mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 14:47:31 +00:00
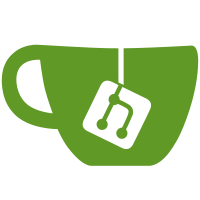
* wip: issuance module * add keeper and module methods * add begin blocker * add client * update events * add simulations * ignore v0.8 migration tests for now * ignore migration tests in ci * add test suite * update spec to match implementation details * add unblock method * address review comments * fix typos
56 lines
1.4 KiB
Go
56 lines
1.4 KiB
Go
package cli
|
|
|
|
import (
|
|
"fmt"
|
|
|
|
"github.com/spf13/cobra"
|
|
|
|
"github.com/cosmos/cosmos-sdk/client/context"
|
|
"github.com/cosmos/cosmos-sdk/client/flags"
|
|
"github.com/cosmos/cosmos-sdk/codec"
|
|
|
|
"github.com/kava-labs/kava/x/issuance/types"
|
|
)
|
|
|
|
// GetQueryCmd returns the cli query commands for the issuance module
|
|
func GetQueryCmd(queryRoute string, cdc *codec.Codec) *cobra.Command {
|
|
issuanceQueryCmd := &cobra.Command{
|
|
Use: types.ModuleName,
|
|
Short: fmt.Sprintf("Querying commands for the %s module", types.ModuleName),
|
|
}
|
|
|
|
issuanceQueryCmd.AddCommand(flags.GetCommands(
|
|
queryParamsCmd(queryRoute, cdc),
|
|
)...)
|
|
|
|
return issuanceQueryCmd
|
|
|
|
}
|
|
|
|
func queryParamsCmd(queryRoute string, cdc *codec.Codec) *cobra.Command {
|
|
return &cobra.Command{
|
|
Use: "params",
|
|
Short: fmt.Sprintf("get the %s module parameters", types.ModuleName),
|
|
Long: "Get the current global issuance module parameters.",
|
|
Args: cobra.NoArgs,
|
|
RunE: func(cmd *cobra.Command, args []string) error {
|
|
cliCtx := context.NewCLIContext().WithCodec(cdc)
|
|
|
|
// Query
|
|
route := fmt.Sprintf("custom/%s/%s", queryRoute, types.QueryGetParams)
|
|
res, height, err := cliCtx.QueryWithData(route, nil)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
cliCtx = cliCtx.WithHeight(height)
|
|
|
|
// Decode and print results
|
|
var params types.Params
|
|
if err := cdc.UnmarshalJSON(res, ¶ms); err != nil {
|
|
return fmt.Errorf("failed to unmarshal params: %w", err)
|
|
}
|
|
return cliCtx.PrintOutput(params)
|
|
},
|
|
}
|
|
}
|