mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-11-10 10:05:18 +00:00
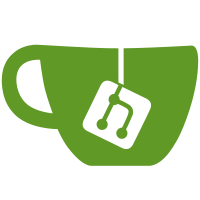
* update params * stub out methods * delete legacy migration test * feat: add infrastructure inflation and payouts * fix: load same module account blocked addrs in kavadist as app * fix: testing edge case where period starts and ends same block * fix: add store key for infra params, update newparams function * fix: testing edge case where period starts and ends in same block * add test for basic infra minting * add test for stand-alone core, partner rewards * fix: set time elapsed in each case * add e2e test for infra rewards * fix: use standard method for naming infra params key
36 lines
985 B
Go
36 lines
985 B
Go
package simulation
|
|
|
|
import (
|
|
"fmt"
|
|
"math/rand"
|
|
|
|
simtypes "github.com/cosmos/cosmos-sdk/types/simulation"
|
|
"github.com/cosmos/cosmos-sdk/x/simulation"
|
|
|
|
"github.com/kava-labs/kava/x/kavadist/types"
|
|
)
|
|
|
|
// ParamChanges defines the parameters that can be modified by param change proposals
|
|
// on the simulation
|
|
func ParamChanges(r *rand.Rand) []simtypes.ParamChange {
|
|
// Hacky way to validate periods since validation is wrapped in params
|
|
active := genRandomActive(r)
|
|
periods := genRandomPeriods(r, simtypes.RandTimestamp(r))
|
|
if err := types.NewParams(active, periods, types.DefaultInfraParams).Validate(); err != nil {
|
|
panic(err)
|
|
}
|
|
|
|
return []simtypes.ParamChange{
|
|
simulation.NewSimParamChange(types.ModuleName, string(types.KeyActive),
|
|
func(r *rand.Rand) string {
|
|
return fmt.Sprintf("%t", active)
|
|
},
|
|
),
|
|
simulation.NewSimParamChange(types.ModuleName, string(types.KeyPeriods),
|
|
func(r *rand.Rand) string {
|
|
return fmt.Sprintf("%v", periods)
|
|
},
|
|
),
|
|
}
|
|
}
|