mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-11-10 18:15:19 +00:00
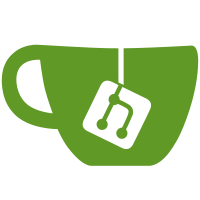
* Add basic savings strategy Supports ukava * Use clearer error message for ErrInvalidVaultStrategy * Add invariants * Separate specific vault/all vaults query, update depositor inconsistencies * Update swagger * Use single bkava AllowedVault for all bkava variants * Do not use allowedVault.Denom for value * Fix vault balance query * Update query to list bkava vaults * Add vaults query doc * Update grpc query test with no supply * Add earn hooks * Handle errors * Update outdated doc comments, make getAllowedVault_Raw private * Fix outdated comments, lints * Fix comment maths * Use AccAddressFromBech32 to validate message addresses
41 lines
1.2 KiB
Go
41 lines
1.2 KiB
Go
package keeper
|
|
|
|
import (
|
|
"fmt"
|
|
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
"github.com/kava-labs/kava/x/earn/types"
|
|
)
|
|
|
|
// Strategy is the interface that must be implemented by a strategy.
|
|
type Strategy interface {
|
|
// GetStrategyType returns the strategy type
|
|
GetStrategyType() types.StrategyType
|
|
|
|
// GetEstimatedTotalAssets returns the estimated total assets of the
|
|
// strategy with the specified denom. This is the value if the strategy were
|
|
// to liquidate all assets.
|
|
//
|
|
// **Note:** This may not reflect the true value as it may become outdated
|
|
// from market changes.
|
|
GetEstimatedTotalAssets(ctx sdk.Context, denom string) (sdk.Coin, error)
|
|
|
|
// Deposit the specified amount of coins into this strategy.
|
|
Deposit(ctx sdk.Context, amount sdk.Coin) error
|
|
|
|
// Withdraw the specified amount of coins from this strategy.
|
|
Withdraw(ctx sdk.Context, amount sdk.Coin) error
|
|
}
|
|
|
|
// GetStrategy returns the strategy for the given strategy type.
|
|
func (k *Keeper) GetStrategy(strategyType types.StrategyType) (Strategy, error) {
|
|
switch strategyType {
|
|
case types.STRATEGY_TYPE_HARD:
|
|
return (*HardStrategy)(k), nil
|
|
case types.STRATEGY_TYPE_SAVINGS:
|
|
return (*SavingsStrategy)(k), nil
|
|
default:
|
|
return nil, fmt.Errorf("unknown strategy type: %s", strategyType)
|
|
}
|
|
}
|