mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 14:47:31 +00:00
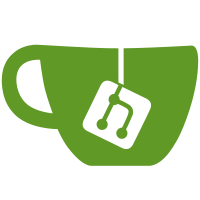
* Update incentive test to use beginblocker instead manual accumulation * Update integration test suite * Add base integration test, wip staking reward calculation * Get actual staking reward amounts from BeginBlocker events, calculate expected indexes * Simplify event parsing * Add initial earn accum test with real keepers * Add the rest of the accum integration tests with real keepers * Check if delegation rewards are zero before transferring * Update staking integration test to use updated methods
56 lines
1.3 KiB
Go
56 lines
1.3 KiB
Go
package keeper
|
|
|
|
import (
|
|
"fmt"
|
|
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
|
|
"github.com/kava-labs/kava/x/liquid/types"
|
|
)
|
|
|
|
func (k Keeper) CollectStakingRewards(
|
|
ctx sdk.Context,
|
|
validator sdk.ValAddress,
|
|
destinationModAccount string,
|
|
) (sdk.Coins, error) {
|
|
macc := k.accountKeeper.GetModuleAccount(ctx, types.ModuleAccountName)
|
|
|
|
// Ensure withdraw address is as expected
|
|
withdrawAddr := k.distributionKeeper.GetDelegatorWithdrawAddr(ctx, macc.GetAddress())
|
|
if !withdrawAddr.Equals(macc.GetAddress()) {
|
|
panic(fmt.Sprintf(
|
|
"unexpected withdraw address for liquid staking module account, expected %s, got %s",
|
|
macc.GetAddress(), withdrawAddr,
|
|
))
|
|
}
|
|
|
|
rewards, err := k.distributionKeeper.WithdrawDelegationRewards(ctx, macc.GetAddress(), validator)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
if rewards.IsZero() {
|
|
return rewards, nil
|
|
}
|
|
|
|
err = k.bankKeeper.SendCoinsFromModuleToModule(ctx, types.ModuleAccountName, destinationModAccount, rewards)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return rewards, nil
|
|
}
|
|
|
|
func (k Keeper) CollectStakingRewardsByDenom(
|
|
ctx sdk.Context,
|
|
derivativeDenom string,
|
|
destinationModAccount string,
|
|
) (sdk.Coins, error) {
|
|
valAddr, err := types.ParseLiquidStakingTokenDenom(derivativeDenom)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return k.CollectStakingRewards(ctx, valAddr, destinationModAccount)
|
|
}
|