mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 22:57:32 +00:00
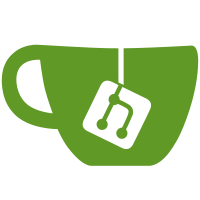
* Simplify strategies to lend and savings * Add hard and savings keepers * Add ctx to strategy interface, fill in lend strategy * Rename lend strategy to hard * Fix hard deposit query, fix withdraw bank send * Fix misleading borrow instead of withdraw for hard * Remove liquidateall strategy method * Withdraw tests * Add hard gs to testutil suite * Update withdraw tests with working hard strategy, clean strategy interface methods * Check allowed denom for strategy * Update GetVaultTotalValue doc note * Update error wrap message for unsupported denom * Remove unnecessary viewvault keeper * Withdraw amount from account value, not supplied value * Test value > supplied withdraw * Use dec when dividing for withdrawAmountPercent * Use the correct store prefix for vault shares * Update swap references to earn * Simplify vault shares, use a single share for all coins per address
48 lines
1.1 KiB
Go
48 lines
1.1 KiB
Go
package keeper
|
|
|
|
import (
|
|
"github.com/cosmos/cosmos-sdk/codec"
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
"github.com/kava-labs/kava/x/earn/types"
|
|
|
|
paramtypes "github.com/cosmos/cosmos-sdk/x/params/types"
|
|
)
|
|
|
|
// Keeper keeper for the earn module
|
|
type Keeper struct {
|
|
key sdk.StoreKey
|
|
cdc codec.Codec
|
|
paramSubspace paramtypes.Subspace
|
|
accountKeeper types.AccountKeeper
|
|
bankKeeper types.BankKeeper
|
|
|
|
// Keepers used for strategies
|
|
hardKeeper types.HardKeeper
|
|
savingsKeeper types.SavingsKeeper
|
|
}
|
|
|
|
// NewKeeper creates a new keeper
|
|
func NewKeeper(
|
|
cdc codec.Codec,
|
|
key sdk.StoreKey,
|
|
paramstore paramtypes.Subspace,
|
|
accountKeeper types.AccountKeeper,
|
|
bankKeeper types.BankKeeper,
|
|
hardKeeper types.HardKeeper,
|
|
savingsKeeper types.SavingsKeeper,
|
|
) Keeper {
|
|
if !paramstore.HasKeyTable() {
|
|
paramstore = paramstore.WithKeyTable(types.ParamKeyTable())
|
|
}
|
|
|
|
return Keeper{
|
|
key: key,
|
|
cdc: cdc,
|
|
paramSubspace: paramstore,
|
|
accountKeeper: accountKeeper,
|
|
bankKeeper: bankKeeper,
|
|
hardKeeper: hardKeeper,
|
|
savingsKeeper: savingsKeeper,
|
|
}
|
|
}
|