mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 14:47:31 +00:00
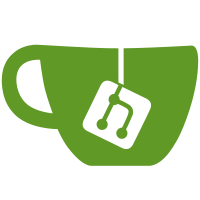
* Committee types (#899) * committee types * refactor to committee interface * include tokencommitee stringer method * add members to BaseCommittee * address revisions * update querier * update querier * fix compilation errors, tests, etc. * Update MsgVote with vote type (#900) * add vote to msg * update querier/rest * update example cli vote msg * remove incorrect comments * address revisions * update handler, stub keeper method * add vote type to vote struct * Committee module keeper logic for token holder governance (#902) * fix keeper/test compilation errors * fix keeper/test compilation errors pt 2 * add setters to committee interface * fix sims compilation errors * fix incentive tests compilation errors * update types, expected keepers * core keeper logic * don't allow bond denom * implement vote tallying * query proposal polling status * update module keepers in app.go * register committee interface * fix failing incentive test * commitee types tests * refactor GetProposalResult by committee types * update invariants * implement most proposal keeper tests * add nulls to custom enums * remove abstain vote type * add test for close proposal * remove outdated TODOs * update ProcessProposals * switch on committee type directly * reintroduce Abstain votes and update vote tallying * don't allow divide by 0 panics * delete unused setters on committee interface * clean up tally methods return values for querier * update enum validation to catch negative ints * reintroduce setters for sims compilation * address revisions * remove commented out test * implement ProcessProposals test * additional revisions * Committee migrations (#909) * add committee v14 legacy types * update migration imports for compile * addRegisterCodec() to committee v14 legacy types * migrate committee genesis state from v14 to v15 * set stability committee permissions properly * fix committee allowed params * migration test, kava-7 sample data * add concrete types to committees (#911) * revisions: migrate + tests * register msgs on legacy codec * Prepare Committee module for migrations (#906) * remove invariants * edits * fix abci test * fix keeper querier tests * add committee interface registration * use codec.Codec * don't allow null vote types * don't allow null tally option * minor spelling fixes * update example cli proposal * fix cli tally query * enable vote abstain from cli * include vote options in cli help text * call CloseProposal from handler * custom enum marshaling * committee: fix failing tests (#921) * fix failing tests * fix: spelling Co-authored-by: rhuairahrighairigh <ruaridh.odonnell@gmail.com> Co-authored-by: Ruaridh <rhuairahrighairidh@users.noreply.github.com> Co-authored-by: Kevin Davis <karzak@users.noreply.github.com>
230 lines
8.1 KiB
Go
230 lines
8.1 KiB
Go
package committee_test
|
|
|
|
import (
|
|
"testing"
|
|
"time"
|
|
|
|
"github.com/stretchr/testify/suite"
|
|
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
"github.com/cosmos/cosmos-sdk/x/gov"
|
|
"github.com/cosmos/cosmos-sdk/x/params"
|
|
"github.com/cosmos/cosmos-sdk/x/upgrade"
|
|
|
|
abci "github.com/tendermint/tendermint/abci/types"
|
|
|
|
"github.com/kava-labs/kava/app"
|
|
"github.com/kava-labs/kava/x/cdp"
|
|
cdptypes "github.com/kava-labs/kava/x/cdp/types"
|
|
"github.com/kava-labs/kava/x/committee"
|
|
"github.com/kava-labs/kava/x/committee/types"
|
|
)
|
|
|
|
type ModuleTestSuite struct {
|
|
suite.Suite
|
|
|
|
keeper committee.Keeper
|
|
app app.TestApp
|
|
ctx sdk.Context
|
|
|
|
addresses []sdk.AccAddress
|
|
}
|
|
|
|
func (suite *ModuleTestSuite) SetupTest() {
|
|
suite.app = app.NewTestApp()
|
|
suite.keeper = suite.app.GetCommitteeKeeper()
|
|
suite.ctx = suite.app.NewContext(true, abci.Header{})
|
|
_, suite.addresses = app.GeneratePrivKeyAddressPairs(5)
|
|
}
|
|
|
|
func (suite *ModuleTestSuite) TestBeginBlock_ClosesExpired() {
|
|
suite.app.InitializeFromGenesisStates()
|
|
|
|
memberCom := committee.MemberCommittee{
|
|
BaseCommittee: committee.BaseCommittee{
|
|
ID: 12,
|
|
Members: suite.addresses[:2],
|
|
Permissions: []committee.Permission{committee.GodPermission{}},
|
|
VoteThreshold: d("0.8"),
|
|
ProposalDuration: time.Hour * 24 * 7,
|
|
},
|
|
}
|
|
suite.keeper.SetCommittee(suite.ctx, memberCom)
|
|
|
|
pprop1 := gov.NewTextProposal("Title 1", "A description of this proposal.")
|
|
id1, err := suite.keeper.SubmitProposal(suite.ctx, memberCom.Members[0], memberCom.ID, pprop1)
|
|
suite.NoError(err)
|
|
|
|
oneHrLaterCtx := suite.ctx.WithBlockTime(suite.ctx.BlockTime().Add(time.Hour))
|
|
pprop2 := gov.NewTextProposal("Title 2", "A description of this proposal.")
|
|
id2, err := suite.keeper.SubmitProposal(oneHrLaterCtx, memberCom.Members[0], memberCom.ID, pprop2)
|
|
suite.NoError(err)
|
|
|
|
// Run BeginBlocker
|
|
proposalDurationLaterCtx := suite.ctx.WithBlockTime(suite.ctx.BlockTime().Add(memberCom.ProposalDuration))
|
|
suite.NotPanics(func() {
|
|
committee.BeginBlocker(proposalDurationLaterCtx, abci.RequestBeginBlock{}, suite.keeper)
|
|
})
|
|
|
|
// Check expired proposals are gone
|
|
_, found := suite.keeper.GetProposal(suite.ctx, id1)
|
|
suite.False(found, "expected expired proposal to be closed")
|
|
_, found = suite.keeper.GetProposal(suite.ctx, id2)
|
|
suite.True(found, "expected non expired proposal to be not closed")
|
|
}
|
|
|
|
func (suite *ModuleTestSuite) TestBeginBlock_EnactsPassed() {
|
|
suite.app.InitializeFromGenesisStates()
|
|
|
|
// setup committee
|
|
normalCom := committee.NewMemberCommittee(12, "committee description", suite.addresses[:2],
|
|
[]committee.Permission{committee.GodPermission{}}, d("0.8"), time.Hour*24*7, types.FirstPastThePost)
|
|
|
|
suite.keeper.SetCommittee(suite.ctx, normalCom)
|
|
|
|
// setup 2 proposals
|
|
previousCDPDebtThreshold := suite.app.GetCDPKeeper().GetParams(suite.ctx).DebtAuctionThreshold
|
|
newDebtThreshold := previousCDPDebtThreshold.Add(i(1000000))
|
|
evenNewerDebtThreshold := newDebtThreshold.Add(i(1000000))
|
|
|
|
pprop1 := params.NewParameterChangeProposal("Title 1", "A description of this proposal.",
|
|
[]params.ParamChange{{
|
|
Subspace: cdptypes.ModuleName,
|
|
Key: string(cdp.KeyDebtThreshold),
|
|
Value: string(cdp.ModuleCdc.MustMarshalJSON(newDebtThreshold)),
|
|
}},
|
|
)
|
|
id1, err := suite.keeper.SubmitProposal(suite.ctx, normalCom.Members[0], normalCom.ID, pprop1)
|
|
suite.NoError(err)
|
|
|
|
pprop2 := params.NewParameterChangeProposal("Title 2", "A description of this proposal.",
|
|
[]params.ParamChange{{
|
|
Subspace: cdptypes.ModuleName,
|
|
Key: string(cdp.KeyDebtThreshold),
|
|
Value: string(cdp.ModuleCdc.MustMarshalJSON(evenNewerDebtThreshold)),
|
|
}},
|
|
)
|
|
id2, err := suite.keeper.SubmitProposal(suite.ctx, normalCom.Members[0], normalCom.ID, pprop2)
|
|
suite.NoError(err)
|
|
|
|
// add enough votes to make the first proposal pass, but not the second
|
|
suite.NoError(suite.keeper.AddVote(suite.ctx, id1, suite.addresses[0], types.Yes))
|
|
suite.NoError(suite.keeper.AddVote(suite.ctx, id1, suite.addresses[1], types.Yes))
|
|
suite.NoError(suite.keeper.AddVote(suite.ctx, id2, suite.addresses[0], types.Yes))
|
|
|
|
// Run BeginBlocker
|
|
suite.NotPanics(func() {
|
|
committee.BeginBlocker(suite.ctx, abci.RequestBeginBlock{}, suite.keeper)
|
|
})
|
|
|
|
// Check the param has been updated
|
|
suite.Equal(newDebtThreshold, suite.app.GetCDPKeeper().GetParams(suite.ctx).DebtAuctionThreshold)
|
|
// Check the passed proposal has gone
|
|
_, found := suite.keeper.GetProposal(suite.ctx, id1)
|
|
suite.False(found, "expected passed proposal to be enacted and closed")
|
|
_, found = suite.keeper.GetProposal(suite.ctx, id2)
|
|
suite.True(found, "expected non passed proposal to be not closed")
|
|
}
|
|
|
|
func (suite *ModuleTestSuite) TestBeginBlock_DoesntEnactFailed() {
|
|
suite.app.InitializeFromGenesisStates()
|
|
|
|
// setup committee
|
|
memberCom := committee.NewMemberCommittee(12, "committee description", suite.addresses[:1],
|
|
[]committee.Permission{committee.SoftwareUpgradePermission{}}, d("1.0"), time.Hour*24*7, types.FirstPastThePost)
|
|
|
|
firstBlockTime := time.Date(1998, 1, 1, 0, 0, 0, 0, time.UTC)
|
|
ctx := suite.ctx.WithBlockTime(firstBlockTime)
|
|
suite.keeper.SetCommittee(ctx, memberCom)
|
|
|
|
// setup an upgrade proposal
|
|
pprop1 := upgrade.NewSoftwareUpgradeProposal("Title 1", "A description of this proposal.",
|
|
upgrade.Plan{
|
|
Name: "upgrade-version-v0.23.1",
|
|
Time: firstBlockTime.Add(time.Second * 5),
|
|
Info: "some information about the upgrade",
|
|
},
|
|
)
|
|
id1, err := suite.keeper.SubmitProposal(ctx, memberCom.Members[0], memberCom.ID, pprop1)
|
|
suite.NoError(err)
|
|
|
|
// add enough votes to make the proposal pass
|
|
suite.NoError(suite.keeper.AddVote(ctx, id1, suite.addresses[0], types.Yes))
|
|
|
|
// Run BeginBlocker 10 seconds later (5 seconds after upgrade expires)
|
|
tenSecLaterCtx := ctx.WithBlockTime(ctx.BlockTime().Add(time.Second * 10))
|
|
suite.NotPanics(func() {
|
|
suite.app.BeginBlocker(tenSecLaterCtx, abci.RequestBeginBlock{})
|
|
})
|
|
|
|
// Check the plan has not been stored
|
|
_, found := suite.app.GetUpgradeKeeper().GetUpgradePlan(tenSecLaterCtx)
|
|
suite.False(found)
|
|
// Check the passed proposal has gone
|
|
_, found = suite.keeper.GetProposal(tenSecLaterCtx, id1)
|
|
suite.False(found, "expected failed proposal to be not enacted and closed")
|
|
|
|
// Check the chain doesn't halt
|
|
oneMinLaterCtx := ctx.WithBlockTime(ctx.BlockTime().Add(time.Minute).Add(time.Second))
|
|
suite.NotPanics(func() {
|
|
suite.app.BeginBlocker(oneMinLaterCtx, abci.RequestBeginBlock{})
|
|
})
|
|
}
|
|
|
|
func (suite *ModuleTestSuite) TestBeginBlock_EnactsPassedUpgrade() {
|
|
suite.app.InitializeFromGenesisStates()
|
|
|
|
// setup committee
|
|
memberCom := committee.MemberCommittee{
|
|
BaseCommittee: committee.BaseCommittee{
|
|
ID: 12,
|
|
Members: suite.addresses[:1],
|
|
Permissions: []committee.Permission{committee.SoftwareUpgradePermission{}},
|
|
VoteThreshold: d("1.0"),
|
|
ProposalDuration: time.Hour * 24 * 7,
|
|
TallyOption: types.FirstPastThePost,
|
|
},
|
|
}
|
|
|
|
firstBlockTime := time.Date(1998, 1, 1, 0, 0, 0, 0, time.UTC)
|
|
ctx := suite.ctx.WithBlockTime(firstBlockTime)
|
|
suite.keeper.SetCommittee(ctx, memberCom)
|
|
|
|
// setup an upgrade proposal
|
|
pprop1 := upgrade.NewSoftwareUpgradeProposal("Title 1", "A description of this proposal.",
|
|
upgrade.Plan{
|
|
Name: "upgrade-version-v0.23.1",
|
|
Time: firstBlockTime.Add(time.Minute * 1),
|
|
Info: "some information about the upgrade",
|
|
},
|
|
)
|
|
id1, err := suite.keeper.SubmitProposal(ctx, memberCom.Members[0], memberCom.ID, pprop1)
|
|
suite.NoError(err)
|
|
|
|
// add enough votes to make the proposal pass
|
|
suite.NoError(suite.keeper.AddVote(ctx, id1, suite.addresses[0], types.Yes))
|
|
|
|
// Run BeginBlocker
|
|
fiveSecLaterCtx := ctx.WithBlockTime(ctx.BlockTime().Add(time.Second * 5))
|
|
suite.NotPanics(func() {
|
|
suite.app.BeginBlocker(fiveSecLaterCtx, abci.RequestBeginBlock{})
|
|
})
|
|
|
|
// Check the plan has been stored
|
|
_, found := suite.app.GetUpgradeKeeper().GetUpgradePlan(fiveSecLaterCtx)
|
|
suite.True(found)
|
|
// Check the passed proposal has gone
|
|
_, found = suite.keeper.GetProposal(fiveSecLaterCtx, id1)
|
|
suite.False(found, "expected passed proposal to be enacted and closed")
|
|
|
|
// Check the chain halts
|
|
oneMinLaterCtx := ctx.WithBlockTime(ctx.BlockTime().Add(time.Minute))
|
|
suite.Panics(func() {
|
|
suite.app.BeginBlocker(oneMinLaterCtx, abci.RequestBeginBlock{})
|
|
})
|
|
}
|
|
|
|
func TestModuleTestSuite(t *testing.T) {
|
|
suite.Run(t, new(ModuleTestSuite))
|
|
}
|