mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 14:47:31 +00:00
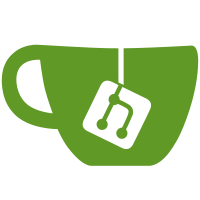
* organise testing committee gen state * remove repeated test app initialization * minor fixes from linter in tests * move more setup to SetupApp * split up KeeperTestSuite for each reward type * simplify KeeperTestSuite * simplify PayoutKeeperSuite * simplify DelegatorRewardSuite * simplify SupplyRewardsSuite * simplify BorrowRewardsSuite * simplify USDXRewardsSuite * add auth genesis builder for easier test setup * migrate all incentive tests to auth builder * add incentive genesis builder for easier setup migrate hard incentive tests * migrate all tests to incentive builder * add hard genesis builder * small tidy ups * deduplicate initialTime from borrow tests * deduplicate initialtTime from supply tests * deduplicate initialTime from usdx and keeper tests * deduplicate initialTime in delgator tests * deduplicate genesis time in payout test * deduplicate test app initialization * make authGenesisBuilder available for all modules * remove unused pricefeed setup * export incentive genesis builder * remove commented out test cases * migrate cdp test to new test state builders * migrate vv payout tests to use new builders * add SynchronizeHardBorrowReward unit test * extract calculatReward method * tidy up unit test for borrow rewards * add helper method to RewardIndexes * user helper to extract logic from SyncBorrowReward * add Get methods to (Multi)RewardIndexes * replace params.Subspace in keeper to test easier * add unit tests for usdx minting * refactor InitializeUSDXMintingClaim * add unit tests for InitializeHardBorrowRewards * refactor SynchronizeUSDXMintingReward * add unit tests for UpdateHardBorrowIndexDenoms * change rewardSource type to Dec needed by delegation rewards * fix typo in test names * refactor UpdateHardBorrowIndexDenoms * update genesis test TODO to use auth builder * add skipped test for bug in usdx sync * extract common method for calculating rewards * doc comment tidy * add unit tests for delegator rewards * tidy up test files * remove old TODOs * reaarrange InitializeHardDelegatorReward to fit with other init reward functions * duplicate borrow unit tests to create supply tests * add tests for syncing with zero rewards per second * refactor SynchronizeHardDelegatorRewards * refactor supply rewards in same way as borrow * fix total delegation calculation bug * fix new usdx reward bug * fix new supply/borrow reward bug * remove working comment * standardize behaviour when global factors missing * improve documentation for CalculateRewards * standardize variable names * remove panic from calculateSingleReward * wip * Tidy up comments * remove wip comment
120 lines
4.0 KiB
Go
120 lines
4.0 KiB
Go
package keeper_test
|
|
|
|
import (
|
|
"testing"
|
|
|
|
"github.com/stretchr/testify/suite"
|
|
|
|
hardtypes "github.com/kava-labs/kava/x/hard/types"
|
|
"github.com/kava-labs/kava/x/incentive/types"
|
|
)
|
|
|
|
// UpdateHardSupplyIndexDenomsTests runs unit tests for the keeper.UpdateHardSupplyIndexDenoms method
|
|
//
|
|
// inputs
|
|
// - claim in store if it exists (only claim.SupplyRewardIndexes)
|
|
// - global indexes in store
|
|
// - deposit function arg (only deposit.Amount)
|
|
//
|
|
// outputs
|
|
// - sets a claim
|
|
type UpdateHardSupplyIndexDenomsTests struct {
|
|
unitTester
|
|
}
|
|
|
|
func TestUpdateHardSupplyIndexDenoms(t *testing.T) {
|
|
suite.Run(t, new(UpdateHardSupplyIndexDenomsTests))
|
|
}
|
|
|
|
func (suite *UpdateHardSupplyIndexDenomsTests) TestClaimIndexesAreRemovedForDenomsNoLongerSupplied() {
|
|
claim := types.HardLiquidityProviderClaim{
|
|
BaseMultiClaim: types.BaseMultiClaim{
|
|
Owner: arbitraryAddress(),
|
|
},
|
|
SupplyRewardIndexes: nonEmptyMultiRewardIndexes,
|
|
}
|
|
suite.storeClaim(claim)
|
|
suite.storeGlobalSupplyIndexes(claim.SupplyRewardIndexes)
|
|
|
|
// remove one denom from the indexes already in the deposit
|
|
expectedIndexes := claim.SupplyRewardIndexes[1:]
|
|
deposit := hardtypes.Deposit{
|
|
Depositor: claim.Owner,
|
|
Amount: arbitraryCoinsWithDenoms(extractCollateralTypes(expectedIndexes)...),
|
|
}
|
|
|
|
suite.keeper.UpdateHardSupplyIndexDenoms(suite.ctx, deposit)
|
|
|
|
syncedClaim, _ := suite.keeper.GetHardLiquidityProviderClaim(suite.ctx, claim.Owner)
|
|
suite.Equal(expectedIndexes, syncedClaim.SupplyRewardIndexes)
|
|
}
|
|
|
|
func (suite *UpdateHardSupplyIndexDenomsTests) TestClaimIndexesAreAddedForNewlySuppliedDenoms() {
|
|
claim := types.HardLiquidityProviderClaim{
|
|
BaseMultiClaim: types.BaseMultiClaim{
|
|
Owner: arbitraryAddress(),
|
|
},
|
|
SupplyRewardIndexes: nonEmptyMultiRewardIndexes,
|
|
}
|
|
suite.storeClaim(claim)
|
|
globalIndexes := appendUniqueMultiRewardIndex(claim.SupplyRewardIndexes)
|
|
suite.storeGlobalSupplyIndexes(globalIndexes)
|
|
|
|
deposit := hardtypes.Deposit{
|
|
Depositor: claim.Owner,
|
|
Amount: arbitraryCoinsWithDenoms(extractCollateralTypes(globalIndexes)...),
|
|
}
|
|
|
|
suite.keeper.UpdateHardSupplyIndexDenoms(suite.ctx, deposit)
|
|
|
|
syncedClaim, _ := suite.keeper.GetHardLiquidityProviderClaim(suite.ctx, claim.Owner)
|
|
suite.Equal(globalIndexes, syncedClaim.SupplyRewardIndexes)
|
|
}
|
|
|
|
func (suite *UpdateHardSupplyIndexDenomsTests) TestClaimIndexesAreUnchangedWhenSuppliedDenomsUnchanged() {
|
|
claim := types.HardLiquidityProviderClaim{
|
|
BaseMultiClaim: types.BaseMultiClaim{
|
|
Owner: arbitraryAddress(),
|
|
},
|
|
SupplyRewardIndexes: nonEmptyMultiRewardIndexes,
|
|
}
|
|
suite.storeClaim(claim)
|
|
// Set global indexes with same denoms but different values.
|
|
// UpdateHardSupplyIndexDenoms should ignore the new values.
|
|
suite.storeGlobalSupplyIndexes(increaseAllRewardFactors(claim.SupplyRewardIndexes))
|
|
|
|
deposit := hardtypes.Deposit{
|
|
Depositor: claim.Owner,
|
|
Amount: arbitraryCoinsWithDenoms(extractCollateralTypes(claim.SupplyRewardIndexes)...),
|
|
}
|
|
|
|
suite.keeper.UpdateHardSupplyIndexDenoms(suite.ctx, deposit)
|
|
|
|
syncedClaim, _ := suite.keeper.GetHardLiquidityProviderClaim(suite.ctx, claim.Owner)
|
|
suite.Equal(claim.SupplyRewardIndexes, syncedClaim.SupplyRewardIndexes)
|
|
}
|
|
|
|
func (suite *UpdateHardSupplyIndexDenomsTests) TestEmptyClaimIndexesAreAddedForNewlySuppliedButNotRewardedDenoms() {
|
|
claim := types.HardLiquidityProviderClaim{
|
|
BaseMultiClaim: types.BaseMultiClaim{
|
|
Owner: arbitraryAddress(),
|
|
},
|
|
SupplyRewardIndexes: nonEmptyMultiRewardIndexes,
|
|
}
|
|
suite.storeClaim(claim)
|
|
suite.storeGlobalSupplyIndexes(claim.SupplyRewardIndexes)
|
|
|
|
// add a denom to the deposited amount that is not in the global or claim's indexes
|
|
expectedIndexes := appendUniqueEmptyMultiRewardIndex(claim.SupplyRewardIndexes)
|
|
depositedDenoms := extractCollateralTypes(expectedIndexes)
|
|
deposit := hardtypes.Deposit{
|
|
Depositor: claim.Owner,
|
|
Amount: arbitraryCoinsWithDenoms(depositedDenoms...),
|
|
}
|
|
|
|
suite.keeper.UpdateHardSupplyIndexDenoms(suite.ctx, deposit)
|
|
|
|
syncedClaim, _ := suite.keeper.GetHardLiquidityProviderClaim(suite.ctx, claim.Owner)
|
|
suite.Equal(expectedIndexes, syncedClaim.SupplyRewardIndexes)
|
|
}
|