mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-11-10 18:15:19 +00:00
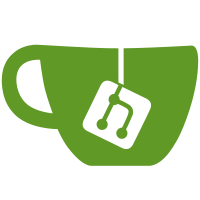
* Add initial earn claim migrations * Use existing types for migrations, add accural time migrations * Add MigrateRewardIndexes * Delete old state after migration * Update store test with multiple entries * Move key methods to keys.go * Update incentive consensus version to 3 * Call MigrateRewardIndexes in main migration, remove debugging statements * Fix migration version to v3 * Update module versions * Update outdated v1 comment
53 lines
1.4 KiB
Go
53 lines
1.4 KiB
Go
package v3
|
|
|
|
import (
|
|
"fmt"
|
|
|
|
"github.com/kava-labs/kava/x/incentive/types"
|
|
)
|
|
|
|
// Legacy store key prefixes
|
|
var (
|
|
EarnClaimKeyPrefix = []byte{0x18} // prefix for keys that store earn claims
|
|
EarnRewardIndexesKeyPrefix = []byte{0x19} // prefix for key that stores earn reward indexes
|
|
PreviousEarnRewardAccrualTimeKeyPrefix = []byte{0x20} // prefix for key that stores the previous time earn rewards accrued
|
|
)
|
|
|
|
func LegacyAccrualTimeKeyFromClaimType(claimType types.ClaimType) []byte {
|
|
switch claimType {
|
|
case types.CLAIM_TYPE_HARD_BORROW:
|
|
panic("todo")
|
|
case types.CLAIM_TYPE_HARD_SUPPLY:
|
|
panic("todo")
|
|
case types.CLAIM_TYPE_EARN:
|
|
return PreviousEarnRewardAccrualTimeKeyPrefix
|
|
case types.CLAIM_TYPE_SAVINGS:
|
|
panic("todo")
|
|
case types.CLAIM_TYPE_SWAP:
|
|
panic("todo")
|
|
case types.CLAIM_TYPE_USDX_MINTING:
|
|
panic("todo")
|
|
default:
|
|
panic(fmt.Sprintf("unrecognized claim type: %s", claimType))
|
|
}
|
|
}
|
|
|
|
func LegacyRewardIndexesKeyFromClaimType(claimType types.ClaimType) []byte {
|
|
switch claimType {
|
|
case types.CLAIM_TYPE_HARD_BORROW:
|
|
panic("todo")
|
|
case types.CLAIM_TYPE_HARD_SUPPLY:
|
|
panic("todo")
|
|
case types.CLAIM_TYPE_EARN:
|
|
return EarnRewardIndexesKeyPrefix
|
|
case types.CLAIM_TYPE_SAVINGS:
|
|
panic("todo")
|
|
case types.CLAIM_TYPE_SWAP:
|
|
panic("todo")
|
|
case types.CLAIM_TYPE_USDX_MINTING:
|
|
panic("todo")
|
|
default:
|
|
panic(fmt.Sprintf("unrecognized claim type: %s", claimType))
|
|
}
|
|
}
|