mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 14:47:31 +00:00
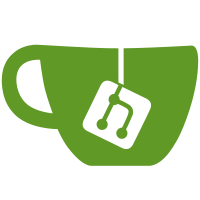
* add proto for allowed sdk denoms -> evm conversion * add validation for AllowedNativeCoinERC20Token * add validation for AllowedNativeCoinERC20Tokens * add AllowedNativeDenoms into params & genesis * add evmutil Params.Validate() test * fix eip712 ante test * update changelog * update internal testnet genesis.json * update state & param specs updates to the sections describing functionality will be updated once that functionality actually exists... :) * update field decimal -> decimals field now matches erc20 spec * add validation decimals will cast to uint8 * add v2 store migration for evmutil * create & register evmutil migrations * adds migrator to evmutil's keeper * sets up Migrate1To2 migration * registers migration in module * updates GetParams to properly handle historic block queries * add unit test for GetParams with historic store
168 lines
5.2 KiB
Go
168 lines
5.2 KiB
Go
package evmutil
|
|
|
|
import (
|
|
"context"
|
|
"encoding/json"
|
|
|
|
"github.com/grpc-ecosystem/grpc-gateway/runtime"
|
|
"github.com/spf13/cobra"
|
|
|
|
abci "github.com/tendermint/tendermint/abci/types"
|
|
|
|
"github.com/cosmos/cosmos-sdk/client"
|
|
"github.com/cosmos/cosmos-sdk/codec"
|
|
cdctypes "github.com/cosmos/cosmos-sdk/codec/types"
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
"github.com/cosmos/cosmos-sdk/types/module"
|
|
|
|
"github.com/kava-labs/kava/x/evmutil/client/cli"
|
|
"github.com/kava-labs/kava/x/evmutil/keeper"
|
|
"github.com/kava-labs/kava/x/evmutil/types"
|
|
)
|
|
|
|
// ConsensusVersion defines the current module consensus version.
|
|
const ConsensusVersion = 2
|
|
|
|
var (
|
|
_ module.AppModule = AppModule{}
|
|
_ module.AppModuleBasic = AppModuleBasic{}
|
|
)
|
|
|
|
// ----------------------------------------------------------------------------
|
|
// AppModuleBasic
|
|
// ----------------------------------------------------------------------------
|
|
|
|
// AppModuleBasic app module basics object
|
|
type AppModuleBasic struct{}
|
|
|
|
func NewAppModuleBasic() AppModuleBasic {
|
|
return AppModuleBasic{}
|
|
}
|
|
|
|
// Name get module name
|
|
func (AppModuleBasic) Name() string {
|
|
return types.ModuleName
|
|
}
|
|
|
|
// Registers legacy amino codec
|
|
func (AppModuleBasic) RegisterLegacyAminoCodec(cdc *codec.LegacyAmino) {}
|
|
|
|
// RegisterInterfaces registers the module's interface types
|
|
func (a AppModuleBasic) RegisterInterfaces(reg cdctypes.InterfaceRegistry) {
|
|
types.RegisterInterfaces(reg)
|
|
}
|
|
|
|
// DefaultGenesis default genesis state
|
|
func (AppModuleBasic) DefaultGenesis(cdc codec.JSONCodec) json.RawMessage {
|
|
gs := types.DefaultGenesisState()
|
|
return cdc.MustMarshalJSON(gs)
|
|
}
|
|
|
|
// ValidateGenesis module validate genesis
|
|
func (AppModuleBasic) ValidateGenesis(cdc codec.JSONCodec, config client.TxEncodingConfig, bz json.RawMessage) error {
|
|
var gs types.GenesisState
|
|
err := cdc.UnmarshalJSON(bz, &gs)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
return gs.Validate()
|
|
}
|
|
|
|
// RegisterGRPCGatewayRoutes registers the gRPC Gateway routes for evmutil module.
|
|
func (AppModuleBasic) RegisterGRPCGatewayRoutes(clientCtx client.Context, mux *runtime.ServeMux) {
|
|
if err := types.RegisterQueryHandlerClient(context.Background(), mux, types.NewQueryClient(clientCtx)); err != nil {
|
|
panic(err)
|
|
}
|
|
}
|
|
|
|
// GetTxCmd returns evmutil module's root tx command.
|
|
func (a AppModuleBasic) GetTxCmd() *cobra.Command {
|
|
return cli.GetTxCmd()
|
|
}
|
|
|
|
// GetQueryCmd returns evmutil module's root query command.
|
|
func (AppModuleBasic) GetQueryCmd() *cobra.Command {
|
|
return cli.GetQueryCmd()
|
|
}
|
|
|
|
// ----------------------------------------------------------------------------
|
|
// AppModule
|
|
// ----------------------------------------------------------------------------
|
|
|
|
// AppModule implements the AppModule interface for evmutil module.
|
|
type AppModule struct {
|
|
AppModuleBasic
|
|
|
|
keeper keeper.Keeper
|
|
bankKeeper types.BankKeeper
|
|
}
|
|
|
|
// NewAppModule creates a new AppModule object
|
|
func NewAppModule(keeper keeper.Keeper, bankKeeper types.BankKeeper) AppModule {
|
|
return AppModule{
|
|
AppModuleBasic: NewAppModuleBasic(),
|
|
keeper: keeper,
|
|
bankKeeper: bankKeeper,
|
|
}
|
|
}
|
|
|
|
// Name returns evmutil module's name.
|
|
func (am AppModule) Name() string {
|
|
return am.AppModuleBasic.Name()
|
|
}
|
|
|
|
// Route returns evmutil module's message route.
|
|
func (am AppModule) Route() sdk.Route { return sdk.Route{} }
|
|
|
|
// QuerierRoute returns evmutil module's query routing key.
|
|
func (AppModule) QuerierRoute() string { return "" }
|
|
|
|
// LegacyQuerierHandler returns evmutil module's Querier.
|
|
func (am AppModule) LegacyQuerierHandler(legacyQuerierCdc *codec.LegacyAmino) sdk.Querier {
|
|
return nil
|
|
}
|
|
|
|
// RegisterServices registers a GRPC query service to respond to the
|
|
// module-specific GRPC queries.
|
|
func (am AppModule) RegisterServices(cfg module.Configurator) {
|
|
types.RegisterMsgServer(cfg.MsgServer(), keeper.NewMsgServerImpl(am.keeper))
|
|
types.RegisterQueryServer(cfg.QueryServer(), keeper.NewQueryServerImpl(am.keeper))
|
|
|
|
m := keeper.NewMigrator(am.keeper)
|
|
cfg.RegisterMigration(types.ModuleName, 1, m.Migrate1to2)
|
|
}
|
|
|
|
// RegisterInvariants registers evmutil module's invariants.
|
|
func (am AppModule) RegisterInvariants(ir sdk.InvariantRegistry) {
|
|
keeper.RegisterInvariants(ir, am.bankKeeper, am.keeper)
|
|
}
|
|
|
|
// InitGenesis performs evmutil module's genesis initialization It returns
|
|
// no validator updates.
|
|
func (am AppModule) InitGenesis(ctx sdk.Context, cdc codec.JSONCodec, gs json.RawMessage) []abci.ValidatorUpdate {
|
|
var genState types.GenesisState
|
|
// Initialize global index to index in genesis state
|
|
cdc.MustUnmarshalJSON(gs, &genState)
|
|
|
|
InitGenesis(ctx, am.keeper, &genState)
|
|
return []abci.ValidatorUpdate{}
|
|
}
|
|
|
|
// ExportGenesis returns evmutil module's exported genesis state as raw JSON bytes.
|
|
func (am AppModule) ExportGenesis(ctx sdk.Context, cdc codec.JSONCodec) json.RawMessage {
|
|
gs := ExportGenesis(ctx, am.keeper)
|
|
return cdc.MustMarshalJSON(gs)
|
|
}
|
|
|
|
// ConsensusVersion implements ConsensusVersion.
|
|
func (AppModule) ConsensusVersion() uint64 { return ConsensusVersion }
|
|
|
|
// BeginBlock executes all ABCI BeginBlock logic respective to evmutil module.
|
|
func (am AppModule) BeginBlock(ctx sdk.Context, _ abci.RequestBeginBlock) {}
|
|
|
|
// EndBlock executes all ABCI EndBlock logic respective to evmutil module. It
|
|
// returns no validator updates.
|
|
func (am AppModule) EndBlock(_ sdk.Context, _ abci.RequestEndBlock) []abci.ValidatorUpdate {
|
|
return []abci.ValidatorUpdate{}
|
|
}
|