mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 14:47:31 +00:00
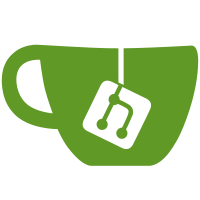
* spike: incentive/types * spike: incentive/types tests * spike: incentive/types/expected_keepers.go * spike: incentive/keeper * spike: incentive/keeper tests * spike: incentive/sims and incentive/sims tests * spike: incentive/module * spike: incentive/module tests * spike: hard/types * spike: hard/types hooks * spike: hard/types * spike: hard/keeper basics * spike: hard/keeper hooks * integrate hard/keeper/borrow.go * integrate hard/keeper/deposit.go * integrate hard/keeper/liquidation.go * integrate hard/keeper/withdraw.go * integrate hard/keeper/repay.go * spike: hard/sims * spike: hard/sims tests * spike: hard/client * spike: hard/module * integrate app.go * spike: x/hard/keeper compile tests * incentive/keeper test clean up * validate usdx incentive types in genesis * refactoring & fix deposit test * fix liquidaton tests * fix incentive tests for hard supply rewards * fix hard genesis tests * update incentive genesis state and params * update cdp rewards accumulation * update app init order and begin blocker order Co-authored-by: karzak <kjydavis3@gmail.com>
70 lines
2.0 KiB
Go
70 lines
2.0 KiB
Go
package incentive
|
|
|
|
import (
|
|
"fmt"
|
|
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
|
|
"github.com/kava-labs/kava/x/incentive/keeper"
|
|
"github.com/kava-labs/kava/x/incentive/types"
|
|
)
|
|
|
|
// InitGenesis initializes the store state from a genesis state.
|
|
func InitGenesis(ctx sdk.Context, k keeper.Keeper, supplyKeeper types.SupplyKeeper, cdpKeeper types.CdpKeeper, gs types.GenesisState) {
|
|
|
|
// check if the module account exists
|
|
moduleAcc := supplyKeeper.GetModuleAccount(ctx, types.IncentiveMacc)
|
|
if moduleAcc == nil {
|
|
panic(fmt.Sprintf("%s module account has not been set", types.IncentiveMacc))
|
|
}
|
|
|
|
if err := gs.Validate(); err != nil {
|
|
panic(fmt.Sprintf("failed to validate %s genesis state: %s", types.ModuleName, err))
|
|
}
|
|
|
|
for _, rp := range gs.Params.USDXMintingRewardPeriods {
|
|
_, found := cdpKeeper.GetCollateral(ctx, rp.CollateralType)
|
|
if !found {
|
|
panic(fmt.Sprintf("usdx minting collateral type %s not found in cdp collateral types", rp.CollateralType))
|
|
}
|
|
}
|
|
|
|
k.SetParams(ctx, gs.Params)
|
|
|
|
// TODO: previous hard module accrual times/indexes should be set here
|
|
|
|
for _, gat := range gs.PreviousAccumulationTimes {
|
|
k.SetPreviousUSDXMintingAccrualTime(ctx, gat.CollateralType, gat.PreviousAccumulationTime)
|
|
k.SetUSDXMintingRewardFactor(ctx, gat.CollateralType, gat.RewardFactor)
|
|
}
|
|
|
|
for _, claim := range gs.USDXMintingClaims {
|
|
k.SetUSDXMintingClaim(ctx, claim)
|
|
}
|
|
|
|
}
|
|
|
|
// ExportGenesis export genesis state for incentive module
|
|
func ExportGenesis(ctx sdk.Context, k keeper.Keeper) types.GenesisState {
|
|
params := k.GetParams(ctx)
|
|
|
|
claims := k.GetAllUSDXMintingClaims(ctx)
|
|
|
|
var gats GenesisAccumulationTimes
|
|
|
|
for _, rp := range params.USDXMintingRewardPeriods {
|
|
pat, found := k.GetPreviousUSDXMintingAccrualTime(ctx, rp.CollateralType)
|
|
if !found {
|
|
pat = ctx.BlockTime()
|
|
}
|
|
factor, found := k.GetUSDXMintingRewardFactor(ctx, rp.CollateralType)
|
|
if !found {
|
|
factor = sdk.ZeroDec()
|
|
}
|
|
gat := types.NewGenesisAccumulationTime(rp.CollateralType, pat, factor)
|
|
gats = append(gats, gat)
|
|
}
|
|
|
|
return types.NewGenesisState(params, gats, claims)
|
|
}
|