mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 14:47:31 +00:00
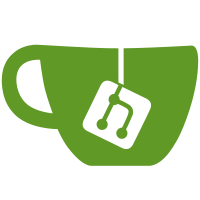
* Update cometbft, cosmos, ethermint, and ibc-go * Replace github.com/tendermint/tendermint by github.com/cometbft/cometbft * Replace github.com/tendermint/tm-db by github.com/cometbft/cometbft-db * Replace gogo/protobuf with cosmos/gogoproto & simapp replacement * Replace cosmos-sdk/simapp/helpers with cosmos-sdk/testutil/sims * Remove no longer used simulations * Replace ibchost with ibcexported See https://github.com/cosmos/ibc-go/blob/v7.2.2/docs/migrations/v6-to-v7.md#ibc-module-constants * Add new consensus params keeper * Add consensus keeper to blockers * Fix keeper and module issues in app.go * Add IsSendEnabledCoins and update SetParams interface changes * Fix protobuf build for cosmos 47 (#1800) * fix cp errors by using -f; fix lint by only linting our proto dir; and use proofs.proto directly from ics23 for ibc-go v7 * run proto-all; commit updated third party deps and swagger changes * regenerate proto files * use correct gocosmos build plugin for buf * re-gen all protobuf files to update paths for new gocosmos plugin * update protoc and buf to latest versions * fix staking keeper issues in app.go * update tally handler for gov changes * chain id fix and flag fixes * update deps for cometbft 47.7 upgrade * remove all module legacy queriers * update stakingKeeper to pointer * Replace ModuleCdc from govv1beta1 to govcodec * remove simulations * abci.LastCommitInfo → abci.CommitInfo * Remove unused code in keys.go * simapp.MakeTestEncodingConfig -> moduletestutil.MakeTestEncodingConfi * Fix chain id issues in tests * Fix remaining unit test issues * Update changelog for upgrade * Fix e2e tests using updated kvtool * Update protonet to v47 compatible genesis * Bump cometbft-db to v0.9.1-kava.1 * Update kvtool * Remove extra changelog * Fix merged rocksdb issues * go mod cleanup * Bump cometbft-db to v9 and go to 1.21 * Bump rocksdb version to v8.10.0 * Update kvtool to latest version * Update gin to v1.9.0 * Use ibctm.ModuleName in app_test * Fallback to genesis chain id instead of client toml * Remove all simulations * Fix cdp migrations issue with v47 * Update dependencies to correct tags --------- Co-authored-by: Nick DeLuca <nickdeluca08@gmail.com>
155 lines
3.8 KiB
Go
155 lines
3.8 KiB
Go
package keeper
|
|
|
|
import (
|
|
"fmt"
|
|
|
|
"github.com/cosmos/cosmos-sdk/client"
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
|
|
"github.com/kava-labs/kava/x/cdp/types"
|
|
)
|
|
|
|
// FilterCDPs queries the store for all CDPs that match query params
|
|
func FilterCDPs(ctx sdk.Context, k Keeper, params types.QueryCdpsParams) (types.AugmentedCDPs, error) {
|
|
var matchCollateralType, matchOwner, matchID, matchRatio types.CDPs
|
|
|
|
// match cdp owner (if supplied)
|
|
if len(params.Owner) > 0 {
|
|
denoms := k.GetCollateralTypes(ctx)
|
|
for _, denom := range denoms {
|
|
cdp, found := k.GetCdpByOwnerAndCollateralType(ctx, params.Owner, denom)
|
|
if found {
|
|
matchOwner = append(matchOwner, cdp)
|
|
}
|
|
}
|
|
}
|
|
|
|
// match cdp collateral denom (if supplied)
|
|
if len(params.CollateralType) > 0 {
|
|
// if owner is specified only iterate over already matched cdps for efficiency
|
|
if len(params.Owner) > 0 {
|
|
for _, cdp := range matchOwner {
|
|
if cdp.Type == params.CollateralType {
|
|
matchCollateralType = append(matchCollateralType, cdp)
|
|
}
|
|
}
|
|
} else {
|
|
_, found := k.GetCollateral(ctx, params.CollateralType)
|
|
if !found {
|
|
return nil, fmt.Errorf("invalid collateral type")
|
|
}
|
|
matchCollateralType = k.GetAllCdpsByCollateralType(ctx, params.CollateralType)
|
|
}
|
|
}
|
|
|
|
// match cdp ID (if supplied)
|
|
if params.ID != 0 {
|
|
denoms := k.GetCollateralTypes(ctx)
|
|
for _, denom := range denoms {
|
|
cdp, found := k.GetCDP(ctx, denom, params.ID)
|
|
if found {
|
|
matchID = append(matchID, cdp)
|
|
}
|
|
}
|
|
}
|
|
|
|
// match cdp ratio (if supplied)
|
|
if !params.Ratio.IsNil() && params.Ratio.GT(sdk.ZeroDec()) {
|
|
denoms := k.GetCollateralTypes(ctx)
|
|
for _, denom := range denoms {
|
|
ratio, err := k.CalculateCollateralizationRatioFromAbsoluteRatio(ctx, denom, params.Ratio, "liquidation")
|
|
if err != nil {
|
|
continue
|
|
}
|
|
cdpsUnderRatio := k.GetAllCdpsByCollateralTypeAndRatio(ctx, denom, ratio)
|
|
matchRatio = append(matchRatio, cdpsUnderRatio...)
|
|
}
|
|
}
|
|
|
|
var commonCDPs types.CDPs
|
|
// If no params specified, fetch all CDPs
|
|
if params.CollateralType == "" && len(params.Owner) == 0 && params.ID == 0 && params.Ratio.Equal(sdk.ZeroDec()) {
|
|
commonCDPs = k.GetAllCdps(ctx)
|
|
}
|
|
|
|
// Find the intersection of any matched CDPs
|
|
if params.CollateralType != "" {
|
|
if len(matchCollateralType) == 0 {
|
|
return nil, nil
|
|
}
|
|
|
|
commonCDPs = matchCollateralType
|
|
}
|
|
|
|
if len(params.Owner) > 0 {
|
|
if len(matchCollateralType) > 0 {
|
|
if len(commonCDPs) > 0 {
|
|
commonCDPs = FindIntersection(commonCDPs, matchOwner)
|
|
} else {
|
|
commonCDPs = matchOwner
|
|
}
|
|
} else {
|
|
commonCDPs = matchOwner
|
|
}
|
|
}
|
|
|
|
if params.ID != 0 {
|
|
if len(matchID) == 0 {
|
|
return nil, nil
|
|
}
|
|
|
|
if len(commonCDPs) > 0 {
|
|
commonCDPs = FindIntersection(commonCDPs, matchID)
|
|
} else {
|
|
commonCDPs = matchID
|
|
}
|
|
}
|
|
|
|
if !params.Ratio.IsNil() && params.Ratio.GT(sdk.ZeroDec()) {
|
|
if len(matchRatio) == 0 {
|
|
return nil, nil
|
|
}
|
|
|
|
if len(commonCDPs) > 0 {
|
|
commonCDPs = FindIntersection(commonCDPs, matchRatio)
|
|
} else {
|
|
commonCDPs = matchRatio
|
|
}
|
|
}
|
|
|
|
// Load augmented CDPs
|
|
var augmentedCDPs types.AugmentedCDPs
|
|
for _, cdp := range commonCDPs {
|
|
augmentedCDP := k.LoadAugmentedCDP(ctx, cdp)
|
|
augmentedCDPs = append(augmentedCDPs, augmentedCDP)
|
|
}
|
|
|
|
// Apply page and limit params
|
|
start, end := client.Paginate(len(augmentedCDPs), params.Page, params.Limit, 100)
|
|
if start < 0 || end < 0 {
|
|
return nil, nil
|
|
}
|
|
|
|
return augmentedCDPs[start:end], nil
|
|
}
|
|
|
|
// FindIntersection finds the intersection of two CDP arrays in linear time complexity O(n + n)
|
|
func FindIntersection(x types.CDPs, y types.CDPs) types.CDPs {
|
|
cdpSet := make(types.CDPs, 0)
|
|
cdpMap := make(map[uint64]bool)
|
|
|
|
for i := 0; i < len(x); i++ {
|
|
cdp := x[i]
|
|
cdpMap[cdp.ID] = true
|
|
}
|
|
|
|
for i := 0; i < len(y); i++ {
|
|
cdp := y[i]
|
|
if _, found := cdpMap[cdp.ID]; found {
|
|
cdpSet = append(cdpSet, cdp)
|
|
}
|
|
}
|
|
|
|
return cdpSet
|
|
}
|