mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-11-10 10:05:18 +00:00
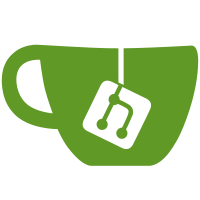
* feat: disable inflation upgrade logic * improve disable inflation comments * add upgrade tests * update changelog * split disable inflation upgrade and the upgrade check * remove pay rewards logic * clean up incentives test * add abci test * refactor upgradeTime and blockTime check * fix abci test * fix wrong pr in changelog * refactor disable inflation tests, behavior, and implementation - Unit tests are now shared between keeper and abci begin blocker since behavior is 100% the same - ABCI is unaware of keeper initial keeper logic branch (keeper methods required to be called in certain order by begin blocker) - Behavior of zero time is changed -- this now doesn't run for the zero time. This is more ideal for new chains (genesis should set all correct state instead of relying on inflation disable logic), and allows for a simpler implementation. - Begin blocker now panics if parameters are not in state * remove previous tests and implementation * remove previous block time keeper state -- not needed for inflation disalbing * move inflation disabling to private method and add more comments * remove unused key * use correct spelling for idempotence --------- Co-authored-by: Nick DeLuca <nickdeluca08@gmail.com>
163 lines
4.9 KiB
Go
163 lines
4.9 KiB
Go
package community
|
|
|
|
import (
|
|
"context"
|
|
"encoding/json"
|
|
"fmt"
|
|
|
|
"github.com/grpc-ecosystem/grpc-gateway/runtime"
|
|
"github.com/spf13/cobra"
|
|
|
|
"github.com/cosmos/cosmos-sdk/client"
|
|
"github.com/cosmos/cosmos-sdk/codec"
|
|
codectypes "github.com/cosmos/cosmos-sdk/codec/types"
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
"github.com/cosmos/cosmos-sdk/types/module"
|
|
|
|
abci "github.com/tendermint/tendermint/abci/types"
|
|
|
|
"github.com/kava-labs/kava/x/community/client/cli"
|
|
"github.com/kava-labs/kava/x/community/keeper"
|
|
"github.com/kava-labs/kava/x/community/types"
|
|
)
|
|
|
|
// ConsensusVersion defines the current module consensus version.
|
|
const ConsensusVersion = 2
|
|
|
|
var (
|
|
_ module.AppModule = AppModule{}
|
|
_ module.AppModuleBasic = AppModuleBasic{}
|
|
)
|
|
|
|
// AppModuleBasic app module basics object
|
|
type AppModuleBasic struct{}
|
|
|
|
// Name returns the module name
|
|
func (AppModuleBasic) Name() string {
|
|
return types.ModuleName
|
|
}
|
|
|
|
// RegisterLegacyAminoCodec register module codec
|
|
// Deprecated: unused but necessary to fulfill AppModuleBasic interface
|
|
func (AppModuleBasic) RegisterLegacyAminoCodec(cdc *codec.LegacyAmino) {
|
|
types.RegisterLegacyAminoCodec(cdc)
|
|
}
|
|
|
|
// DefaultGenesis default genesis state
|
|
func (AppModuleBasic) DefaultGenesis(cdc codec.JSONCodec) json.RawMessage {
|
|
gs := types.DefaultGenesisState()
|
|
return cdc.MustMarshalJSON(&gs)
|
|
}
|
|
|
|
// ValidateGenesis module validate genesis
|
|
func (AppModuleBasic) ValidateGenesis(cdc codec.JSONCodec, config client.TxEncodingConfig, bz json.RawMessage) error {
|
|
var gs types.GenesisState
|
|
err := cdc.UnmarshalJSON(bz, &gs)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
return gs.Validate()
|
|
}
|
|
|
|
// RegisterInterfaces implements InterfaceModule.RegisterInterfaces
|
|
func (a AppModuleBasic) RegisterInterfaces(registry codectypes.InterfaceRegistry) {
|
|
types.RegisterInterfaces(registry)
|
|
}
|
|
|
|
// RegisterGRPCGatewayRoutes registers the gRPC Gateway routes for the module.
|
|
func (a AppModuleBasic) RegisterGRPCGatewayRoutes(clientCtx client.Context, mux *runtime.ServeMux) {
|
|
if err := types.RegisterQueryHandlerClient(context.Background(), mux, types.NewQueryClient(clientCtx)); err != nil {
|
|
panic(err)
|
|
}
|
|
}
|
|
|
|
// GetTxCmd returns the root tx command for the module.
|
|
func (AppModuleBasic) GetTxCmd() *cobra.Command {
|
|
return cli.GetTxCmd()
|
|
}
|
|
|
|
// GetQueryCmd returns no root query command for the module.
|
|
func (AppModuleBasic) GetQueryCmd() *cobra.Command {
|
|
return cli.GetQueryCmd()
|
|
}
|
|
|
|
//____________________________________________________________________________
|
|
|
|
// AppModule app module type
|
|
type AppModule struct {
|
|
AppModuleBasic
|
|
|
|
keeper keeper.Keeper
|
|
accountKeeper types.AccountKeeper
|
|
}
|
|
|
|
// NewAppModule creates a new AppModule object
|
|
func NewAppModule(keeper keeper.Keeper, ak types.AccountKeeper) AppModule {
|
|
return AppModule{
|
|
AppModuleBasic: AppModuleBasic{},
|
|
keeper: keeper,
|
|
accountKeeper: ak,
|
|
}
|
|
}
|
|
|
|
// Name module name
|
|
func (am AppModule) Name() string {
|
|
return am.AppModuleBasic.Name()
|
|
}
|
|
|
|
// RegisterInvariants register module invariants
|
|
func (am AppModule) RegisterInvariants(_ sdk.InvariantRegistry) {}
|
|
|
|
// Route module message route name
|
|
// Deprecated: unused but necessary to fulfill AppModule interface
|
|
func (am AppModule) Route() sdk.Route { return sdk.Route{} }
|
|
|
|
// QuerierRoute module querier route name
|
|
// Deprecated: unused but necessary to fulfill AppModule interface
|
|
func (AppModule) QuerierRoute() string { return types.RouterKey }
|
|
|
|
// LegacyQuerierHandler returns no sdk.Querier.
|
|
// Deprecated: unused but necessary to fulfill AppModule interface
|
|
func (am AppModule) LegacyQuerierHandler(_ *codec.LegacyAmino) sdk.Querier {
|
|
return nil
|
|
}
|
|
|
|
// ConsensusVersion implements AppModule/ConsensusVersion.
|
|
func (AppModule) ConsensusVersion() uint64 { return ConsensusVersion }
|
|
|
|
// RegisterServices registers module services.
|
|
func (am AppModule) RegisterServices(cfg module.Configurator) {
|
|
types.RegisterMsgServer(cfg.MsgServer(), keeper.NewMsgServerImpl(am.keeper))
|
|
types.RegisterQueryServer(cfg.QueryServer(), keeper.NewQueryServerImpl(am.keeper))
|
|
|
|
m := keeper.NewMigrator(am.keeper)
|
|
if err := cfg.RegisterMigration(types.ModuleName, 1, m.Migrate1to2); err != nil {
|
|
panic(fmt.Sprintf("failed to migrate x/community from version 1 to 2: %v", err))
|
|
}
|
|
}
|
|
|
|
// InitGenesis module init-genesis
|
|
func (am AppModule) InitGenesis(ctx sdk.Context, cdc codec.JSONCodec, gs json.RawMessage) []abci.ValidatorUpdate {
|
|
var genState types.GenesisState
|
|
cdc.MustUnmarshalJSON(gs, &genState)
|
|
|
|
InitGenesis(ctx, am.keeper, am.accountKeeper, genState)
|
|
return []abci.ValidatorUpdate{}
|
|
}
|
|
|
|
// ExportGenesis module export genesis
|
|
func (am AppModule) ExportGenesis(ctx sdk.Context, cdc codec.JSONCodec) json.RawMessage {
|
|
gs := ExportGenesis(ctx, am.keeper)
|
|
return cdc.MustMarshalJSON(&gs)
|
|
}
|
|
|
|
// BeginBlock module begin-block
|
|
func (am AppModule) BeginBlock(ctx sdk.Context, _ abci.RequestBeginBlock) {
|
|
BeginBlocker(ctx, am.keeper)
|
|
}
|
|
|
|
// EndBlock module end-block
|
|
func (am AppModule) EndBlock(_ sdk.Context, _ abci.RequestEndBlock) []abci.ValidatorUpdate {
|
|
return []abci.ValidatorUpdate{}
|
|
}
|