mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 14:47:31 +00:00
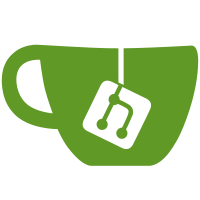
* Use custom aggregate handler for querying 'bkava' vault * Add 3rd bkava vault * Add special kava deposit handlers * Separate bkava logic to parent deposits handler * Rename single vault/account queries * Remove all deposits queries * Include empty vaults in /vaults query * Respond with empty values when querying account deposits with no deposits * return ukava value in bkava vault queries * remove refernce to specific staked token denom * return ukava value in bkava deposit queries Co-authored-by: rhuairahrighairigh <ruaridh.odonnell@gmail.com>
66 lines
1.5 KiB
Go
66 lines
1.5 KiB
Go
package keeper
|
|
|
|
import (
|
|
"github.com/cosmos/cosmos-sdk/codec"
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
"github.com/kava-labs/kava/x/earn/types"
|
|
|
|
paramtypes "github.com/cosmos/cosmos-sdk/x/params/types"
|
|
)
|
|
|
|
// Keeper keeper for the earn module
|
|
type Keeper struct {
|
|
key sdk.StoreKey
|
|
cdc codec.Codec
|
|
paramSubspace paramtypes.Subspace
|
|
hooks types.EarnHooks
|
|
accountKeeper types.AccountKeeper
|
|
bankKeeper types.BankKeeper
|
|
liquidKeeper types.LiquidKeeper
|
|
|
|
// Keepers used for strategies
|
|
hardKeeper types.HardKeeper
|
|
savingsKeeper types.SavingsKeeper
|
|
}
|
|
|
|
// NewKeeper creates a new keeper
|
|
func NewKeeper(
|
|
cdc codec.Codec,
|
|
key sdk.StoreKey,
|
|
paramstore paramtypes.Subspace,
|
|
accountKeeper types.AccountKeeper,
|
|
bankKeeper types.BankKeeper,
|
|
liquidKeeper types.LiquidKeeper,
|
|
hardKeeper types.HardKeeper,
|
|
savingsKeeper types.SavingsKeeper,
|
|
) Keeper {
|
|
if !paramstore.HasKeyTable() {
|
|
paramstore = paramstore.WithKeyTable(types.ParamKeyTable())
|
|
}
|
|
|
|
return Keeper{
|
|
key: key,
|
|
cdc: cdc,
|
|
paramSubspace: paramstore,
|
|
accountKeeper: accountKeeper,
|
|
bankKeeper: bankKeeper,
|
|
liquidKeeper: liquidKeeper,
|
|
hardKeeper: hardKeeper,
|
|
savingsKeeper: savingsKeeper,
|
|
}
|
|
}
|
|
|
|
// SetHooks adds hooks to the keeper.
|
|
func (k *Keeper) SetHooks(sh types.EarnHooks) *Keeper {
|
|
if k.hooks != nil {
|
|
panic("cannot set earn hooks twice")
|
|
}
|
|
k.hooks = sh
|
|
return k
|
|
}
|
|
|
|
// ClearHooks clears the hooks on the keeper
|
|
func (k *Keeper) ClearHooks() {
|
|
k.hooks = nil
|
|
}
|