mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 14:47:31 +00:00
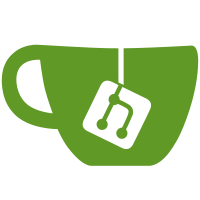
* update claim attribute type to MultiRewardIndexes * update param attribute type to MultiRewardPeriods * keeper: update params to match types * keeper: update delegator core keeper methods * keeper: update InitializeHardDelegatorReward * keeper: update SynchronizeHardDelegatorRewards * remove reward factor in favor of reward indexes * update querier * fix test: delegator init test * fix test: delegator sync test * implement delegator reward accumulation * fix test: delegator general tests * add legact types, update v0_11 -> v0_14 migration * remove duplicate import form v0_15 migration * implement v0_15incentive migration * test data and migration test * add multiple reward denoms to init/sync tests * update delegator test with multiple reward coins * clean up simulation sync * types: introduce DelegatorClaim, refactor HardClaim * add core DelegateClaim store methods * refactor delegator reward init, accumulation, sync * update hooks * update params and genesis logic * update abci * update types tests * update querier types/keeper for compile * update supply rewards tests * update borrow reward tests * update delegator reward tests * update handler/genesis test for compile * add new msg type * implement delegator claim payouts * submission + handling of new msg * implement new querier types/keeper logic * add new queries to cli/rest * update migration * register new msgs/types on codec * remove delegator syncing from hard sync method
120 lines
4.0 KiB
Go
120 lines
4.0 KiB
Go
package keeper_test
|
|
|
|
import (
|
|
"testing"
|
|
|
|
"github.com/stretchr/testify/suite"
|
|
|
|
hardtypes "github.com/kava-labs/kava/x/hard/types"
|
|
"github.com/kava-labs/kava/x/incentive/types"
|
|
)
|
|
|
|
// UpdateHardBorrowIndexDenomsTests runs unit tests for the keeper.UpdateHardBorrowIndexDenoms method
|
|
//
|
|
// inputs
|
|
// - claim in store if it exists (only claim.BorrowRewardIndexes)
|
|
// - global indexes in store
|
|
// - borrow function arg (only borrow.Amount)
|
|
//
|
|
// outputs
|
|
// - sets a claim
|
|
type UpdateHardBorrowIndexDenomsTests struct {
|
|
unitTester
|
|
}
|
|
|
|
func TestUpdateHardBorrowIndexDenoms(t *testing.T) {
|
|
suite.Run(t, new(UpdateHardBorrowIndexDenomsTests))
|
|
}
|
|
|
|
func (suite *UpdateHardBorrowIndexDenomsTests) TestClaimIndexesAreRemovedForDenomsNoLongerBorrowed() {
|
|
claim := types.HardLiquidityProviderClaim{
|
|
BaseMultiClaim: types.BaseMultiClaim{
|
|
Owner: arbitraryAddress(),
|
|
},
|
|
BorrowRewardIndexes: nonEmptyMultiRewardIndexes,
|
|
}
|
|
suite.storeHardClaim(claim)
|
|
suite.storeGlobalBorrowIndexes(claim.BorrowRewardIndexes)
|
|
|
|
// remove one denom from the indexes already in the borrow
|
|
expectedIndexes := claim.BorrowRewardIndexes[1:]
|
|
borrow := hardtypes.Borrow{
|
|
Borrower: claim.Owner,
|
|
Amount: arbitraryCoinsWithDenoms(extractCollateralTypes(expectedIndexes)...),
|
|
}
|
|
|
|
suite.keeper.UpdateHardBorrowIndexDenoms(suite.ctx, borrow)
|
|
|
|
syncedClaim, _ := suite.keeper.GetHardLiquidityProviderClaim(suite.ctx, claim.Owner)
|
|
suite.Equal(expectedIndexes, syncedClaim.BorrowRewardIndexes)
|
|
}
|
|
|
|
func (suite *UpdateHardBorrowIndexDenomsTests) TestClaimIndexesAreAddedForNewlyBorrowedDenoms() {
|
|
claim := types.HardLiquidityProviderClaim{
|
|
BaseMultiClaim: types.BaseMultiClaim{
|
|
Owner: arbitraryAddress(),
|
|
},
|
|
BorrowRewardIndexes: nonEmptyMultiRewardIndexes,
|
|
}
|
|
suite.storeHardClaim(claim)
|
|
globalIndexes := appendUniqueMultiRewardIndex(claim.BorrowRewardIndexes)
|
|
suite.storeGlobalBorrowIndexes(globalIndexes)
|
|
|
|
borrow := hardtypes.Borrow{
|
|
Borrower: claim.Owner,
|
|
Amount: arbitraryCoinsWithDenoms(extractCollateralTypes(globalIndexes)...),
|
|
}
|
|
|
|
suite.keeper.UpdateHardBorrowIndexDenoms(suite.ctx, borrow)
|
|
|
|
syncedClaim, _ := suite.keeper.GetHardLiquidityProviderClaim(suite.ctx, claim.Owner)
|
|
suite.Equal(globalIndexes, syncedClaim.BorrowRewardIndexes)
|
|
}
|
|
|
|
func (suite *UpdateHardBorrowIndexDenomsTests) TestClaimIndexesAreUnchangedWhenBorrowedDenomsUnchanged() {
|
|
claim := types.HardLiquidityProviderClaim{
|
|
BaseMultiClaim: types.BaseMultiClaim{
|
|
Owner: arbitraryAddress(),
|
|
},
|
|
BorrowRewardIndexes: nonEmptyMultiRewardIndexes,
|
|
}
|
|
suite.storeHardClaim(claim)
|
|
// Set global indexes with same denoms but different values.
|
|
// UpdateHardBorrowIndexDenoms should ignore the new values.
|
|
suite.storeGlobalBorrowIndexes(increaseAllRewardFactors(claim.BorrowRewardIndexes))
|
|
|
|
borrow := hardtypes.Borrow{
|
|
Borrower: claim.Owner,
|
|
Amount: arbitraryCoinsWithDenoms(extractCollateralTypes(claim.BorrowRewardIndexes)...),
|
|
}
|
|
|
|
suite.keeper.UpdateHardBorrowIndexDenoms(suite.ctx, borrow)
|
|
|
|
syncedClaim, _ := suite.keeper.GetHardLiquidityProviderClaim(suite.ctx, claim.Owner)
|
|
suite.Equal(claim.BorrowRewardIndexes, syncedClaim.BorrowRewardIndexes)
|
|
}
|
|
|
|
func (suite *UpdateHardBorrowIndexDenomsTests) TestEmptyClaimIndexesAreAddedForNewlyBorrowedButNotRewardedDenoms() {
|
|
claim := types.HardLiquidityProviderClaim{
|
|
BaseMultiClaim: types.BaseMultiClaim{
|
|
Owner: arbitraryAddress(),
|
|
},
|
|
BorrowRewardIndexes: nonEmptyMultiRewardIndexes,
|
|
}
|
|
suite.storeHardClaim(claim)
|
|
suite.storeGlobalBorrowIndexes(claim.BorrowRewardIndexes)
|
|
|
|
// add a denom to the borrowed amount that is not in the global or claim's indexes
|
|
expectedIndexes := appendUniqueEmptyMultiRewardIndex(claim.BorrowRewardIndexes)
|
|
borrowedDenoms := extractCollateralTypes(expectedIndexes)
|
|
borrow := hardtypes.Borrow{
|
|
Borrower: claim.Owner,
|
|
Amount: arbitraryCoinsWithDenoms(borrowedDenoms...),
|
|
}
|
|
|
|
suite.keeper.UpdateHardBorrowIndexDenoms(suite.ctx, borrow)
|
|
|
|
syncedClaim, _ := suite.keeper.GetHardLiquidityProviderClaim(suite.ctx, claim.Owner)
|
|
suite.Equal(expectedIndexes, syncedClaim.BorrowRewardIndexes)
|
|
}
|