mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 14:47:31 +00:00
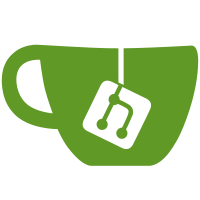
* initial sketch * add module migrations * add migrations for all accout types * test account migration * add tendermint migration and migrate cmd * remove need for errors pkg dependency * add bech32 decoding fork * add suggested params and cmd to write them * add basic upgrade instructions * fix tests * address some migration todos * tidy contrib folder * finalize params values * align cdp init genesis with other modules * add tendermint and distribution test add custom distribution migration to patch bug * add staking migration test * add slashing, evidence tests, refactor auth tests * add full migration test * remove go-amino dependency from go.mod also tidy up unused indirect dependencies * address remaining TODOs * remove commented out code from legacy types * add spot/liquidation markets ids to kava-3 params * Apply suggestions from code review Co-authored-by: Alexander Bezobchuk <alexanderbez@users.noreply.github.com> Co-authored-by: Federico Kunze <31522760+fedekunze@users.noreply.github.com> * address code review suggestions * add validate genesis to migrate test * refactor add params func * remove commented out code from old types * fix add params * add deputy address * add tests using exported kava-2 state * incorporate new cdp params from master * update params from review Co-authored-by: Kevin Davis <karzak@users.noreply.github.com> * add deputy account * add committee permissions for new params Co-authored-by: Alexander Bezobchuk <alexanderbez@users.noreply.github.com> Co-authored-by: Federico Kunze <31522760+fedekunze@users.noreply.github.com> Co-authored-by: Kevin Davis <karzak@users.noreply.github.com>
193 lines
6.3 KiB
Go
193 lines
6.3 KiB
Go
package v0_8
|
|
|
|
import (
|
|
"io/ioutil"
|
|
"os"
|
|
"path/filepath"
|
|
"testing"
|
|
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
"github.com/cosmos/cosmos-sdk/x/genutil"
|
|
v032tendermint "github.com/kava-labs/kava/migrate/v0_8/tendermint/v0_32"
|
|
v033tendermint "github.com/kava-labs/kava/migrate/v0_8/tendermint/v0_33"
|
|
"github.com/stretchr/testify/require"
|
|
tmtypes "github.com/tendermint/tendermint/types"
|
|
|
|
"github.com/kava-labs/kava/app"
|
|
)
|
|
|
|
func TestMain(m *testing.M) {
|
|
config := sdk.GetConfig()
|
|
app.SetBech32AddressPrefixes(config)
|
|
app.SetBip44CoinType(config)
|
|
|
|
os.Exit(m.Run())
|
|
}
|
|
|
|
func TestMigrate_Auth_BaseAccount(t *testing.T) {
|
|
bz, err := ioutil.ReadFile(filepath.Join("testdata", "auth-base-old.json"))
|
|
require.NoError(t, err)
|
|
oldAppState := genutil.AppMap{"auth": bz}
|
|
|
|
newAppState := MigrateAppState(oldAppState)
|
|
|
|
bz, err = ioutil.ReadFile(filepath.Join("testdata", "auth-base-new.json"))
|
|
require.NoError(t, err)
|
|
require.JSONEq(t, string(bz), string(newAppState["auth"]))
|
|
}
|
|
func TestMigrate_Auth_MultiSigAccount(t *testing.T) {
|
|
bz, err := ioutil.ReadFile(filepath.Join("testdata", "auth-base-multisig-old.json"))
|
|
require.NoError(t, err)
|
|
oldAppState := genutil.AppMap{"auth": bz}
|
|
|
|
newAppState := MigrateAppState(oldAppState)
|
|
|
|
bz, err = ioutil.ReadFile(filepath.Join("testdata", "auth-base-multisig-new.json"))
|
|
require.NoError(t, err)
|
|
require.JSONEq(t, string(bz), string(newAppState["auth"]))
|
|
}
|
|
|
|
func TestMigrate_Auth_ValidatorVestingAccount(t *testing.T) {
|
|
bz, err := ioutil.ReadFile(filepath.Join("testdata", "auth-valvesting-old.json"))
|
|
require.NoError(t, err)
|
|
oldAppState := genutil.AppMap{"auth": bz}
|
|
|
|
newAppState := MigrateAppState(oldAppState)
|
|
|
|
bz, err = ioutil.ReadFile(filepath.Join("testdata", "auth-valvesting-new.json"))
|
|
require.NoError(t, err)
|
|
require.JSONEq(t, string(bz), string(newAppState["auth"]))
|
|
}
|
|
|
|
func TestMigrate_Auth_ModuleAccount(t *testing.T) {
|
|
bz, err := ioutil.ReadFile(filepath.Join("testdata", "auth-module-old.json"))
|
|
require.NoError(t, err)
|
|
oldAppState := genutil.AppMap{"auth": bz}
|
|
|
|
newAppState := MigrateAppState(oldAppState)
|
|
|
|
bz, err = ioutil.ReadFile(filepath.Join("testdata", "auth-module-new.json"))
|
|
require.NoError(t, err)
|
|
require.JSONEq(t, string(bz), string(newAppState["auth"]))
|
|
}
|
|
|
|
func TestMigrate_Auth_PeriodicVestingAccount(t *testing.T) {
|
|
bz, err := ioutil.ReadFile(filepath.Join("testdata", "auth-periodic-old.json"))
|
|
require.NoError(t, err)
|
|
oldAppState := genutil.AppMap{"auth": bz}
|
|
|
|
newAppState := MigrateAppState(oldAppState)
|
|
|
|
bz, err = ioutil.ReadFile(filepath.Join("testdata", "auth-periodic-new.json"))
|
|
require.NoError(t, err)
|
|
require.JSONEq(t, string(bz), string(newAppState["auth"]))
|
|
}
|
|
|
|
func TestMigrate_Distribution(t *testing.T) {
|
|
bz, err := ioutil.ReadFile(filepath.Join("testdata", "distribution-old.json"))
|
|
require.NoError(t, err)
|
|
oldAppState := genutil.AppMap{"distribution": bz}
|
|
|
|
newAppState := MigrateSDK(oldAppState)
|
|
|
|
bz, err = ioutil.ReadFile(filepath.Join("testdata", "distribution-new.json"))
|
|
require.NoError(t, err)
|
|
require.JSONEq(t, string(bz), string(newAppState["distribution"]))
|
|
}
|
|
|
|
func TestMigrate_Staking(t *testing.T) {
|
|
bz, err := ioutil.ReadFile(filepath.Join("testdata", "staking-old.json"))
|
|
require.NoError(t, err)
|
|
oldAppState := genutil.AppMap{"staking": bz}
|
|
|
|
newAppState := MigrateSDK(oldAppState)
|
|
|
|
bz, err = ioutil.ReadFile(filepath.Join("testdata", "staking-new.json"))
|
|
require.NoError(t, err)
|
|
require.JSONEq(t, string(bz), string(newAppState["staking"]))
|
|
}
|
|
|
|
func TestMigrate_Slashing(t *testing.T) {
|
|
bz, err := ioutil.ReadFile(filepath.Join("testdata", "slashing-old.json"))
|
|
require.NoError(t, err)
|
|
oldAppState := genutil.AppMap{"slashing": bz}
|
|
|
|
newAppState := MigrateSDK(oldAppState)
|
|
|
|
bz, err = ioutil.ReadFile(filepath.Join("testdata", "slashing-new.json"))
|
|
require.NoError(t, err)
|
|
require.JSONEq(t, string(bz), string(newAppState["slashing"]))
|
|
}
|
|
|
|
func TestMigrate_Evidence(t *testing.T) {
|
|
bz, err := ioutil.ReadFile(filepath.Join("testdata", "slashing-old.json"))
|
|
require.NoError(t, err)
|
|
oldAppState := genutil.AppMap{"slashing": bz}
|
|
|
|
newAppState := MigrateSDK(oldAppState)
|
|
|
|
bz, err = ioutil.ReadFile(filepath.Join("testdata", "evidence-new.json"))
|
|
require.NoError(t, err)
|
|
require.JSONEq(t, string(bz), string(newAppState["evidence"]))
|
|
}
|
|
|
|
func TestMigrate_Tendermint(t *testing.T) {
|
|
oldGenDoc, err := v032tendermint.GenesisDocFromFile(filepath.Join("testdata", "tendermint-old.json"))
|
|
require.NoError(t, err)
|
|
|
|
newGenDoc := v033tendermint.Migrate(*oldGenDoc)
|
|
|
|
expectedGenDoc, err := tmtypes.GenesisDocFromFile(filepath.Join("testdata", "tendermint-new.json"))
|
|
require.NoError(t, err)
|
|
require.Equal(t, *expectedGenDoc, newGenDoc)
|
|
}
|
|
|
|
func TestMigrate(t *testing.T) {
|
|
oldGenDoc, err := v032tendermint.GenesisDocFromFile(filepath.Join("testdata", "all-old.json"))
|
|
require.NoError(t, err)
|
|
|
|
newGenDoc := Migrate(*oldGenDoc)
|
|
|
|
expectedGenDoc, err := tmtypes.GenesisDocFromFile(filepath.Join("testdata", "all-new.json"))
|
|
require.NoError(t, err)
|
|
// check each field seperately to aid debugging
|
|
require.Equal(t, expectedGenDoc.AppHash, newGenDoc.AppHash)
|
|
require.JSONEq(t, string(expectedGenDoc.AppState), string(newGenDoc.AppState))
|
|
require.Equal(t, expectedGenDoc.ChainID, newGenDoc.ChainID)
|
|
require.Equal(t, expectedGenDoc.ConsensusParams, newGenDoc.ConsensusParams)
|
|
require.Equal(t, expectedGenDoc.GenesisTime, newGenDoc.GenesisTime)
|
|
require.Equal(t, expectedGenDoc.Validators, newGenDoc.Validators)
|
|
|
|
var newAppState genutil.AppMap
|
|
require.NoError(t,
|
|
app.MakeCodec().UnmarshalJSON(newGenDoc.AppState, &newAppState),
|
|
)
|
|
require.NoError(t,
|
|
app.ModuleBasics.ValidateGenesis(newAppState),
|
|
)
|
|
}
|
|
|
|
func TestMigrate_Full(t *testing.T) {
|
|
// 1) load an exported kava-2 state
|
|
oldGenDoc, err := v032tendermint.GenesisDocFromFile(filepath.Join("testdata", "kava-2.json"))
|
|
require.NoError(t, err)
|
|
tApp := app.NewTestApp() // also sets the bech32 prefix on sdk.Config
|
|
cdc := app.MakeCodec()
|
|
|
|
// 2) migrate
|
|
newGenDoc := Migrate(*oldGenDoc)
|
|
|
|
// 3) check new genesis is valid
|
|
var newAppState genutil.AppMap
|
|
require.NoError(t,
|
|
cdc.UnmarshalJSON(newGenDoc.AppState, &newAppState),
|
|
)
|
|
require.NoError(t,
|
|
app.ModuleBasics.ValidateGenesis(newAppState),
|
|
)
|
|
require.NotPanics(t, func() {
|
|
// this runs both InitGenesis for all modules (which panic on errors) and runs all invariants
|
|
tApp.InitializeFromGenesisStates(app.GenesisState(newAppState))
|
|
})
|
|
}
|