mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 14:47:31 +00:00
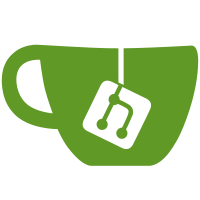
* define and generate proto types * implement savings claim msg + test * implement savings claim + test * register msg and add store keys * implement savings claim keeper methods * update function comment
95 lines
3.6 KiB
Protocol Buffer
95 lines
3.6 KiB
Protocol Buffer
syntax = "proto3";
|
|
package kava.incentive.v1beta1;
|
|
|
|
import "gogoproto/gogo.proto";
|
|
|
|
option go_package = "github.com/kava-labs/kava/x/incentive/types";
|
|
|
|
// Msg defines the incentive Msg service.
|
|
service Msg {
|
|
// ClaimUSDXMintingReward is a message type used to claim USDX minting rewards
|
|
rpc ClaimUSDXMintingReward(MsgClaimUSDXMintingReward) returns (MsgClaimUSDXMintingRewardResponse);
|
|
|
|
// ClaimHardReward is a message type used to claim Hard liquidity provider rewards
|
|
rpc ClaimHardReward(MsgClaimHardReward) returns (MsgClaimHardRewardResponse);
|
|
|
|
// ClaimDelegatorReward is a message type used to claim delegator rewards
|
|
rpc ClaimDelegatorReward(MsgClaimDelegatorReward) returns (MsgClaimDelegatorRewardResponse);
|
|
|
|
// ClaimSwapReward is a message type used to claim delegator rewards
|
|
rpc ClaimSwapReward(MsgClaimSwapReward) returns (MsgClaimSwapRewardResponse);
|
|
|
|
// ClaimSavingsReward is a message type used to claim savings rewards
|
|
rpc ClaimSavingsReward(MsgClaimSavingsReward) returns (MsgClaimSavingsRewardResponse);
|
|
}
|
|
|
|
// Selection is a pair of denom and multiplier name. It holds the choice of multiplier a user makes when they claim a
|
|
// denom.
|
|
message Selection {
|
|
option (gogoproto.equal) = false;
|
|
option (gogoproto.goproto_getters) = false;
|
|
|
|
string denom = 1;
|
|
string multiplier_name = 2;
|
|
}
|
|
|
|
// MsgClaimUSDXMintingReward message type used to claim USDX minting rewards
|
|
message MsgClaimUSDXMintingReward {
|
|
option (gogoproto.equal) = false;
|
|
option (gogoproto.goproto_getters) = false;
|
|
|
|
string sender = 1;
|
|
string multiplier_name = 2;
|
|
}
|
|
|
|
// MsgClaimUSDXMintingRewardResponse defines the Msg/ClaimUSDXMintingReward response type.
|
|
message MsgClaimUSDXMintingRewardResponse {}
|
|
|
|
// MsgClaimHardReward message type used to claim Hard liquidity provider rewards
|
|
message MsgClaimHardReward {
|
|
option (gogoproto.equal) = false;
|
|
option (gogoproto.goproto_getters) = false;
|
|
|
|
string sender = 1;
|
|
repeated Selection denoms_to_claim = 2 [(gogoproto.castrepeated) = "Selections", (gogoproto.nullable) = false];
|
|
}
|
|
|
|
// MsgClaimHardRewardResponse defines the Msg/ClaimHardReward response type.
|
|
message MsgClaimHardRewardResponse {}
|
|
|
|
// MsgClaimDelegatorReward message type used to claim delegator rewards
|
|
message MsgClaimDelegatorReward {
|
|
option (gogoproto.equal) = false;
|
|
option (gogoproto.goproto_getters) = false;
|
|
|
|
string sender = 1;
|
|
repeated Selection denoms_to_claim = 2 [(gogoproto.castrepeated) = "Selections", (gogoproto.nullable) = false];
|
|
}
|
|
|
|
// MsgClaimDelegatorRewardResponse defines the Msg/ClaimDelegatorReward response type.
|
|
message MsgClaimDelegatorRewardResponse {}
|
|
|
|
// MsgClaimSwapReward message type used to claim delegator rewards
|
|
message MsgClaimSwapReward {
|
|
option (gogoproto.equal) = false;
|
|
option (gogoproto.goproto_getters) = false;
|
|
|
|
string sender = 1;
|
|
repeated Selection denoms_to_claim = 2 [(gogoproto.castrepeated) = "Selections", (gogoproto.nullable) = false];
|
|
}
|
|
|
|
// MsgClaimSwapRewardResponse defines the Msg/ClaimSwapReward response type.
|
|
message MsgClaimSwapRewardResponse {}
|
|
|
|
// MsgClaimSavingsReward message type used to claim savings rewards
|
|
message MsgClaimSavingsReward {
|
|
option (gogoproto.equal) = false;
|
|
option (gogoproto.goproto_getters) = false;
|
|
|
|
string sender = 1;
|
|
repeated Selection denoms_to_claim = 2 [(gogoproto.castrepeated) = "Selections", (gogoproto.nullable) = false];
|
|
}
|
|
|
|
// MsgClaimSavingsRewardResponse defines the Msg/ClaimSavingsReward response type.
|
|
message MsgClaimSavingsRewardResponse {}
|