mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 14:47:31 +00:00
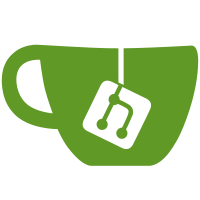
* update kvtool * add env vars for e2e automated upgrades * run kvtool with upgrade flags when enabled * add e2e test placeholder for upgrade handler tests * allow override of kvtool's kava.configTemplate * use the correct image tag when running e2e tests * update kvtool * always docker-build when running make test-e2e * refactor skip shutdown to always skip shutdown * add CtxAtHeight grpc helper * add Community & Earn queriers * expose UpgradeHeight in suite * document e2e chain upgrades * add reference to example branch
47 lines
1.0 KiB
Go
47 lines
1.0 KiB
Go
package util
|
|
|
|
import (
|
|
"context"
|
|
"crypto/tls"
|
|
"fmt"
|
|
"net/url"
|
|
"strconv"
|
|
|
|
"google.golang.org/grpc"
|
|
"google.golang.org/grpc/credentials"
|
|
"google.golang.org/grpc/metadata"
|
|
|
|
grpctypes "github.com/cosmos/cosmos-sdk/types/grpc"
|
|
)
|
|
|
|
// NewGrpcConnection parses a GRPC endpoint and creates a connection to it
|
|
func NewGrpcConnection(endpoint string) (*grpc.ClientConn, error) {
|
|
grpcUrl, err := url.Parse(endpoint)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
var secureOpt grpc.DialOption
|
|
switch grpcUrl.Scheme {
|
|
case "http":
|
|
secureOpt = grpc.WithInsecure()
|
|
case "https":
|
|
creds := credentials.NewTLS(&tls.Config{})
|
|
secureOpt = grpc.WithTransportCredentials(creds)
|
|
default:
|
|
return nil, fmt.Errorf("unknown grpc url scheme: %s", grpcUrl.Scheme)
|
|
}
|
|
|
|
grpcConn, err := grpc.Dial(grpcUrl.Host, secureOpt)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return grpcConn, nil
|
|
}
|
|
|
|
func CtxAtHeight(height int64) context.Context {
|
|
heightStr := strconv.FormatInt(height, 10)
|
|
return metadata.AppendToOutgoingContext(context.Background(), grpctypes.GRPCBlockHeightHeader, heightStr)
|
|
}
|