mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 14:47:31 +00:00
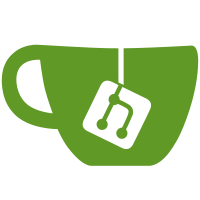
* add new field upgrade_time_set_staking_rewards_per_second with intention of integrating into the disable inflation logic to set an initial staking reward time * when the disable inflation upgrade time occurs, set the staking rewards per second to the value specified by the new upgrade_time_set_staking_rewards_per_second. This will allow a decoupled implementation between the ugprade switching logic, and the core functionality of paying staking rewards from the pool * add staking rewards state to community keeper and community module genesis that is required to calculate and track staking reward payouts accross blocks * add implementation of staking reward payouts * remove unused error * touch up tests and add a test case that fully tests behavior when pool is drained * add function comments * refactor and pull out main calculation to private pure function with no dependence on keeper * zero out default parameters -- these are too chain specific to have useful defaults * small touch ups on comments, test cases * use correct Int from sdkmath, not old sdk types; update protonet genesis for new parmater * fix copy pasta comment * use bond denom from staking keeper instead of referncing ukava directly * add staking reward state for valid genesis * update kvtool genesis for new params and rewards state
102 lines
2.7 KiB
Go
102 lines
2.7 KiB
Go
package community_test
|
|
|
|
import (
|
|
"testing"
|
|
"time"
|
|
|
|
"github.com/stretchr/testify/suite"
|
|
|
|
sdkmath "cosmossdk.io/math"
|
|
authtypes "github.com/cosmos/cosmos-sdk/x/auth/types"
|
|
|
|
"github.com/kava-labs/kava/x/community"
|
|
"github.com/kava-labs/kava/x/community/testutil"
|
|
"github.com/kava-labs/kava/x/community/types"
|
|
)
|
|
|
|
type genesisTestSuite struct {
|
|
testutil.Suite
|
|
}
|
|
|
|
func (suite *genesisTestSuite) SetupTest() {
|
|
suite.Suite.SetupTest()
|
|
}
|
|
|
|
func TestGenesisTestSuite(t *testing.T) {
|
|
suite.Run(t, new(genesisTestSuite))
|
|
}
|
|
|
|
func (suite *genesisTestSuite) TestInitGenesis() {
|
|
|
|
accountKeeper := suite.App.GetAccountKeeper()
|
|
|
|
genesisState := types.NewGenesisState(
|
|
types.NewParams(
|
|
time.Date(1998, 1, 1, 0, 0, 0, 0, time.UTC),
|
|
sdkmath.LegacyNewDec(1000),
|
|
sdkmath.LegacyNewDec(1000),
|
|
),
|
|
types.NewStakingRewardsState(
|
|
time.Date(1997, 1, 1, 0, 0, 0, 0, time.UTC),
|
|
sdkmath.LegacyMustNewDecFromStr("0.100000000000000000"),
|
|
),
|
|
)
|
|
|
|
suite.NotPanics(func() {
|
|
community.InitGenesis(suite.Ctx, suite.Keeper, accountKeeper, genesisState)
|
|
})
|
|
|
|
// check for module account this way b/c GetModuleAccount creates if not existing.
|
|
acc := accountKeeper.GetAccount(suite.Ctx, suite.MaccAddress)
|
|
suite.NotNil(acc)
|
|
_, ok := acc.(authtypes.ModuleAccountI)
|
|
suite.True(ok)
|
|
|
|
keeper := suite.App.GetCommunityKeeper()
|
|
storedParams, found := keeper.GetParams(suite.Ctx)
|
|
suite.True(found)
|
|
suite.Equal(genesisState.Params, storedParams)
|
|
|
|
stakingRewardsState := keeper.GetStakingRewardsState(suite.Ctx)
|
|
suite.Equal(genesisState.StakingRewardsState, stakingRewardsState)
|
|
}
|
|
|
|
func (suite *genesisTestSuite) TestExportGenesis() {
|
|
params := types.NewParams(
|
|
time.Date(1998, 1, 1, 0, 0, 0, 0, time.UTC),
|
|
sdkmath.LegacyNewDec(1000),
|
|
sdkmath.LegacyNewDec(1000),
|
|
)
|
|
suite.Keeper.SetParams(suite.Ctx, params)
|
|
|
|
stakingRewardsState := types.NewStakingRewardsState(
|
|
time.Date(1997, 1, 1, 0, 0, 0, 0, time.UTC),
|
|
sdkmath.LegacyMustNewDecFromStr("0.100000000000000000"),
|
|
)
|
|
suite.Keeper.SetStakingRewardsState(suite.Ctx, stakingRewardsState)
|
|
|
|
genesisState := community.ExportGenesis(suite.Ctx, suite.Keeper)
|
|
|
|
suite.Equal(params, genesisState.Params)
|
|
suite.Equal(stakingRewardsState, genesisState.StakingRewardsState)
|
|
}
|
|
|
|
func (suite *genesisTestSuite) TestInitExportIsLossless() {
|
|
genesisState := types.NewGenesisState(
|
|
types.NewParams(
|
|
time.Date(1998, 1, 1, 0, 0, 0, 0, time.UTC),
|
|
sdkmath.LegacyNewDec(1000),
|
|
sdkmath.LegacyNewDec(1000),
|
|
),
|
|
types.NewStakingRewardsState(
|
|
time.Date(1997, 1, 1, 0, 0, 0, 0, time.UTC),
|
|
sdkmath.LegacyMustNewDecFromStr("0.100000000000000000"),
|
|
),
|
|
)
|
|
|
|
community.InitGenesis(suite.Ctx, suite.Keeper, suite.App.GetAccountKeeper(), genesisState)
|
|
exportedState := community.ExportGenesis(suite.Ctx, suite.Keeper)
|
|
|
|
suite.Equal(genesisState, exportedState)
|
|
}
|