mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 22:57:32 +00:00
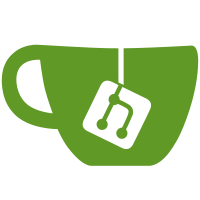
* Add 'InterestFactor' to CDP type (#734) * update cdp type to include interest factor * fix build * Add cdp accumulator methods (#735) * remame fees to interest * add accumulate interest method * add basic test * add note * address review comments * update tests * Add sync cdp interest method (#737) * remame fees to interest * add accumulate interest method * add basic test * add note * address review comments * update tests * remove old fee functions * add method to synchronize cdp interest * add multi-cdp tests * add test with many blocks * add test for interest getter * address review comments * calculate time difference then convert to seconds * fix: update collateral index when syncing interest * fix: differentiate between case when apy is zero and all fees are being rounded to zero * fix: round time difference properly * update cdp genesis state and migrations (#738) * remame fees to interest * add accumulate interest method * add basic test * add note * address review comments * update tests * remove old fee functions * add method to synchronize cdp interest * add multi-cdp tests * add test with many blocks * add test for interest getter * update cdp genesis state and migrations * address review comments * calculate time difference then convert to seconds * fix: update collateral index when syncing interest * fix: differentiate between case when apy is zero and all fees are being rounded to zero * fix: simplify add/remove/update collateral index * update genesis state to include total principal amounts * update migration * Delete kava-4-cdp-state-block-500000.json * Add cdp liquidations by external keeper (#750) * feat: split liquidations between external keepers and automated begin blocker * address review comments * USDX incentive accumulators (#752) * feat: split liquidations between external keepers and automated begin blocker * wip: refactor usdx minting incentives to use accumulators/hooks * wip: refactor usdx minting claim object * feat: use accumulators/hooks for usdx minting rewards * fix: get tests passing * fix: don't create claim objects unless that cdp type is eligable for rewards * add begin blocker * update client * cleanup comments/tests * update querier * address review comments * fix: check for division by zero * address review comments * run hook before interest is synced * Remove savings rate (#764) * remove savings rate * remove savings rate from debt param * update migrations * address review comments * Add usdx incentives calculation test (#765) * add usdx incentive calculation test * update reward calculation * add allowable error to test criteria * Update x/incentive/keeper/rewards_test.go Co-authored-by: Kevin Davis <karzak@users.noreply.github.com> * fix: remove old fields from test genesis state Co-authored-by: Ruaridh <rhuairahrighairidh@users.noreply.github.com> Co-authored-by: Ruaridh <rhuairahrighairidh@users.noreply.github.com>
173 lines
6.9 KiB
Go
173 lines
6.9 KiB
Go
package keeper
|
|
|
|
import (
|
|
"math"
|
|
"time"
|
|
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
|
|
cdptypes "github.com/kava-labs/kava/x/cdp/types"
|
|
"github.com/kava-labs/kava/x/incentive/types"
|
|
)
|
|
|
|
// AccumulateRewards updates the rewards accumulated for the input reward period
|
|
func (k Keeper) AccumulateRewards(ctx sdk.Context, rewardPeriod types.RewardPeriod) error {
|
|
if !rewardPeriod.Active {
|
|
k.SetPreviousAccrualTime(ctx, rewardPeriod.CollateralType, ctx.BlockTime())
|
|
return nil
|
|
}
|
|
previousAccrualTime, found := k.GetPreviousAccrualTime(ctx, rewardPeriod.CollateralType)
|
|
if !found {
|
|
k.SetPreviousAccrualTime(ctx, rewardPeriod.CollateralType, ctx.BlockTime())
|
|
return nil
|
|
}
|
|
timeElapsed := CalculateTimeElapsed(rewardPeriod, ctx.BlockTime(), previousAccrualTime)
|
|
if timeElapsed.IsZero() {
|
|
return nil
|
|
}
|
|
if rewardPeriod.RewardsPerSecond.Amount.IsZero() {
|
|
k.SetPreviousAccrualTime(ctx, rewardPeriod.CollateralType, ctx.BlockTime())
|
|
return nil
|
|
}
|
|
totalPrincipal := k.cdpKeeper.GetTotalPrincipal(ctx, rewardPeriod.CollateralType, types.PrincipalDenom).ToDec()
|
|
if totalPrincipal.IsZero() {
|
|
k.SetPreviousAccrualTime(ctx, rewardPeriod.CollateralType, ctx.BlockTime())
|
|
return nil
|
|
}
|
|
newRewards := timeElapsed.Mul(rewardPeriod.RewardsPerSecond.Amount)
|
|
cdpFactor, found := k.cdpKeeper.GetInterestFactor(ctx, rewardPeriod.CollateralType)
|
|
if !found {
|
|
k.SetPreviousAccrualTime(ctx, rewardPeriod.CollateralType, ctx.BlockTime())
|
|
return nil
|
|
}
|
|
rewardFactor := newRewards.ToDec().Mul(cdpFactor).Quo(totalPrincipal)
|
|
|
|
previousRewardFactor, found := k.GetRewardFactor(ctx, rewardPeriod.CollateralType)
|
|
if !found {
|
|
previousRewardFactor = sdk.ZeroDec()
|
|
}
|
|
newRewardFactor := previousRewardFactor.Add(rewardFactor)
|
|
k.SetRewardFactor(ctx, rewardPeriod.CollateralType, newRewardFactor)
|
|
k.SetPreviousAccrualTime(ctx, rewardPeriod.CollateralType, ctx.BlockTime())
|
|
return nil
|
|
}
|
|
|
|
// InitializeClaim creates or updates a claim such that no new rewards are accrued, but any existing rewards are not lost.
|
|
// this function should be called after a cdp is created. If a user previously had a cdp, then closed it, they shouldn't
|
|
// accrue rewards during the period the cdp was closed. By setting the reward factor to the current global reward factor,
|
|
// any unclaimed rewards are preserved, but no new rewards are added.
|
|
func (k Keeper) InitializeClaim(ctx sdk.Context, cdp cdptypes.CDP) {
|
|
_, found := k.GetRewardPeriod(ctx, cdp.Type)
|
|
if !found {
|
|
// this collateral type is not incentivized, do nothing
|
|
return
|
|
}
|
|
rewardFactor, found := k.GetRewardFactor(ctx, cdp.Type)
|
|
if !found {
|
|
rewardFactor = sdk.ZeroDec()
|
|
}
|
|
claim, found := k.GetClaim(ctx, cdp.Owner)
|
|
if !found { // this is the owner's first usdx minting reward claim
|
|
claim = types.NewUSDXMintingClaim(cdp.Owner, sdk.NewCoin(types.USDXMintingRewardDenom, sdk.ZeroInt()), types.RewardIndexes{types.NewRewardIndex(cdp.Type, rewardFactor)})
|
|
k.SetClaim(ctx, claim)
|
|
return
|
|
}
|
|
// the owner has an existing usdx minting reward claim
|
|
index, hasRewardIndex := claim.HasRewardIndex(cdp.Type)
|
|
if !hasRewardIndex { // this is the owner's first usdx minting reward for this collateral type
|
|
claim.RewardIndexes = append(claim.RewardIndexes, types.NewRewardIndex(cdp.Type, rewardFactor))
|
|
} else { // the owner has a previous usdx minting reward for this collateral type
|
|
claim.RewardIndexes[index] = types.NewRewardIndex(cdp.Type, rewardFactor)
|
|
}
|
|
k.SetClaim(ctx, claim)
|
|
}
|
|
|
|
// SynchronizeReward updates the claim object by adding any accumulated rewards and updating the reward index value.
|
|
// this should be called before a cdp is modified, immediately after the 'SynchronizeInterest' method is called in the cdp module
|
|
func (k Keeper) SynchronizeReward(ctx sdk.Context, cdp cdptypes.CDP) {
|
|
_, found := k.GetRewardPeriod(ctx, cdp.Type)
|
|
if !found {
|
|
// this collateral type is not incentivized, do nothing
|
|
return
|
|
}
|
|
|
|
globalRewardFactor, found := k.GetRewardFactor(ctx, cdp.Type)
|
|
if !found {
|
|
globalRewardFactor = sdk.ZeroDec()
|
|
}
|
|
claim, found := k.GetClaim(ctx, cdp.Owner)
|
|
if !found {
|
|
claim = types.NewUSDXMintingClaim(cdp.Owner, sdk.NewCoin(types.USDXMintingRewardDenom, sdk.ZeroInt()), types.RewardIndexes{types.NewRewardIndex(cdp.Type, globalRewardFactor)})
|
|
k.SetClaim(ctx, claim)
|
|
return
|
|
}
|
|
|
|
// the owner has an existing usdx minting reward claim
|
|
index, hasRewardIndex := claim.HasRewardIndex(cdp.Type)
|
|
if !hasRewardIndex { // this is the owner's first usdx minting reward for this collateral type
|
|
claim.RewardIndexes = append(claim.RewardIndexes, types.NewRewardIndex(cdp.Type, globalRewardFactor))
|
|
k.SetClaim(ctx, claim)
|
|
return
|
|
}
|
|
userRewardFactor := claim.RewardIndexes[index].RewardFactor
|
|
rewardsAccumulatedFactor := globalRewardFactor.Sub(userRewardFactor)
|
|
if rewardsAccumulatedFactor.IsZero() {
|
|
return
|
|
}
|
|
claim.RewardIndexes[index].RewardFactor = globalRewardFactor
|
|
newRewardsAmount := cdp.GetTotalPrincipal().Amount.ToDec().Quo(cdp.InterestFactor).Mul(rewardsAccumulatedFactor).RoundInt()
|
|
if newRewardsAmount.IsZero() {
|
|
k.SetClaim(ctx, claim)
|
|
return
|
|
}
|
|
newRewardsCoin := sdk.NewCoin(types.USDXMintingRewardDenom, newRewardsAmount)
|
|
claim.Reward = claim.Reward.Add(newRewardsCoin)
|
|
k.SetClaim(ctx, claim)
|
|
return
|
|
}
|
|
|
|
// ZeroClaim zeroes out the claim object's rewards and returns the updated claim object
|
|
func (k Keeper) ZeroClaim(ctx sdk.Context, claim types.USDXMintingClaim) types.USDXMintingClaim {
|
|
claim.Reward = sdk.NewCoin(claim.Reward.Denom, sdk.ZeroInt())
|
|
k.SetClaim(ctx, claim)
|
|
return claim
|
|
}
|
|
|
|
// SynchronizeClaim updates the claim object by adding any rewards that have accumulated.
|
|
// Returns the updated claim object
|
|
func (k Keeper) SynchronizeClaim(ctx sdk.Context, claim types.USDXMintingClaim) (types.USDXMintingClaim, error) {
|
|
for _, ri := range claim.RewardIndexes {
|
|
cdp, found := k.cdpKeeper.GetCdpByOwnerAndCollateralType(ctx, claim.Owner, ri.CollateralType)
|
|
if !found {
|
|
// if the cdp for this collateral type has been closed, no updates are needed
|
|
continue
|
|
}
|
|
claim = k.synchronizeRewardAndReturnClaim(ctx, cdp)
|
|
}
|
|
return claim, nil
|
|
}
|
|
|
|
// this function assumes a claim already exists, so don't call it if that's not the case
|
|
func (k Keeper) synchronizeRewardAndReturnClaim(ctx sdk.Context, cdp cdptypes.CDP) types.USDXMintingClaim {
|
|
k.SynchronizeReward(ctx, cdp)
|
|
claim, _ := k.GetClaim(ctx, cdp.Owner)
|
|
return claim
|
|
}
|
|
|
|
// CalculateTimeElapsed calculates the number of reward-eligible seconds that have passed since the previous
|
|
// time rewards were accrued, taking into account the end time of the reward period
|
|
func CalculateTimeElapsed(rewardPeriod types.RewardPeriod, blockTime time.Time, previousAccrualTime time.Time) sdk.Int {
|
|
if rewardPeriod.End.Before(blockTime) &&
|
|
(rewardPeriod.End.Before(previousAccrualTime) || rewardPeriod.End.Equal(previousAccrualTime)) {
|
|
return sdk.ZeroInt()
|
|
}
|
|
if rewardPeriod.End.Before(blockTime) {
|
|
return sdk.NewInt(int64(math.RoundToEven(
|
|
rewardPeriod.End.Sub(previousAccrualTime).Seconds(),
|
|
)))
|
|
}
|
|
return sdk.NewInt(int64(math.RoundToEven(
|
|
blockTime.Sub(previousAccrualTime).Seconds(),
|
|
)))
|
|
}
|