mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-11-10 10:05:18 +00:00
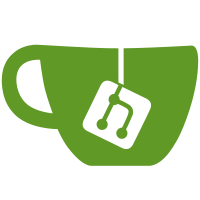
* first pass at genesis and msgs * add proposal generation * add permission generation * add decoder * add invariants * add committee change proposal generator * improve committee change proposal generation * fix error formatting * update sims to v0.38 * Update x/committee/keeper/invariants.go Co-Authored-By: Denali Marsh <denali@kava.io> * Update x/committee/keeper/invariants.go Co-Authored-By: Denali Marsh <denali@kava.io> * tidy up comments * tidy up random helpers * add committee to ImportExport test * add member check to vote invariant * fix comment wording Co-authored-by: Kevin Davis <karzak@users.noreply.github.com> Co-authored-by: Denali Marsh <denali@kava.io> Co-authored-by: Kevin Davis <karzak@users.noreply.github.com>
44 lines
1.4 KiB
Go
44 lines
1.4 KiB
Go
package simulation
|
|
|
|
import (
|
|
"bytes"
|
|
"fmt"
|
|
|
|
"github.com/cosmos/cosmos-sdk/codec"
|
|
|
|
"github.com/tendermint/tendermint/libs/kv"
|
|
|
|
"github.com/kava-labs/kava/x/committee/types"
|
|
)
|
|
|
|
// DecodeStore unmarshals the KVPair's Value to the corresponding module type
|
|
func DecodeStore(cdc *codec.Codec, kvA, kvB kv.Pair) string {
|
|
switch {
|
|
case bytes.Equal(kvA.Key[:1], types.CommitteeKeyPrefix):
|
|
var committeeA, committeeB types.Committee
|
|
cdc.MustUnmarshalBinaryLengthPrefixed(kvA.Value, &committeeA)
|
|
cdc.MustUnmarshalBinaryLengthPrefixed(kvB.Value, &committeeB)
|
|
return fmt.Sprintf("%v\n%v", committeeA, committeeB)
|
|
|
|
case bytes.Equal(kvA.Key[:1], types.ProposalKeyPrefix):
|
|
var proposalA, proposalB types.Proposal
|
|
cdc.MustUnmarshalBinaryLengthPrefixed(kvA.Value, &proposalA)
|
|
cdc.MustUnmarshalBinaryLengthPrefixed(kvB.Value, &proposalB)
|
|
return fmt.Sprintf("%v\n%v", proposalA, proposalB)
|
|
|
|
case bytes.Equal(kvA.Key[:1], types.VoteKeyPrefix):
|
|
var voteA, voteB types.Vote
|
|
cdc.MustUnmarshalBinaryLengthPrefixed(kvA.Value, &voteA)
|
|
cdc.MustUnmarshalBinaryLengthPrefixed(kvB.Value, &voteB)
|
|
return fmt.Sprintf("%v\n%v", voteA, voteB)
|
|
|
|
case bytes.Equal(kvA.Key[:1], types.NextProposalIDKey):
|
|
proposalIDA := types.Uint64FromBytes(kvA.Value)
|
|
proposalIDB := types.Uint64FromBytes(kvB.Value)
|
|
return fmt.Sprintf("%d\n%d", proposalIDA, proposalIDB)
|
|
|
|
default:
|
|
panic(fmt.Sprintf("invalid %s key prefix %X", types.ModuleName, kvA.Key[:1]))
|
|
}
|
|
}
|