mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-11-10 10:05:18 +00:00
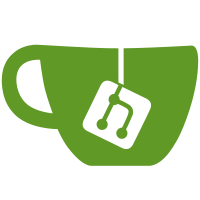
- Upgrade cosmos-sdk to v0.44.5 from v0.39.2 - Add Legacy Tx Endpoint for backwards compatibility - Add IBC v1.2.3 Support Co-authored-by: DracoLi <draco@dracoli.com> Co-authored-by: drklee3 <derrick@dlee.dev> Co-authored-by: denalimarsh <denalimarsh@gmail.com> Co-authored-by: Draco Li <draco@kava.io> Co-authored-by: Nick DeLuca <nickdeluca08@gmail.com> Co-authored-by: Kevin Davis <karzak@users.noreply.github.com> Co-authored-by: Denali Marsh <denali@kava.io>
65 lines
1.7 KiB
Go
65 lines
1.7 KiB
Go
package app
|
|
|
|
import (
|
|
"net/http"
|
|
|
|
"github.com/gorilla/mux"
|
|
|
|
"github.com/cosmos/cosmos-sdk/client/context"
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
"github.com/cosmos/cosmos-sdk/types/rest"
|
|
"github.com/cosmos/cosmos-sdk/x/auth"
|
|
)
|
|
|
|
// SimulateRequest represents attributes of a tx that will be simulated
|
|
type SimulateRequest struct {
|
|
Msgs []sdk.Msg `json:"msgs"`
|
|
Fee auth.StdFee `json:"fee"`
|
|
Memo string `json:"memo"`
|
|
}
|
|
|
|
// RegisterSimulateRoutes registers a tx simulate route to a mux router with
|
|
// a provided cli context
|
|
func RegisterSimulateRoutes(cliCtx context.CLIContext, r *mux.Router) {
|
|
r.HandleFunc("/tx/simulate", postAppSimulateHandlerFn(cliCtx)).Methods("POST")
|
|
}
|
|
|
|
// postAppSimulateHandlerFn handles tx simulate requests and returns the height and
|
|
// output of the simulation
|
|
func postAppSimulateHandlerFn(cliCtx context.CLIContext) http.HandlerFunc {
|
|
return func(w http.ResponseWriter, r *http.Request) {
|
|
var req SimulateRequest
|
|
if !rest.ReadRESTReq(w, r, cliCtx.Codec, &req) {
|
|
return
|
|
}
|
|
|
|
tx := auth.NewStdTx(
|
|
req.Msgs,
|
|
req.Fee,
|
|
[]auth.StdSignature{{}},
|
|
req.Memo,
|
|
)
|
|
|
|
txBz, err := cliCtx.Codec.MarshalBinaryLengthPrefixed(tx)
|
|
if err != nil {
|
|
rest.WriteErrorResponse(w, http.StatusBadRequest, err.Error())
|
|
return
|
|
}
|
|
|
|
bz, height, err := cliCtx.QueryWithData("/app/simulate", txBz)
|
|
cliCtx = cliCtx.WithHeight(height)
|
|
if err != nil {
|
|
rest.WriteErrorResponse(w, http.StatusBadRequest, err.Error())
|
|
return
|
|
}
|
|
|
|
var simRes sdk.SimulationResponse
|
|
if err := cliCtx.Codec.UnmarshalBinaryBare(bz, &simRes); err != nil {
|
|
rest.WriteErrorResponse(w, http.StatusInternalServerError, err.Error())
|
|
return
|
|
}
|
|
|
|
rest.PostProcessResponse(w, cliCtx, simRes)
|
|
}
|
|
}
|