mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 14:47:31 +00:00
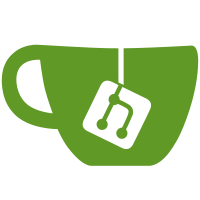
* add message types for swaps * add tx client commands * add test coverage for swap message deadlines * start handler swap tests, export handler result message event into private method, add stubbed keeper methods * add initial swap implementation to get handler tests passing; adds event specific for trades * add handler acceptance test for slippage in exact input and exact output swaps * implement slippage limit for swap keeper methods * add tests to ensure a user can only swap spendable coins * test pool not found, panic on invalid pool, and panic when module account does not have enough funds * validate that the exact output when using for exact swaps is less than the pool liquidity * nit: long line * add validation that swap output is greater than zero * add rest txs for swap messages * nit: lints * dry up swap keeper methods * from pr feedback - spelling and increase clairty around the output amount of a swap rounding to zero
107 lines
3.4 KiB
Go
107 lines
3.4 KiB
Go
package rest
|
|
|
|
import (
|
|
"fmt"
|
|
"net/http"
|
|
|
|
"github.com/gorilla/mux"
|
|
|
|
"github.com/cosmos/cosmos-sdk/client/context"
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
"github.com/cosmos/cosmos-sdk/types/rest"
|
|
"github.com/cosmos/cosmos-sdk/x/auth/client/utils"
|
|
|
|
"github.com/kava-labs/kava/x/swap/types"
|
|
)
|
|
|
|
func registerTxRoutes(cliCtx context.CLIContext, r *mux.Router) {
|
|
r.HandleFunc(fmt.Sprintf("/%s/deposit", types.ModuleName), postDepositHandlerFn(cliCtx)).Methods("POST")
|
|
r.HandleFunc(fmt.Sprintf("/%s/withdraw", types.ModuleName), postWithdrawHandlerFn(cliCtx)).Methods("POST")
|
|
r.HandleFunc(fmt.Sprintf("/%s/swapExactForTokens", types.ModuleName), postSwapExactForTokensHandlerFn(cliCtx)).Methods("POST")
|
|
r.HandleFunc(fmt.Sprintf("/%s/swapForExactTokens", types.ModuleName), postSwapForExactTokensHandlerFn(cliCtx)).Methods("POST")
|
|
}
|
|
|
|
func postDepositHandlerFn(cliCtx context.CLIContext) http.HandlerFunc {
|
|
return func(w http.ResponseWriter, r *http.Request) {
|
|
// Decode POST request body
|
|
var req PostCreateDepositReq
|
|
if !rest.ReadRESTReq(w, r, cliCtx.Codec, &req) {
|
|
return
|
|
}
|
|
req.BaseReq = req.BaseReq.Sanitize()
|
|
if !req.BaseReq.ValidateBasic(w) {
|
|
return
|
|
}
|
|
|
|
msg := types.NewMsgDeposit(req.From, req.TokenA, req.TokenB, req.Slippage, req.Deadline)
|
|
if err := msg.ValidateBasic(); err != nil {
|
|
rest.WriteErrorResponse(w, http.StatusBadRequest, err.Error())
|
|
return
|
|
}
|
|
utils.WriteGenerateStdTxResponse(w, cliCtx, req.BaseReq, []sdk.Msg{msg})
|
|
}
|
|
}
|
|
|
|
func postWithdrawHandlerFn(cliCtx context.CLIContext) http.HandlerFunc {
|
|
return func(w http.ResponseWriter, r *http.Request) {
|
|
// Decode POST request body
|
|
var req PostCreateWithdrawReq
|
|
if !rest.ReadRESTReq(w, r, cliCtx.Codec, &req) {
|
|
return
|
|
}
|
|
req.BaseReq = req.BaseReq.Sanitize()
|
|
if !req.BaseReq.ValidateBasic(w) {
|
|
return
|
|
}
|
|
|
|
msg := types.NewMsgWithdraw(req.From, req.Shares, req.MinTokenA, req.MinTokenA, req.Deadline)
|
|
if err := msg.ValidateBasic(); err != nil {
|
|
rest.WriteErrorResponse(w, http.StatusBadRequest, err.Error())
|
|
return
|
|
}
|
|
utils.WriteGenerateStdTxResponse(w, cliCtx, req.BaseReq, []sdk.Msg{msg})
|
|
}
|
|
}
|
|
|
|
func postSwapExactForTokensHandlerFn(cliCtx context.CLIContext) http.HandlerFunc {
|
|
return func(w http.ResponseWriter, r *http.Request) {
|
|
// Decode POST request body
|
|
var req PostCreateSwapExactForTokensReq
|
|
if !rest.ReadRESTReq(w, r, cliCtx.Codec, &req) {
|
|
return
|
|
}
|
|
req.BaseReq = req.BaseReq.Sanitize()
|
|
if !req.BaseReq.ValidateBasic(w) {
|
|
return
|
|
}
|
|
|
|
msg := types.NewMsgSwapExactForTokens(req.Requester, req.ExactTokenA, req.TokenB, req.Slippage, req.Deadline)
|
|
if err := msg.ValidateBasic(); err != nil {
|
|
rest.WriteErrorResponse(w, http.StatusBadRequest, err.Error())
|
|
return
|
|
}
|
|
utils.WriteGenerateStdTxResponse(w, cliCtx, req.BaseReq, []sdk.Msg{msg})
|
|
}
|
|
}
|
|
|
|
func postSwapForExactTokensHandlerFn(cliCtx context.CLIContext) http.HandlerFunc {
|
|
return func(w http.ResponseWriter, r *http.Request) {
|
|
// Decode POST request body
|
|
var req PostCreateSwapForExactTokensReq
|
|
if !rest.ReadRESTReq(w, r, cliCtx.Codec, &req) {
|
|
return
|
|
}
|
|
req.BaseReq = req.BaseReq.Sanitize()
|
|
if !req.BaseReq.ValidateBasic(w) {
|
|
return
|
|
}
|
|
|
|
msg := types.NewMsgSwapForExactTokens(req.Requester, req.TokenA, req.ExactTokenB, req.Slippage, req.Deadline)
|
|
if err := msg.ValidateBasic(); err != nil {
|
|
rest.WriteErrorResponse(w, http.StatusBadRequest, err.Error())
|
|
return
|
|
}
|
|
utils.WriteGenerateStdTxResponse(w, cliCtx, req.BaseReq, []sdk.Msg{msg})
|
|
}
|
|
}
|