mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 14:47:31 +00:00
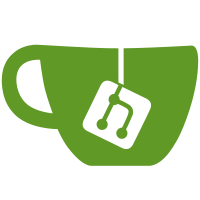
- Upgrade cosmos-sdk to v0.44.5 from v0.39.2 - Add Legacy Tx Endpoint for backwards compatibility - Add IBC v1.2.3 Support Co-authored-by: DracoLi <draco@dracoli.com> Co-authored-by: drklee3 <derrick@dlee.dev> Co-authored-by: denalimarsh <denalimarsh@gmail.com> Co-authored-by: Draco Li <draco@kava.io> Co-authored-by: Nick DeLuca <nickdeluca08@gmail.com> Co-authored-by: Kevin Davis <karzak@users.noreply.github.com> Co-authored-by: Denali Marsh <denali@kava.io>
50 lines
1.7 KiB
Go
50 lines
1.7 KiB
Go
package rest
|
|
|
|
import (
|
|
"github.com/gorilla/mux"
|
|
|
|
"github.com/cosmos/cosmos-sdk/client"
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
"github.com/cosmos/cosmos-sdk/types/rest"
|
|
)
|
|
|
|
// RegisterRoutes - Central function to define routes that get registered by the main application
|
|
func RegisterRoutes(cliCtx client.Context, r *mux.Router) {
|
|
registerQueryRoutes(cliCtx, r)
|
|
registerTxRoutes(cliCtx, r)
|
|
}
|
|
|
|
// PostIssueReq defines the properties of an issue token request's body
|
|
type PostIssueReq struct {
|
|
BaseReq rest.BaseReq `json:"base_req" yaml:"base_req"`
|
|
Tokens sdk.Coin `json:"tokens" yaml:"tokens"`
|
|
Receiver sdk.AccAddress `json:"receiver" yaml:"receiver"`
|
|
}
|
|
|
|
// PostRedeemReq defines the properties of a redeem token request's body
|
|
type PostRedeemReq struct {
|
|
BaseReq rest.BaseReq `json:"base_req" yaml:"base_req"`
|
|
Tokens sdk.Coin `json:"tokens" yaml:"tokens"`
|
|
}
|
|
|
|
// PostBlockAddressReq defines the properties of a block address request's body
|
|
type PostBlockAddressReq struct {
|
|
BaseReq rest.BaseReq `json:"base_req" yaml:"base_req"`
|
|
Address sdk.AccAddress `json:"blocked_address" yaml:"blocked_address"`
|
|
Denom string `json:"denom" yaml:"denom"`
|
|
}
|
|
|
|
// PostUnblockAddressReq defines the properties of a unblock address request's body
|
|
type PostUnblockAddressReq struct {
|
|
BaseReq rest.BaseReq `json:"base_req" yaml:"base_req"`
|
|
Address sdk.AccAddress `json:"blocked_address" yaml:"blocked_address"`
|
|
Denom string `json:"denom" yaml:"denom"`
|
|
}
|
|
|
|
// PostPauseReq defines the properties of a pause request's body
|
|
type PostPauseReq struct {
|
|
BaseReq rest.BaseReq `json:"base_req" yaml:"base_req"`
|
|
Denom string `json:"denom" yaml:"denom"`
|
|
Status bool `json:"status" yaml:"status"`
|
|
}
|