mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 14:47:31 +00:00
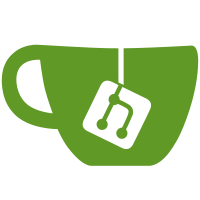
* comments from review Co-authored-by: Sunny Aggarwal <sunnya97@protonmail.ch> Co-authored-by: jmahess <maheswaran@google.com> Co-authored-by: Alexander Bezobchuk <alexanderbez@users.noreply.github.com> * add vote methods * add draft new param change permission * add and update tests * rename ParamChangePermission * account for perms becoming invalid at a later time * add debtParam to permission * add bep3 AssetParam to permissions * add pricefeed Markets to permission * add upgrade permission * move proposal passing to the begin blocker * fix iteration bug Co-authored-by: Federico Kunze <31522760+fedekunze@users.noreply.github.com> * address todos and audit comments * add proposal examples * refactor handler to be easier to read * address review comments * update comments Co-authored-by: Kevin Davis <kjydavis3@gmail.com> Co-authored-by: Sunny Aggarwal <sunnya97@protonmail.ch> Co-authored-by: jmahess <maheswaran@google.com> Co-authored-by: Alexander Bezobchuk <alexanderbez@users.noreply.github.com> Co-authored-by: Federico Kunze <31522760+fedekunze@users.noreply.github.com>
189 lines
4.3 KiB
Go
189 lines
4.3 KiB
Go
package keeper_test
|
|
|
|
import (
|
|
"testing"
|
|
"time"
|
|
|
|
"github.com/stretchr/testify/suite"
|
|
abci "github.com/tendermint/tendermint/abci/types"
|
|
|
|
govtypes "github.com/cosmos/cosmos-sdk/x/gov/types"
|
|
paramstypes "github.com/cosmos/cosmos-sdk/x/params/types"
|
|
|
|
"github.com/kava-labs/kava/app"
|
|
"github.com/kava-labs/kava/x/committee/types"
|
|
)
|
|
|
|
type TypesTestSuite struct {
|
|
suite.Suite
|
|
}
|
|
|
|
func (suite *TypesTestSuite) TestCommittee_HasPermissionsFor() {
|
|
|
|
testcases := []struct {
|
|
name string
|
|
permissions []types.Permission
|
|
pubProposal types.PubProposal
|
|
expectHasPermissions bool
|
|
}{
|
|
{
|
|
name: "normal (single permission)",
|
|
permissions: []types.Permission{types.SimpleParamChangePermission{
|
|
AllowedParams: types.AllowedParams{
|
|
{
|
|
Subspace: "cdp",
|
|
Key: "DebtThreshold",
|
|
},
|
|
}}},
|
|
pubProposal: paramstypes.NewParameterChangeProposal(
|
|
"A Title",
|
|
"A description of this proposal.",
|
|
[]paramstypes.ParamChange{
|
|
{
|
|
Subspace: "cdp",
|
|
Key: "DebtThreshold",
|
|
|
|
Value: `{"denom": "usdx", "amount": "1000000"}`,
|
|
},
|
|
},
|
|
),
|
|
expectHasPermissions: true,
|
|
},
|
|
{
|
|
name: "normal (multiple permissions)",
|
|
permissions: []types.Permission{
|
|
types.SimpleParamChangePermission{
|
|
AllowedParams: types.AllowedParams{
|
|
{
|
|
Subspace: "cdp",
|
|
Key: "DebtThreshold",
|
|
},
|
|
}},
|
|
types.TextPermission{},
|
|
},
|
|
pubProposal: govtypes.NewTextProposal("A Proposal Title", "A description of this proposal"),
|
|
expectHasPermissions: true,
|
|
},
|
|
{
|
|
name: "overruling permission",
|
|
permissions: []types.Permission{
|
|
types.SimpleParamChangePermission{
|
|
AllowedParams: types.AllowedParams{
|
|
{
|
|
Subspace: "cdp",
|
|
Key: "DebtThreshold",
|
|
},
|
|
}},
|
|
types.GodPermission{},
|
|
},
|
|
pubProposal: paramstypes.NewParameterChangeProposal(
|
|
"A Title",
|
|
"A description of this proposal.",
|
|
[]paramstypes.ParamChange{
|
|
{
|
|
Subspace: "cdp",
|
|
Key: "CollateralParams",
|
|
|
|
Value: `[]`,
|
|
},
|
|
},
|
|
),
|
|
expectHasPermissions: true,
|
|
},
|
|
{
|
|
name: "no permissions",
|
|
permissions: nil,
|
|
pubProposal: paramstypes.NewParameterChangeProposal(
|
|
"A Title",
|
|
"A description of this proposal.",
|
|
[]paramstypes.ParamChange{
|
|
{
|
|
Subspace: "cdp",
|
|
Key: "CollateralParams",
|
|
|
|
Value: `[]`,
|
|
},
|
|
},
|
|
),
|
|
expectHasPermissions: false,
|
|
},
|
|
{
|
|
name: "split permissions",
|
|
// These permissions looks like they allow the param change proposal, however a proposal must pass a single permission independently of others.
|
|
permissions: []types.Permission{
|
|
types.SimpleParamChangePermission{
|
|
AllowedParams: types.AllowedParams{
|
|
{
|
|
Subspace: "cdp",
|
|
Key: "DebtThreshold",
|
|
},
|
|
}},
|
|
types.SimpleParamChangePermission{
|
|
AllowedParams: types.AllowedParams{
|
|
{
|
|
Subspace: "cdp",
|
|
Key: "DebtParams",
|
|
},
|
|
}},
|
|
},
|
|
pubProposal: paramstypes.NewParameterChangeProposal(
|
|
"A Title",
|
|
"A description of this proposal.",
|
|
[]paramstypes.ParamChange{
|
|
{
|
|
Subspace: "cdp",
|
|
Key: "DebtThreshold",
|
|
|
|
Value: `{"denom": "usdx", "amount": "1000000"}`,
|
|
},
|
|
{
|
|
Subspace: "cdp",
|
|
Key: "DebtParams",
|
|
|
|
Value: `[]`,
|
|
},
|
|
},
|
|
),
|
|
expectHasPermissions: false,
|
|
},
|
|
{
|
|
name: "unregistered proposal",
|
|
permissions: []types.Permission{
|
|
types.SimpleParamChangePermission{
|
|
AllowedParams: types.AllowedParams{
|
|
{
|
|
Subspace: "cdp",
|
|
Key: "DebtThreshold",
|
|
},
|
|
}},
|
|
},
|
|
pubProposal: UnregisteredPubProposal{govtypes.TextProposal{Title: "A Title", Description: "A description."}},
|
|
expectHasPermissions: false,
|
|
},
|
|
}
|
|
|
|
for _, tc := range testcases {
|
|
suite.Run(tc.name, func() {
|
|
tApp := app.NewTestApp()
|
|
ctx := tApp.NewContext(true, abci.Header{})
|
|
tApp.InitializeFromGenesisStates()
|
|
com := types.NewCommittee(
|
|
12,
|
|
"a description of this committee",
|
|
nil,
|
|
tc.permissions,
|
|
d("0.5"),
|
|
24*time.Hour,
|
|
)
|
|
suite.Equal(
|
|
tc.expectHasPermissions,
|
|
com.HasPermissionsFor(ctx, tApp.Codec(), tApp.GetParamsKeeper(), tc.pubProposal),
|
|
)
|
|
})
|
|
}
|
|
}
|
|
|
|
func TestTypesTestSuite(t *testing.T) {
|
|
suite.Run(t, new(TypesTestSuite))
|
|
}
|