mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 14:47:31 +00:00
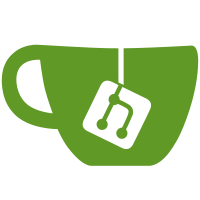
* Add accumulators * Move accumulator back to keeper package * Add earn specific accumulators * Move store methods to sub-package * Move earn accumulator * Rename accumulator files * Add store doc comment * Add earn accumulator tests, panic if accumulator not used with earn claim type * Update earn accumulator tests to use new methods * Add staking test for earn accumulator * Add test for accumulator proportional rewards * Remove old copy of GetProportionalRewardsPerSecond * Add test for basic accumulator * Fix AddIncentiveMultiRewardPeriod replacement * Deduplicate base earn reward accumulator * Check errors in tests * Validate RewardPeriods in Params.Validate() * Use adapter to fetch earn total shares
48 lines
1.3 KiB
Go
48 lines
1.3 KiB
Go
package incentive
|
|
|
|
import (
|
|
"fmt"
|
|
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
|
|
"github.com/kava-labs/kava/x/incentive/keeper"
|
|
)
|
|
|
|
// BeginBlocker runs at the start of every block
|
|
func BeginBlocker(ctx sdk.Context, k keeper.Keeper) {
|
|
params := k.GetParams(ctx)
|
|
|
|
for _, rp := range params.USDXMintingRewardPeriods {
|
|
k.AccumulateUSDXMintingRewards(ctx, rp)
|
|
}
|
|
for _, rp := range params.HardSupplyRewardPeriods {
|
|
k.AccumulateHardSupplyRewards(ctx, rp)
|
|
}
|
|
for _, rp := range params.HardBorrowRewardPeriods {
|
|
k.AccumulateHardBorrowRewards(ctx, rp)
|
|
}
|
|
for _, rp := range params.DelegatorRewardPeriods {
|
|
k.AccumulateDelegatorRewards(ctx, rp)
|
|
}
|
|
for _, rp := range params.SwapRewardPeriods {
|
|
k.AccumulateSwapRewards(ctx, rp)
|
|
}
|
|
for _, rp := range params.SavingsRewardPeriods {
|
|
k.AccumulateSavingsRewards(ctx, rp)
|
|
}
|
|
for _, rp := range params.EarnRewardPeriods {
|
|
if err := k.AccumulateEarnRewards(ctx, rp); err != nil {
|
|
panic(fmt.Sprintf("failed to accumulate earn rewards: %s", err))
|
|
}
|
|
}
|
|
|
|
// New generic RewardPeriods
|
|
for _, mrp := range params.RewardPeriods {
|
|
for _, rp := range mrp.RewardPeriods {
|
|
if err := k.AccumulateRewards(ctx, mrp.ClaimType, rp); err != nil {
|
|
panic(fmt.Errorf("failed to accumulate rewards for claim type %s: %w", mrp.ClaimType, err))
|
|
}
|
|
}
|
|
}
|
|
}
|