(c("bnb", 1e11))))
|
|
suite.NoError(suite.DeliverHardMsgBorrow(userAddr, cs(c("bnb", 1e10))))
|
|
|
|
// accumulate some rewards
|
|
suite.NextBlockAfter(7 * time.Second)
|
|
|
|
preClaimBal := suite.GetBalance(userAddr)
|
|
|
|
// Check rewards cannot be claimed by vvesting claim msgs
|
|
err := suite.DeliverIncentiveMsg(
|
|
types.NewMsgClaimHardRewardVVesting(userAddr, suite.addrs[1], "large", []string{"swap"}),
|
|
)
|
|
suite.ErrorIs(err, types.ErrInvalidAccountType)
|
|
|
|
// Claim rewards
|
|
err = suite.DeliverIncentiveMsg(
|
|
types.NewMsgClaimHardReward(userAddr, "large", []string{"swap"}),
|
|
)
|
|
suite.NoError(err)
|
|
|
|
// Check rewards were paid out
|
|
expectedRewards := c("swap", 2*7*1e6)
|
|
suite.BalanceEquals(userAddr, preClaimBal.Add(expectedRewards))
|
|
|
|
suite.VestingPeriodsEqual(userAddr, vesting.Periods{
|
|
{Length: 33004793, Amount: cs(expectedRewards)},
|
|
})
|
|
|
|
// Check that claimed coins have been removed from a claim's reward
|
|
suite.HardRewardEquals(userAddr, cs(c("hard", 2*7*1e6)))
|
|
}
|
|
|
|
func (suite *HandlerTestSuite) TestPayoutHardClaimVVesting() {
|
|
valAddr, receiverAddr := suite.addrs[0], suite.addrs[1]
|
|
|
|
vva := suite.NewValidatorVestingAccountWithBalance(valAddr, cs(c("bnb", 1e12)))
|
|
|
|
authBulder := suite.authBuilder().
|
|
WithAccounts(vva).
|
|
WithSimpleAccount(receiverAddr, nil)
|
|
|
|
incentBuilder := suite.incentiveBuilder().
|
|
WithSimpleSupplyRewardPeriod("bnb", cs(c("hard", 1e6))).
|
|
WithSimpleBorrowRewardPeriod("bnb", cs(c("hard", 1e6)))
|
|
|
|
suite.SetupWithGenState(authBulder, incentBuilder)
|
|
|
|
// create a deposit and borrow
|
|
suite.NoError(suite.DeliverHardMsgDeposit(valAddr, cs(c("bnb", 1e11))))
|
|
suite.NoError(suite.DeliverHardMsgBorrow(valAddr, cs(c("bnb", 1e10))))
|
|
|
|
// accumulate some rewards
|
|
suite.NextBlockAfter(7 * time.Second)
|
|
|
|
preClaimBal := suite.GetBalance(receiverAddr)
|
|
|
|
// Check rewards cannot be claimed by normal claim msgs
|
|
err := suite.DeliverIncentiveMsg(
|
|
types.NewMsgClaimHardReward(valAddr, "large", nil),
|
|
)
|
|
suite.ErrorIs(err, types.ErrInvalidAccountType)
|
|
|
|
// Claim rewards
|
|
err = suite.DeliverIncentiveMsg(
|
|
types.NewMsgClaimHardRewardVVesting(valAddr, receiverAddr, "large", nil),
|
|
)
|
|
suite.NoError(err)
|
|
|
|
// Check rewards were paid out
|
|
expectedRewards := c("hard", 2*7*1e6)
|
|
suite.BalanceEquals(receiverAddr, preClaimBal.Add(expectedRewards))
|
|
|
|
suite.VestingPeriodsEqual(receiverAddr, vesting.Periods{
|
|
{Length: 33004793, Amount: cs(expectedRewards)},
|
|
})
|
|
|
|
// Check that each claim reward coin's amount has been reset to 0
|
|
suite.HardRewardEquals(valAddr, nil)
|
|
}
|
|
|
|
func (suite *HandlerTestSuite) TestPayoutHardClaimVVestingSingleDenom() {
|
|
valAddr, receiverAddr := suite.addrs[0], suite.addrs[1]
|
|
|
|
vva := suite.NewValidatorVestingAccountWithBalance(valAddr, cs(c("bnb", 1e12)))
|
|
|
|
authBulder := suite.authBuilder().
|
|
WithAccounts(vva).
|
|
WithSimpleAccount(receiverAddr, nil)
|
|
|
|
incentBuilder := suite.incentiveBuilder().
|
|
WithSimpleSupplyRewardPeriod("bnb", cs(c("hard", 1e6), c("swap", 1e6))).
|
|
WithSimpleBorrowRewardPeriod("bnb", cs(c("hard", 1e6), c("swap", 1e6)))
|
|
|
|
suite.SetupWithGenState(authBulder, incentBuilder)
|
|
|
|
// create a deposit and borrow
|
|
suite.NoError(suite.DeliverHardMsgDeposit(valAddr, cs(c("bnb", 1e11))))
|
|
suite.NoError(suite.DeliverHardMsgBorrow(valAddr, cs(c("bnb", 1e10))))
|
|
|
|
// accumulate some rewards
|
|
suite.NextBlockAfter(7 * time.Second)
|
|
|
|
preClaimBal := suite.GetBalance(receiverAddr)
|
|
|
|
// Check rewards cannot be claimed by normal claim msgs
|
|
err := suite.DeliverIncentiveMsg(
|
|
types.NewMsgClaimHardReward(valAddr, "large", []string{"swap"}),
|
|
)
|
|
suite.ErrorIs(err, types.ErrInvalidAccountType)
|
|
|
|
// Claim rewards
|
|
err = suite.DeliverIncentiveMsg(
|
|
types.NewMsgClaimHardRewardVVesting(valAddr, receiverAddr, "large", []string{"swap"}),
|
|
)
|
|
suite.NoError(err)
|
|
|
|
// Check rewards were paid out
|
|
expectedRewards := c("swap", 2*7*1e6)
|
|
suite.BalanceEquals(receiverAddr, preClaimBal.Add(expectedRewards))
|
|
|
|
suite.VestingPeriodsEqual(receiverAddr, vesting.Periods{
|
|
{Length: 33004793, Amount: cs(expectedRewards)},
|
|
})
|
|
|
|
// Check that claimed coins have been removed from a claim's reward
|
|
suite.HardRewardEquals(valAddr, cs(c("hard", 2*7*1e6)))
|
|
}
|