mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 14:47:31 +00:00
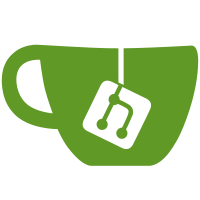
* feat: use only module account for bep3 txs * wip: add time-based supply limits * add tests and sims * update genesis tests * fix migration, committee tests * update migrations * fix: set previous block time in begin block * update store decoder * add additional bep3 params to committee * revert incorrect rebase changes * add migration test * address review comments
67 lines
2.1 KiB
Go
67 lines
2.1 KiB
Go
package simulation
|
|
|
|
import (
|
|
"fmt"
|
|
"testing"
|
|
"time"
|
|
|
|
"github.com/stretchr/testify/require"
|
|
|
|
tmbytes "github.com/tendermint/tendermint/libs/bytes"
|
|
"github.com/tendermint/tendermint/libs/kv"
|
|
|
|
"github.com/cosmos/cosmos-sdk/codec"
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
|
|
"github.com/kava-labs/kava/x/bep3/types"
|
|
)
|
|
|
|
func makeTestCodec() (cdc *codec.Codec) {
|
|
cdc = codec.New()
|
|
sdk.RegisterCodec(cdc)
|
|
types.RegisterCodec(cdc)
|
|
return
|
|
}
|
|
|
|
func TestDecodeBep3Store(t *testing.T) {
|
|
cdc := makeTestCodec()
|
|
prevBlockTime := time.Now().UTC()
|
|
|
|
oneCoin := sdk.NewCoin("coin", sdk.OneInt())
|
|
swap := types.NewAtomicSwap(sdk.Coins{oneCoin}, nil, 10, 100, nil, nil, "otherChainSender", "otherChainRec", 200, types.Completed, true, types.Outgoing)
|
|
supply := types.AssetSupply{IncomingSupply: oneCoin, OutgoingSupply: oneCoin, CurrentSupply: oneCoin, TimeLimitedCurrentSupply: oneCoin, TimeElapsed: time.Duration(0)}
|
|
bz := tmbytes.HexBytes([]byte{1, 2})
|
|
|
|
kvPairs := kv.Pairs{
|
|
kv.Pair{Key: types.AtomicSwapKeyPrefix, Value: cdc.MustMarshalBinaryLengthPrefixed(swap)},
|
|
kv.Pair{Key: types.AssetSupplyPrefix, Value: cdc.MustMarshalBinaryLengthPrefixed(supply)},
|
|
kv.Pair{Key: types.AtomicSwapByBlockPrefix, Value: bz},
|
|
kv.Pair{Key: types.AtomicSwapByBlockPrefix, Value: bz},
|
|
kv.Pair{Key: types.PreviousBlockTimeKey, Value: cdc.MustMarshalBinaryLengthPrefixed(prevBlockTime)},
|
|
kv.Pair{Key: []byte{0x99}, Value: []byte{0x99}},
|
|
}
|
|
|
|
tests := []struct {
|
|
name string
|
|
expectedLog string
|
|
}{
|
|
{"AtomicSwap", fmt.Sprintf("%v\n%v", swap, swap)},
|
|
{"AssetSupply", fmt.Sprintf("%v\n%v", supply, supply)},
|
|
{"AtomicSwapByBlock", fmt.Sprintf("%s\n%s", bz, bz)},
|
|
{"AtomicSwapLongtermStorage", fmt.Sprintf("%s\n%s", bz, bz)},
|
|
{"PreviousBlockTime", fmt.Sprintf("%s\n%s", prevBlockTime, prevBlockTime)},
|
|
{"other", ""},
|
|
}
|
|
for i, tt := range tests {
|
|
i, tt := i, tt
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
switch i {
|
|
case len(tests) - 1:
|
|
require.Panics(t, func() { DecodeStore(cdc, kvPairs[i], kvPairs[i]) }, tt.name)
|
|
default:
|
|
require.Equal(t, tt.expectedLog, DecodeStore(cdc, kvPairs[i], kvPairs[i]), tt.name)
|
|
}
|
|
})
|
|
}
|
|
}
|