mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 14:47:31 +00:00
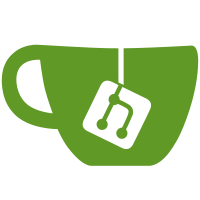
* add new field upgrade_time_set_staking_rewards_per_second with intention of integrating into the disable inflation logic to set an initial staking reward time * when the disable inflation upgrade time occurs, set the staking rewards per second to the value specified by the new upgrade_time_set_staking_rewards_per_second. This will allow a decoupled implementation between the ugprade switching logic, and the core functionality of paying staking rewards from the pool * add staking rewards state to community keeper and community module genesis that is required to calculate and track staking reward payouts accross blocks * add implementation of staking reward payouts * remove unused error * touch up tests and add a test case that fully tests behavior when pool is drained * add function comments * refactor and pull out main calculation to private pure function with no dependence on keeper * zero out default parameters -- these are too chain specific to have useful defaults * small touch ups on comments, test cases * use correct Int from sdkmath, not old sdk types; update protonet genesis for new parmater * fix copy pasta comment * use bond denom from staking keeper instead of referncing ukava directly * add staking reward state for valid genesis * update kvtool genesis for new params and rewards state
46 lines
922 B
Go
46 lines
922 B
Go
package keeper
|
|
|
|
import (
|
|
"fmt"
|
|
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
|
|
"github.com/kava-labs/kava/x/community/types"
|
|
)
|
|
|
|
// GetParams returns the params from the store
|
|
func (k Keeper) GetParams(ctx sdk.Context) (types.Params, bool) {
|
|
store := ctx.KVStore(k.key)
|
|
|
|
bz := store.Get(types.ParamsKey)
|
|
if bz == nil {
|
|
return types.Params{}, false
|
|
}
|
|
|
|
params := types.Params{}
|
|
k.cdc.MustUnmarshal(bz, ¶ms)
|
|
|
|
return params, true
|
|
}
|
|
|
|
// SetParams sets params on the store
|
|
func (k Keeper) SetParams(ctx sdk.Context, params types.Params) {
|
|
if err := params.Validate(); err != nil {
|
|
panic(fmt.Sprintf("invalid params: %s", err))
|
|
}
|
|
|
|
store := ctx.KVStore(k.key)
|
|
bz := k.cdc.MustMarshal(¶ms)
|
|
|
|
store.Set(types.ParamsKey, bz)
|
|
}
|
|
|
|
func (k Keeper) mustGetParams(ctx sdk.Context) types.Params {
|
|
params, found := k.GetParams(ctx)
|
|
if !found {
|
|
panic("invalid state: module parameters not found")
|
|
}
|
|
|
|
return params
|
|
}
|