mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 22:57:32 +00:00
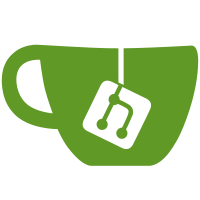
* update savings module macc balances getter * add savings keeper to incentive module * add savings keeper to incentive module #2 * savings reward syncing * claim savings reward * update txs, queries * update txs, queries #2 * update claim test * add savings keeper to incentive module in app.go * re-commit files to disk * define and call hooks * keeper methods for init/sync savings reward * update other tests for easier extendibility * init savings reward test * add helper methods to global incentive unit tester * sync savings test progress * savings init fix + completed tests * sync savings updates + tests * nit: simplify false check * fix: calculate set difference of incoming deposit denoms Co-authored-by: karzak <kjydavis3@gmail.com>
78 lines
2.6 KiB
Go
78 lines
2.6 KiB
Go
package keeper_test
|
|
|
|
import (
|
|
"testing"
|
|
|
|
"github.com/stretchr/testify/suite"
|
|
|
|
"github.com/kava-labs/kava/x/incentive/types"
|
|
)
|
|
|
|
// InitializeHardSupplyRewardTests runs unit tests for the keeper.InitializeHardSupplyReward method
|
|
type InitializeHardSupplyRewardTests struct {
|
|
unitTester
|
|
}
|
|
|
|
func TestInitializeHardSupplyReward(t *testing.T) {
|
|
suite.Run(t, new(InitializeHardSupplyRewardTests))
|
|
}
|
|
|
|
func (suite *InitializeHardSupplyRewardTests) TestClaimIndexesAreSetWhenClaimExists() {
|
|
claim := types.HardLiquidityProviderClaim{
|
|
BaseMultiClaim: types.BaseMultiClaim{
|
|
Owner: arbitraryAddress(),
|
|
},
|
|
// Indexes should always be empty when initialize is called.
|
|
// If initialize is called then the user must have repaid their deposit positions,
|
|
// which means UpdateHardSupplyIndexDenoms was called and should have remove indexes.
|
|
SupplyRewardIndexes: types.MultiRewardIndexes{},
|
|
}
|
|
suite.storeHardClaim(claim)
|
|
|
|
globalIndexes := nonEmptyMultiRewardIndexes
|
|
suite.storeGlobalSupplyIndexes(globalIndexes)
|
|
|
|
deposit := NewHardDepositBuilder(claim.Owner).
|
|
WithArbitrarySourceShares(extractCollateralTypes(globalIndexes)...).
|
|
Build()
|
|
|
|
suite.keeper.InitializeHardSupplyReward(suite.ctx, deposit)
|
|
|
|
syncedClaim, _ := suite.keeper.GetHardLiquidityProviderClaim(suite.ctx, claim.Owner)
|
|
suite.Equal(globalIndexes, syncedClaim.SupplyRewardIndexes)
|
|
}
|
|
func (suite *InitializeHardSupplyRewardTests) TestClaimIndexesAreSetWhenClaimDoesNotExist() {
|
|
globalIndexes := nonEmptyMultiRewardIndexes
|
|
suite.storeGlobalSupplyIndexes(globalIndexes)
|
|
|
|
owner := arbitraryAddress()
|
|
deposit := NewHardDepositBuilder(owner).
|
|
WithArbitrarySourceShares(extractCollateralTypes(globalIndexes)...).
|
|
Build()
|
|
|
|
suite.keeper.InitializeHardSupplyReward(suite.ctx, deposit)
|
|
|
|
syncedClaim, found := suite.keeper.GetHardLiquidityProviderClaim(suite.ctx, owner)
|
|
suite.True(found)
|
|
suite.Equal(globalIndexes, syncedClaim.SupplyRewardIndexes)
|
|
}
|
|
func (suite *InitializeHardSupplyRewardTests) TestClaimIndexesAreSetEmptyForMissingIndexes() {
|
|
|
|
globalIndexes := nonEmptyMultiRewardIndexes
|
|
suite.storeGlobalSupplyIndexes(globalIndexes)
|
|
|
|
owner := arbitraryAddress()
|
|
// Supply a denom that is not in the global indexes.
|
|
// This happens when a deposit denom has no rewards associated with it.
|
|
expectedIndexes := appendUniqueEmptyMultiRewardIndex(globalIndexes)
|
|
depositedDenoms := extractCollateralTypes(expectedIndexes)
|
|
deposit := NewHardDepositBuilder(owner).
|
|
WithArbitrarySourceShares(depositedDenoms...).
|
|
Build()
|
|
|
|
suite.keeper.InitializeHardSupplyReward(suite.ctx, deposit)
|
|
|
|
syncedClaim, _ := suite.keeper.GetHardLiquidityProviderClaim(suite.ctx, owner)
|
|
suite.Equal(expectedIndexes, syncedClaim.SupplyRewardIndexes)
|
|
}
|