mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-11-10 10:05:18 +00:00
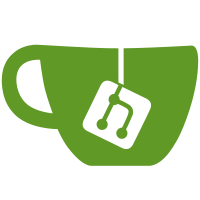
* spike: incentive/types * spike: incentive/types tests * spike: incentive/types/expected_keepers.go * spike: incentive/keeper * spike: incentive/keeper tests * spike: incentive/sims and incentive/sims tests * spike: incentive/module * spike: incentive/module tests * spike: hard/types * spike: hard/types hooks * spike: hard/types * spike: hard/keeper basics * spike: hard/keeper hooks * integrate hard/keeper/borrow.go * integrate hard/keeper/deposit.go * integrate hard/keeper/liquidation.go * integrate hard/keeper/withdraw.go * integrate hard/keeper/repay.go * spike: hard/sims * spike: hard/sims tests * spike: hard/client * spike: hard/module * integrate app.go * spike: x/hard/keeper compile tests * incentive/keeper test clean up * validate usdx incentive types in genesis * refactoring & fix deposit test * fix liquidaton tests * fix incentive tests for hard supply rewards * fix hard genesis tests * update incentive genesis state and params * update cdp rewards accumulation * update app init order and begin blocker order Co-authored-by: karzak <kjydavis3@gmail.com>
74 lines
3.1 KiB
Go
74 lines
3.1 KiB
Go
package simulation
|
|
|
|
import (
|
|
"fmt"
|
|
"testing"
|
|
"time"
|
|
|
|
"github.com/stretchr/testify/require"
|
|
|
|
"github.com/cosmos/cosmos-sdk/codec"
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
|
|
"github.com/tendermint/tendermint/libs/kv"
|
|
|
|
"github.com/kava-labs/kava/x/incentive/types"
|
|
)
|
|
|
|
func makeTestCodec() (cdc *codec.Codec) {
|
|
cdc = codec.New()
|
|
sdk.RegisterCodec(cdc)
|
|
types.RegisterCodec(cdc)
|
|
return
|
|
}
|
|
|
|
func TestDecodeDistributionStore(t *testing.T) {
|
|
cdc := makeTestCodec()
|
|
addr, _ := sdk.AccAddressFromBech32("kava15qdefkmwswysgg4qxgqpqr35k3m49pkx2jdfnw")
|
|
claim := types.NewUSDXMintingClaim(addr, sdk.NewCoin("ukava", sdk.NewInt(1000000)), types.RewardIndexes{types.NewRewardIndex("bnb-a", sdk.ZeroDec())})
|
|
prevBlockTime := time.Now().Add(time.Hour * -1).UTC()
|
|
factor := sdk.ZeroDec()
|
|
|
|
kvPairs := kv.Pairs{
|
|
kv.Pair{Key: types.USDXMintingClaimKeyPrefix, Value: cdc.MustMarshalBinaryBare(claim)},
|
|
kv.Pair{Key: []byte(types.PreviousUSDXMintingRewardAccrualTimeKeyPrefix), Value: cdc.MustMarshalBinaryBare(prevBlockTime)},
|
|
kv.Pair{Key: []byte(types.USDXMintingRewardFactorKeyPrefix), Value: cdc.MustMarshalBinaryBare(factor)},
|
|
// kv.Pair{Key: types.HardLiquidityClaimKeyPrefix, Value: cdc.MustMarshalBinaryBare(claim)},
|
|
// kv.Pair{Key: []byte(types.HardSupplyRewardFactorKeyPrefix), Value: cdc.MustMarshalBinaryBare(factor)},
|
|
// kv.Pair{Key: []byte(types.PreviousHardSupplyRewardAccrualTimeKeyPrefix), Value: cdc.MustMarshalBinaryBare(prevBlockTime)},
|
|
// kv.Pair{Key: []byte(types.HardBorrowRewardFactorKeyPrefix), Value: cdc.MustMarshalBinaryBare(factor)},
|
|
// kv.Pair{Key: []byte(types.PreviousHardBorrowRewardAccrualTimeKeyPrefix), Value: cdc.MustMarshalBinaryBare(prevBlockTime)},
|
|
// kv.Pair{Key: []byte(types.HardDelegatorRewardFactorKeyPrefix), Value: cdc.MustMarshalBinaryBare(factor)},
|
|
// kv.Pair{Key: []byte(types.PreviousHardDelegatorRewardAccrualTimeKeyPrefix), Value: cdc.MustMarshalBinaryBare(prevBlockTime)},
|
|
kv.Pair{Key: []byte{0x99}, Value: []byte{0x99}},
|
|
}
|
|
|
|
tests := []struct {
|
|
name string
|
|
expectedLog string
|
|
}{
|
|
{"USDXMintingClaim", fmt.Sprintf("%v\n%v", claim, claim)},
|
|
{"PreviousUSDXMintingRewardAccrualTime", fmt.Sprintf("%v\n%v", prevBlockTime, prevBlockTime)},
|
|
{"USDXMintingRewardFactor", fmt.Sprintf("%v\n%v", factor, factor)},
|
|
// {"HardLiquidityClaim", fmt.Sprintf("%v\n%v", claim, claim)},
|
|
// {"PreviousHardSupplyRewardAccrualTime", fmt.Sprintf("%v\n%v", prevBlockTime, prevBlockTime)},
|
|
// {"HardSupplyRewardFactor", fmt.Sprintf("%v\n%v", factor, factor)},
|
|
// {"PreviousHardBorrowRewardAccrualTime", fmt.Sprintf("%v\n%v", prevBlockTime, prevBlockTime)},
|
|
// {"HardBorrowRewardFactor", fmt.Sprintf("%v\n%v", factor, factor)},
|
|
// {"PreviousHardDelegatorRewardAccrualTime", fmt.Sprintf("%v\n%v", prevBlockTime, prevBlockTime)},
|
|
// {"HardSupplyDelegatorFactor", fmt.Sprintf("%v\n%v", factor, factor)},
|
|
{"other", ""},
|
|
}
|
|
for i, tt := range tests {
|
|
i, tt := i, tt
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
switch i {
|
|
case len(tests) - 1:
|
|
require.Panics(t, func() { DecodeStore(cdc, kvPairs[i], kvPairs[i]) }, tt.name)
|
|
default:
|
|
require.Equal(t, tt.expectedLog, DecodeStore(cdc, kvPairs[i], kvPairs[i]), tt.name)
|
|
}
|
|
})
|
|
}
|
|
}
|