mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 22:57:32 +00:00
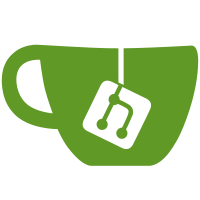
* rough auction type refactor * replace endTime type * split keeper file up * update store methods * move store methods to keeper.go * move nextAuctionID from params to genState * simplify auction type to not use pointers * add basic auction tests * update endblocker test * add payout to depositors feature * add more tests * move index updates to Get/Set for more safety * remove slightly unecessary ID type * remove unused message types * feat: add spec, update redundant type names * stop sending zero coins * use only one coins field in MsgPlaceBid * remove uncessary Auction interface methods * give auction types more accurate names * remove vuepress comments from spec * minor spec updates * update doc comments * add params validation * code cleanup, address review comments * resolve minor TODOs * sync spec with code Co-authored-by: Kevin Davis <karzak@users.noreply.github.com>
37 lines
802 B
Go
37 lines
802 B
Go
package keeper
|
|
|
|
import (
|
|
"testing"
|
|
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
"github.com/stretchr/testify/require"
|
|
)
|
|
|
|
func TestSplitIntIntoWeightedBuckets(t *testing.T) {
|
|
testCases := []struct {
|
|
name string
|
|
amount sdk.Int
|
|
buckets []sdk.Int
|
|
want []sdk.Int
|
|
}{
|
|
{"2split1,1", i(2), is(1, 1), is(1, 1)},
|
|
{"100split1,9", i(100), is(1, 9), is(10, 90)},
|
|
{"7split1,2", i(7), is(1, 2), is(2, 5)},
|
|
{"17split1,1,1", i(17), is(1, 1, 1), is(6, 6, 5)},
|
|
}
|
|
for _, tc := range testCases {
|
|
t.Run(tc.name, func(t *testing.T) {
|
|
got := splitIntIntoWeightedBuckets(tc.amount, tc.buckets)
|
|
require.Equal(t, tc.want, got)
|
|
})
|
|
}
|
|
}
|
|
|
|
func i(n int64) sdk.Int { return sdk.NewInt(n) }
|
|
func is(ns ...int64) (is []sdk.Int) {
|
|
for _, n := range ns {
|
|
is = append(is, sdk.NewInt(n))
|
|
}
|
|
return
|
|
}
|