mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 22:57:32 +00:00
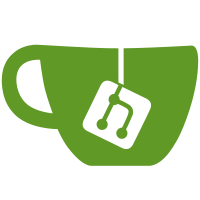
* Simplify strategies to lend and savings * Add hard and savings keepers * Add ctx to strategy interface, fill in lend strategy * Rename lend strategy to hard * Fix hard deposit query, fix withdraw bank send * Fix misleading borrow instead of withdraw for hard * Remove liquidateall strategy method * Withdraw tests * Add hard gs to testutil suite * Update withdraw tests with working hard strategy, clean strategy interface methods * Check allowed denom for strategy * Update GetVaultTotalValue doc note * Update error wrap message for unsupported denom * Remove unnecessary viewvault keeper * Withdraw amount from account value, not supplied value * Test value > supplied withdraw * Use dec when dividing for withdrawAmountPercent * Use the correct store prefix for vault shares * Update swap references to earn * Simplify vault shares, use a single share for all coins per address
49 lines
1.4 KiB
Go
49 lines
1.4 KiB
Go
package keeper
|
|
|
|
import (
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
"github.com/kava-labs/kava/x/earn/types"
|
|
)
|
|
|
|
// HardStrategy defines the strategy that deposits assets to Hard
|
|
type HardStrategy Keeper
|
|
|
|
var _ Strategy = (*HardStrategy)(nil)
|
|
|
|
func (s *HardStrategy) GetStrategyType() types.StrategyType {
|
|
return types.STRATEGY_TYPE_HARD
|
|
}
|
|
|
|
func (s *HardStrategy) IsDenomSupported(denom string) bool {
|
|
return denom == "usdx"
|
|
}
|
|
|
|
func (s *HardStrategy) GetEstimatedTotalAssets(ctx sdk.Context, denom string) (sdk.Coin, error) {
|
|
macc := s.accountKeeper.GetModuleAccount(ctx, types.ModuleName)
|
|
deposit, found := s.hardKeeper.GetSyncedDeposit(ctx, macc.GetAddress())
|
|
if !found {
|
|
// Return 0 if no deposit exists for module account
|
|
return sdk.NewCoin(denom, sdk.ZeroInt()), nil
|
|
}
|
|
|
|
// Only return the deposit for the vault denom.
|
|
for _, coin := range deposit.Amount {
|
|
if coin.Denom == denom {
|
|
return coin, nil
|
|
}
|
|
}
|
|
|
|
// Return 0 if no deposit exists for the vault denom
|
|
return sdk.NewCoin(denom, sdk.ZeroInt()), nil
|
|
}
|
|
|
|
func (s *HardStrategy) Deposit(ctx sdk.Context, amount sdk.Coin) error {
|
|
macc := s.accountKeeper.GetModuleAccount(ctx, types.ModuleName)
|
|
return s.hardKeeper.Deposit(ctx, macc.GetAddress(), sdk.NewCoins(amount))
|
|
}
|
|
|
|
func (s *HardStrategy) Withdraw(ctx sdk.Context, amount sdk.Coin) error {
|
|
macc := s.accountKeeper.GetModuleAccount(ctx, types.ModuleName)
|
|
return s.hardKeeper.Withdraw(ctx, macc.GetAddress(), sdk.NewCoins(amount))
|
|
}
|