mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 14:47:31 +00:00
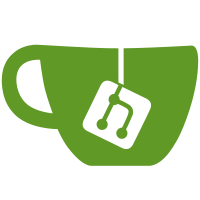
* Add basic savings strategy Supports ukava * Use clearer error message for ErrInvalidVaultStrategy * Add invariants * Separate specific vault/all vaults query, update depositor inconsistencies * Update swagger * Use single bkava AllowedVault for all bkava variants * Do not use allowedVault.Denom for value * Fix vault balance query * Update query to list bkava vaults * Add vaults query doc * Update grpc query test with no supply * Add earn hooks * Handle errors * Update outdated doc comments, make getAllowedVault_Raw private * Fix outdated comments, lints * Fix comment maths * Use AccAddressFromBech32 to validate message addresses
50 lines
1.6 KiB
Go
50 lines
1.6 KiB
Go
package keeper
|
|
|
|
import (
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
"github.com/kava-labs/kava/x/earn/types"
|
|
)
|
|
|
|
// SavingsStrategy defines the strategy that deposits assets to x/savings
|
|
type SavingsStrategy Keeper
|
|
|
|
var _ Strategy = (*SavingsStrategy)(nil)
|
|
|
|
// GetStrategyType returns the strategy type
|
|
func (s *SavingsStrategy) GetStrategyType() types.StrategyType {
|
|
return types.STRATEGY_TYPE_SAVINGS
|
|
}
|
|
|
|
// GetEstimatedTotalAssets returns the current value of all assets deposited
|
|
// in savings.
|
|
func (s *SavingsStrategy) GetEstimatedTotalAssets(ctx sdk.Context, denom string) (sdk.Coin, error) {
|
|
macc := s.accountKeeper.GetModuleAccount(ctx, types.ModuleName)
|
|
deposit, found := s.savingsKeeper.GetDeposit(ctx, macc.GetAddress())
|
|
if !found {
|
|
// Return 0 if no deposit exists for module account
|
|
return sdk.NewCoin(denom, sdk.ZeroInt()), nil
|
|
}
|
|
|
|
// Only return the deposit for the vault denom.
|
|
for _, coin := range deposit.Amount {
|
|
if coin.Denom == denom {
|
|
return coin, nil
|
|
}
|
|
}
|
|
|
|
// Return 0 if no deposit exists for the vault denom
|
|
return sdk.NewCoin(denom, sdk.ZeroInt()), nil
|
|
}
|
|
|
|
// Deposit deposits the specified amount of coins into savings.
|
|
func (s *SavingsStrategy) Deposit(ctx sdk.Context, amount sdk.Coin) error {
|
|
macc := s.accountKeeper.GetModuleAccount(ctx, types.ModuleName)
|
|
return s.savingsKeeper.Deposit(ctx, macc.GetAddress(), sdk.NewCoins(amount))
|
|
}
|
|
|
|
// Withdraw withdraws the specified amount of coins from savings.
|
|
func (s *SavingsStrategy) Withdraw(ctx sdk.Context, amount sdk.Coin) error {
|
|
macc := s.accountKeeper.GetModuleAccount(ctx, types.ModuleName)
|
|
return s.savingsKeeper.Withdraw(ctx, macc.GetAddress(), sdk.NewCoins(amount))
|
|
}
|