mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-21 15:17:32 +00:00
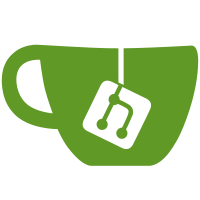
* add message types for swaps * add tx client commands * add test coverage for swap message deadlines * start handler swap tests, export handler result message event into private method, add stubbed keeper methods * add initial swap implementation to get handler tests passing; adds event specific for trades * add handler acceptance test for slippage in exact input and exact output swaps * implement slippage limit for swap keeper methods * add tests to ensure a user can only swap spendable coins * test pool not found, panic on invalid pool, and panic when module account does not have enough funds * validate that the exact output when using for exact swaps is less than the pool liquidity * nit: long line * add validation that swap output is greater than zero * add rest txs for swap messages * nit: lints * dry up swap keeper methods * from pr feedback - spelling and increase clairty around the output amount of a swap rounding to zero
63 lines
2.3 KiB
Go
63 lines
2.3 KiB
Go
package rest
|
|
|
|
import (
|
|
"github.com/gorilla/mux"
|
|
|
|
"github.com/cosmos/cosmos-sdk/client/context"
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
"github.com/cosmos/cosmos-sdk/types/rest"
|
|
)
|
|
|
|
// REST variable names
|
|
// nolint
|
|
const (
|
|
RestPool = "pool"
|
|
RestOwner = "owner"
|
|
)
|
|
|
|
// RegisterRoutes registers swap-related REST handlers to a router
|
|
func RegisterRoutes(cliCtx context.CLIContext, r *mux.Router) {
|
|
registerQueryRoutes(cliCtx, r)
|
|
registerTxRoutes(cliCtx, r)
|
|
}
|
|
|
|
// PostCreateDepositReq defines the properties of a deposit create request's body
|
|
type PostCreateDepositReq struct {
|
|
BaseReq rest.BaseReq `json:"base_req" yaml:"base_req"`
|
|
From sdk.AccAddress `json:"from" yaml:"from"`
|
|
TokenA sdk.Coin `json:"token_a" yaml:"token_a"`
|
|
TokenB sdk.Coin `json:"token_b" yaml:"token_b"`
|
|
Slippage sdk.Dec `json:"slippage" yaml:"slippage"`
|
|
Deadline int64 `json:"deadline" yaml:"deadline"`
|
|
}
|
|
|
|
// PostCreateWithdrawReq defines the properties of a withdraw create request's body
|
|
type PostCreateWithdrawReq struct {
|
|
BaseReq rest.BaseReq `json:"base_req" yaml:"base_req"`
|
|
From sdk.AccAddress `json:"from" yaml:"from"`
|
|
Shares sdk.Int `json:"shares" yaml:"shares"`
|
|
MinTokenA sdk.Coin `json:"token_a" yaml:"token_a"`
|
|
MinTokenB sdk.Coin `json:"token_b" yaml:"token_b"`
|
|
Deadline int64 `json:"deadline" yaml:"deadline"`
|
|
}
|
|
|
|
// PostCreateSwapExactForTokensReq trades an exact coinA for coinB
|
|
type PostCreateSwapExactForTokensReq struct {
|
|
BaseReq rest.BaseReq `json:"base_req" yaml:"base_req"`
|
|
Requester sdk.AccAddress `json:"requester" yaml:"requester"`
|
|
ExactTokenA sdk.Coin `json:"exact_token_a" yaml:"exact_token_a"`
|
|
TokenB sdk.Coin `json:"token_b" yaml:"token_b"`
|
|
Slippage sdk.Dec `json:"slippage" yaml:"slippage"`
|
|
Deadline int64 `json:"deadline" yaml:"deadline"`
|
|
}
|
|
|
|
// PostCreateSwapForExactTokensReq trades an exact coinA for coinB
|
|
type PostCreateSwapForExactTokensReq struct {
|
|
BaseReq rest.BaseReq `json:"base_req" yaml:"base_req"`
|
|
Requester sdk.AccAddress `json:"requester" yaml:"requester"`
|
|
TokenA sdk.Coin `json:"exact_token_a" yaml:"exact_token_a"`
|
|
ExactTokenB sdk.Coin `json:"token_b" yaml:"token_b"`
|
|
Slippage sdk.Dec `json:"slippage" yaml:"slippage"`
|
|
Deadline int64 `json:"deadline" yaml:"deadline"`
|
|
}
|