mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 14:47:31 +00:00
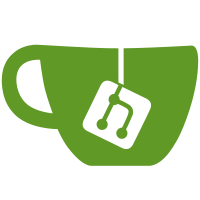
* add eip712 ante * minor cleanup * eip712 integration test with bridge conversion * fix issues * update bridge module * merge bridge module convert logic * update eip712 tests & update deps * remove v17 migrations * remove v17 migrations * fix genesis test * fix erc20 to coin tx * remove eth check * clean up imports * remove * fix evmutil cli * remove bridge comments * address feedback * rename mint method * add transfer checks for locking & unlocking funds * fix gas * increase gas even more * fix amount check Co-authored-by: Ruaridh <rhuairahrighairidh@users.noreply.github.com> Co-authored-by: Ruaridh <rhuairahrighairidh@users.noreply.github.com>
64 lines
1.4 KiB
Go
64 lines
1.4 KiB
Go
package cli
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
|
|
"github.com/cosmos/cosmos-sdk/client"
|
|
"github.com/cosmos/cosmos-sdk/client/flags"
|
|
"github.com/cosmos/cosmos-sdk/version"
|
|
"github.com/spf13/cobra"
|
|
|
|
"github.com/kava-labs/kava/x/evmutil/types"
|
|
)
|
|
|
|
// GetQueryCmd returns the cli query commands for this module
|
|
func GetQueryCmd() *cobra.Command {
|
|
evmutilQueryCmd := &cobra.Command{
|
|
Use: "evmutil",
|
|
Short: "Querying commands for the evmutil module",
|
|
DisableFlagParsing: true,
|
|
SuggestionsMinimumDistance: 2,
|
|
RunE: client.ValidateCmd,
|
|
}
|
|
|
|
cmds := []*cobra.Command{
|
|
QueryParamsCmd(),
|
|
}
|
|
|
|
for _, cmd := range cmds {
|
|
flags.AddQueryFlagsToCmd(cmd)
|
|
}
|
|
|
|
evmutilQueryCmd.AddCommand(cmds...)
|
|
|
|
return evmutilQueryCmd
|
|
}
|
|
|
|
// QueryParamsCmd queries the evmutil module parameters
|
|
func QueryParamsCmd() *cobra.Command {
|
|
return &cobra.Command{
|
|
Use: "params",
|
|
Short: "Query the evmutil module parameters",
|
|
Example: fmt.Sprintf(
|
|
"%[1]s q %[2]s params",
|
|
version.AppName, types.ModuleName,
|
|
),
|
|
Args: cobra.NoArgs,
|
|
RunE: func(cmd *cobra.Command, args []string) error {
|
|
clientCtx, err := client.GetClientQueryContext(cmd)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
queryClient := types.NewQueryClient(clientCtx)
|
|
res, err := queryClient.Params(context.Background(), &types.QueryParamsRequest{})
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
return clientCtx.PrintProto(&res.Params)
|
|
},
|
|
}
|
|
}
|