mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-11-10 18:15:19 +00:00
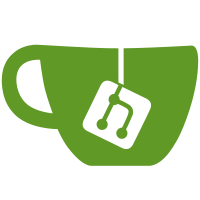
- Upgrade cosmos-sdk to v0.44.5 from v0.39.2 - Add Legacy Tx Endpoint for backwards compatibility - Add IBC v1.2.3 Support Co-authored-by: DracoLi <draco@dracoli.com> Co-authored-by: drklee3 <derrick@dlee.dev> Co-authored-by: denalimarsh <denalimarsh@gmail.com> Co-authored-by: Draco Li <draco@kava.io> Co-authored-by: Nick DeLuca <nickdeluca08@gmail.com> Co-authored-by: Kevin Davis <karzak@users.noreply.github.com> Co-authored-by: Denali Marsh <denali@kava.io>
56 lines
2.1 KiB
Go
56 lines
2.1 KiB
Go
package rest
|
|
|
|
import (
|
|
"github.com/gorilla/mux"
|
|
|
|
tmbytes "github.com/tendermint/tendermint/libs/bytes"
|
|
|
|
"github.com/cosmos/cosmos-sdk/client"
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
"github.com/cosmos/cosmos-sdk/types/rest"
|
|
)
|
|
|
|
// REST Variable names
|
|
// nolint
|
|
const (
|
|
RestExpiration = "expiration"
|
|
RestInvolve = "involve"
|
|
RestStatus = "status"
|
|
RestDirection = "direction"
|
|
)
|
|
|
|
// RegisterRoutes registers bep3-related REST handlers to a router
|
|
func RegisterRoutes(cliCtx client.Context, r *mux.Router) {
|
|
registerQueryRoutes(cliCtx, r)
|
|
registerTxRoutes(cliCtx, r)
|
|
}
|
|
|
|
// PostCreateSwapReq defines the properties of a swap create request's body
|
|
type PostCreateSwapReq struct {
|
|
BaseReq rest.BaseReq `json:"base_req" yaml:"base_req"`
|
|
From sdk.AccAddress `json:"from" yaml:"from"`
|
|
To sdk.AccAddress `json:"to" yaml:"to"`
|
|
RecipientOtherChain string `json:"recipient_other_chain" yaml:"recipient_other_chain"`
|
|
SenderOtherChain string `json:"sender_other_chain" yaml:"sender_other_chain"`
|
|
RandomNumberHash tmbytes.HexBytes `json:"random_number_hash" yaml:"random_number_hash"`
|
|
Timestamp int64 `json:"timestamp" yaml:"timestamp"`
|
|
Amount sdk.Coins `json:"amount" yaml:"amount"`
|
|
HeightSpan uint64 `json:"height_span" yaml:"height_span"`
|
|
CrossChain bool `json:"cross_chain" yaml:"cross_chain"`
|
|
}
|
|
|
|
// PostClaimSwapReq defines the properties of a swap claim request's body
|
|
type PostClaimSwapReq struct {
|
|
BaseReq rest.BaseReq `json:"base_req" yaml:"base_req"`
|
|
From sdk.AccAddress `json:"from" yaml:"from"`
|
|
SwapID tmbytes.HexBytes `json:"swap_id" yaml:"swap_id"`
|
|
RandomNumber tmbytes.HexBytes `json:"random_number" yaml:"random_number"`
|
|
}
|
|
|
|
// PostRefundSwapReq defines the properties of swap refund request's body
|
|
type PostRefundSwapReq struct {
|
|
BaseReq rest.BaseReq `json:"base_req" yaml:"base_req"`
|
|
From sdk.AccAddress `json:"from" yaml:"from"`
|
|
SwapID tmbytes.HexBytes `json:"swap_id" yaml:"swap_id"`
|
|
}
|