mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 14:47:31 +00:00
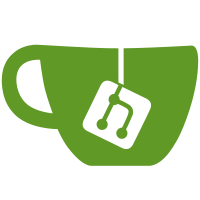
* Committee types (#899) * committee types * refactor to committee interface * include tokencommitee stringer method * add members to BaseCommittee * address revisions * update querier * update querier * fix compilation errors, tests, etc. * Update MsgVote with vote type (#900) * add vote to msg * update querier/rest * update example cli vote msg * remove incorrect comments * address revisions * update handler, stub keeper method * add vote type to vote struct * Committee module keeper logic for token holder governance (#902) * fix keeper/test compilation errors * fix keeper/test compilation errors pt 2 * add setters to committee interface * fix sims compilation errors * fix incentive tests compilation errors * update types, expected keepers * core keeper logic * don't allow bond denom * implement vote tallying * query proposal polling status * update module keepers in app.go * register committee interface * fix failing incentive test * commitee types tests * refactor GetProposalResult by committee types * update invariants * implement most proposal keeper tests * add nulls to custom enums * remove abstain vote type * add test for close proposal * remove outdated TODOs * update ProcessProposals * switch on committee type directly * reintroduce Abstain votes and update vote tallying * don't allow divide by 0 panics * delete unused setters on committee interface * clean up tally methods return values for querier * update enum validation to catch negative ints * reintroduce setters for sims compilation * address revisions * remove commented out test * implement ProcessProposals test * additional revisions * Committee migrations (#909) * add committee v14 legacy types * update migration imports for compile * addRegisterCodec() to committee v14 legacy types * migrate committee genesis state from v14 to v15 * set stability committee permissions properly * fix committee allowed params * migration test, kava-7 sample data * add concrete types to committees (#911) * revisions: migrate + tests * register msgs on legacy codec * Prepare Committee module for migrations (#906) * remove invariants * edits * fix abci test * fix keeper querier tests * add committee interface registration * use codec.Codec * don't allow null vote types * don't allow null tally option * minor spelling fixes * update example cli proposal * fix cli tally query * enable vote abstain from cli * include vote options in cli help text * call CloseProposal from handler * custom enum marshaling * committee: fix failing tests (#921) * fix failing tests * fix: spelling Co-authored-by: rhuairahrighairigh <ruaridh.odonnell@gmail.com> Co-authored-by: Ruaridh <rhuairahrighairidh@users.noreply.github.com> Co-authored-by: Kevin Davis <karzak@users.noreply.github.com>
79 lines
2.0 KiB
Go
79 lines
2.0 KiB
Go
package simulation
|
|
|
|
import (
|
|
"fmt"
|
|
"testing"
|
|
"time"
|
|
|
|
"github.com/stretchr/testify/require"
|
|
"github.com/tendermint/tendermint/libs/kv"
|
|
|
|
"github.com/cosmos/cosmos-sdk/codec"
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
govtypes "github.com/cosmos/cosmos-sdk/x/gov/types"
|
|
|
|
"github.com/kava-labs/kava/x/committee/types"
|
|
)
|
|
|
|
func makeTestCodec() (cdc *codec.Codec) {
|
|
cdc = codec.New()
|
|
sdk.RegisterCodec(cdc)
|
|
govtypes.RegisterCodec(cdc)
|
|
types.RegisterCodec(cdc)
|
|
return cdc
|
|
}
|
|
|
|
func TestDecodeStore(t *testing.T) {
|
|
cdc := makeTestCodec()
|
|
|
|
committee := types.NewMemberCommittee(
|
|
12,
|
|
"This committee is for testing.",
|
|
nil,
|
|
[]types.Permission{types.TextPermission{}},
|
|
sdk.MustNewDecFromStr("0.667"),
|
|
time.Hour*24*7,
|
|
types.FirstPastThePost,
|
|
)
|
|
proposal := types.Proposal{
|
|
ID: 34,
|
|
CommitteeID: 12,
|
|
Deadline: time.Date(1998, time.January, 1, 1, 0, 0, 0, time.UTC),
|
|
PubProposal: govtypes.NewTextProposal("A Title", "A description of this proposal."),
|
|
}
|
|
vote := types.Vote{
|
|
ProposalID: 9,
|
|
Voter: nil,
|
|
}
|
|
|
|
kvPairs := kv.Pairs{
|
|
kv.Pair{Key: types.CommitteeKeyPrefix, Value: cdc.MustMarshalBinaryLengthPrefixed(&committee)},
|
|
kv.Pair{Key: types.ProposalKeyPrefix, Value: cdc.MustMarshalBinaryLengthPrefixed(&proposal)},
|
|
kv.Pair{Key: types.VoteKeyPrefix, Value: cdc.MustMarshalBinaryLengthPrefixed(&vote)},
|
|
kv.Pair{Key: types.NextProposalIDKey, Value: sdk.Uint64ToBigEndian(10)},
|
|
kv.Pair{Key: []byte{0x99}, Value: []byte{0x99}},
|
|
}
|
|
|
|
tests := []struct {
|
|
name string
|
|
expectedLog string
|
|
}{
|
|
{"Committee", fmt.Sprintf("%v\n%v", committee, committee)},
|
|
{"Proposal", fmt.Sprintf("%v\n%v", proposal, proposal)},
|
|
{"Vote", fmt.Sprintf("%v\n%v", vote, vote)},
|
|
{"NextProposalID", "10\n10"},
|
|
{"other", ""},
|
|
}
|
|
for i, tt := range tests {
|
|
i, tt := i, tt
|
|
t.Run(tt.name, func(t *testing.T) {
|
|
switch i {
|
|
case len(tests) - 1:
|
|
require.Panics(t, func() { DecodeStore(cdc, kvPairs[i], kvPairs[i]) }, tt.name)
|
|
default:
|
|
require.Equal(t, tt.expectedLog, DecodeStore(cdc, kvPairs[i], kvPairs[i]), tt.name)
|
|
}
|
|
})
|
|
}
|
|
}
|